In the given example only one object will be created. Firstly JVM will not find any string object with the value “Welcome” in the string constant pool, so it will create a new object. After that it will find the string with the value “Welcome” in the pool, it will not create a new object but will return the reference to the same instance. In this article, we will learn about Java Strings.
What are Strings in Java?
Strings are the type of objects that can store the character of values and in Java, every character is stored in 16 bits i,e using UTF 16-bit encoding. A string acts the same as an array of characters in Java.
Example:
String name = "Geeks";
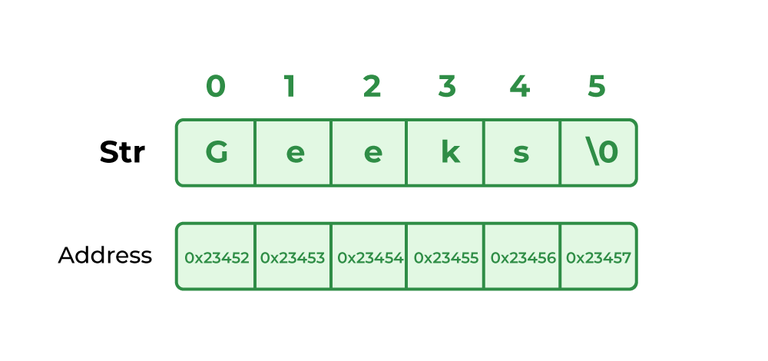
String Example in Java
Below is an example of a String in Java:
Java
// Java Program to demonstrate
// String
public class StringExample {
// Main Function
public static void main(String args[])
{
String str = new String("example");
// creating Java string by new keyword
// this statement create two object i.e
// first the object is created in heap
// memory area and second the object is
// created in String constant pool.
System.out.println(str);
}
}
Ways of Creating a String
There are two ways to create a string in Java:
- String Literal
- Using new Keyword
Syntax:
<String_Type> <string_variable> = "<sequence_of_string>";
1. String literal
To make Java more memory efficient (because no new objects are created if it exists already in the string constant pool).
Example:
String demoString = “GeeksforGeeks”;
2. Using new keyword
- String s = new String(“Welcome”);
- In such a case, JVM will create a new string object in normal (non-pool) heap memory and the literal “Welcome” will be placed in the string constant pool. The variable s will refer to the object in the heap (non-pool)
Example:
String demoString = new String (“GeeksforGeeks”);
Interfaces and Classes in Strings in Java
CharBuffer: This class implements the CharSequence interface. This class is used to allow character buffers to be used in place of CharSequences. An example of such usage is the regular-expression package java.util.regex.
String: It is a sequence of characters. In Java, objects of String are immutable which means a constant and cannot be changed once created.
CharSequence Interface
CharSequence Interface is used for representing the sequence of Characters in Java.
Classes that are implemented using the CharSequence interface are mentioned below and these provides much of functionality like substring, lastoccurence, first occurence, concatenate , toupper, tolower etc.
- String
- StringBuffer
- StringBuilder
1. String
String is an immutable class which means a constant and cannot be changed once created and if wish to change , we need to create an new object and even the functionality it provides like toupper, tolower, etc all these return a new object , its not modify the original object. It is automatically thread safe.
Syntax
String str= "geeks";
or
String str= new String("geeks")
2. StringBuffer
StringBuffer is a peer class of String, it is mutable in nature and it is thread safe class , we can use it when we have multi threaded environment and shared object of string buffer i.e, used by mutiple thread. As it is thread safe so there is extra overhead, so it is mainly used for multithreaded program.
Syntax:
StringBuffer demoString = new StringBuffer("GeeksforGeeks");
3. StringBuilder
StringBuilder in Java represents an alternative to String and StringBuffer Class, as it creates a mutable sequence of characters and it is not thread safe. It is used only within the thread , so there is no extra overhead , so it is mainly used for single threaded program.
Syntax:
StringBuilder demoString = new StringBuilder();
demoString.append("GFG");
StringTokenizer
StringTokenizer class in Java is used to break a string into tokens.
Example:
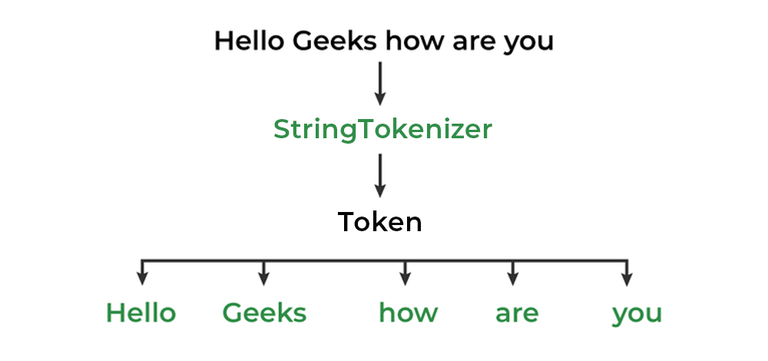
A StringTokenizer object internally maintains a current position within the string to be tokenized. Some operations advance this current position past the characters processed. A token is returned by taking a substring of the string that was used to create the StringTokenizer object.
StringJoiner is a class in java.util package which is used to construct a sequence of characters(strings) separated by a delimiter and optionally starting with a supplied prefix and ending with a supplied suffix. Though this can also be done with the help of the StringBuilder class to append a delimiter after each string, StringJoiner provides an easy way to do that without much code to write.
Syntax:
public StringJoiner(CharSequence delimiter)
Above we saw we can create a string by String Literal.
String demoString =”Welcome”;
Here the JVM checks the String Constant Pool. If the string does not exist, then a new string instance is created and placed in a pool. If the string exists, then it will not create a new object. Rather, it will return the reference to the same instance. The cache that stores these string instances is known as the String Constant pool or String Pool. In earlier versions of Java up to JDK 6 String pool was located inside PermGen(Permanent Generation) space. But in JDK 7 it is moved to the main heap area.
Immutable String in Java
In Java, string objects are immutable. Immutable simply means unmodifiable or unchangeable. Once a string object is created its data or state can’t be changed but a new string object is created.
Below is the implementation of the topic:
Java
// Java Program to demonstrate Immutable String in Java
import java.io.*;
class GFG {
public static void main(String[] args)
{
String s = "Sachin";
// concat() method appends
// the string at the end
s.concat(" Tendulkar");
// This will print Sachin
// because strings are
// immutable objects
System.out.println(s);
}
}
Here Sachin is not changed but a new object is created with “Sachin Tendulkar”. That is why a string is known as immutable.
As you can see in the given figure that two objects are created but s reference variable still refers to “Sachin” and not to “Sachin Tendulkar”. But if we explicitly assign it to the reference variable, it will refer to the “Sachin Tendulkar” object.
For Example:
Java
// Java Program to demonstrate Explicitly assigned strings
import java.io.*;
class GFG {
public static void main(String[] args)
{
String name = "Sachin";
name = name.concat(" Tendulkar");
System.out.println(name);
}
}
Memory Allotment of String
Whenever a String Object is created as a literal, the object will be created in the String constant pool. This allows JVM to optimize the initialization of String literal.
Example:
String demoString = "Geeks";
The string can also be declared using a new operator i.e. dynamically allocated. In case of String are dynamically allocated they are assigned a new memory location in the heap. This string will not be added to the String constant pool.
Example:
String demoString = new String("Geeks");
If you want to store this string in the constant pool then you will need to “intern” it.
Example:
String internedString = demoString.intern();
// this will add the string to string constant pool.
It is preferred to use String literals as it allows JVM to optimize memory allocation.
An example that shows how to declare a String
Java
// Java code to illustrate String
import java.io.*;
import java.lang.*;
class Test {
public static void main(String[] args)
{
// Declare String without using new operator
String name = "GeeksforGeeks";
// Prints the String.
System.out.println("String name = " + name);
// Declare String using new operator
String newString = new String("GeeksforGeeks");
// Prints the String.
System.out.println("String newString = " + newString);
}
}
OutputString name = GeeksforGeeks
String newString = GeeksforGeeks
Note: String Object is created in Heap area and Literals are stored in special memory area known as string constant pool.
Why did the String pool move from PermGen to the normal heap area?
PermGen space is limited, the default size is just 64 MB. it was a problem with creating and storing too many string objects in PermGen space. That’s why the String pool was moved to a larger heap area. To make Java more memory efficient, the concept of string literal is used. By the use of the ‘new’ keyword, The JVM will create a new string object in the normal heap area even if the same string object is present in the string pool.
For example:
String demoString = new String("Bhubaneswar");
Let us have a look at the concept with a Java program and visualize the actual JVM memory structure:
Below is the implementation of the above approach:
Java
// Java program and visualize the
// actual JVM memory structure
// mentioned in image
class StringStorage {
public static void main(String args[])
{
// Declaring Strings
String s1 = "TAT";
String s2 = "TAT";
String s3 = new String("TAT");
String s4 = new String("TAT");
// Printing all the Strings
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
System.out.println(s4);
}
}
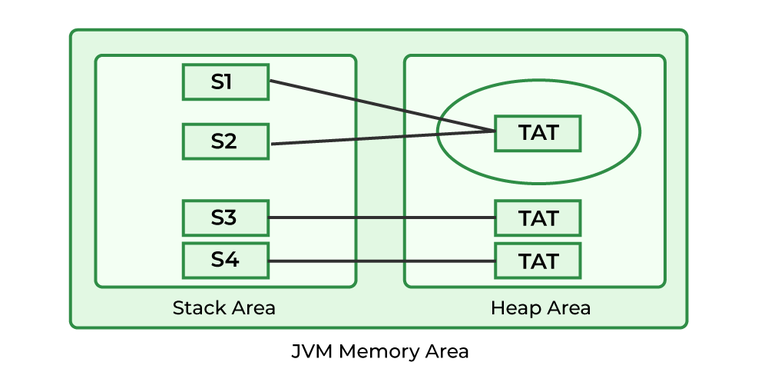
String Pool in Java
Note: All objects in Java are stored in a heap. The reference variable is to the object stored in the stack area or they can be contained in other objects which puts them in the heap area also.
Example 1:
Java
// Construct String from subset of char array
// Driver Class
class GFG {
// main function
public static void main(String args[])
{
byte ascii[] = { 71, 70, 71 };
String firstString = new String(ascii);
System.out.println(firstString);
String secondString = new String(ascii, 1, 2);
System.out.println(secondString);
}
}
Example 2:
Java
// Construct one string from another
class GFG {
public static void main(String args[])
{
char characters[] = { 'G', 'f', 'g' };
String firstString = new String(characters);
String secondString = new String(firstString);
System.out.println(firstString);
System.out.println(secondString);
}
}
Frequently Asked Questions
1. What are strings in Java?
Strings are the types of objects which can store characters as elements.
2. Why string objects are immutable in Java?
Because java uses the concept of string literal. Suppose there are 5 reference variables, all refer to one object “Sachin”. If one reference variable changes the value of the object, it will be affected by all the reference variables. That is why string objects are immutable in Java.
3. Why Java uses the concept of string literal?
To make Java more memory efficient (because no new objects are created if it exists already in the string constant pool).
Share your thoughts in the comments
Please Login to comment...