Chakra UI Data Display List
Last Updated :
04 Feb, 2024
The Chakra UI is a popular React component library that simplifies the process of building user interfaces with a set of customizable and accessible components. Among those features, it also provides support for displaying data lists. It provides various types of list components such as Ordered Lists, Unordered List, List with Icon. In this article, we will learn about the Implementation of different types of list in Chakra UI.
Prerequisites:
Approach:
We have created a Flex Box and inside that box we have used components such as List, OrderedList, UnorderedList to create a different types of List. List and UnorderedList will be rendered as a <ul> tag and OrderedList is rendered as <ol> tag. After that inside all those list, ListItem component is used to create list and it is rendered as <li> tag and ListIcon component is used to create a custom svg icon and it is rendered as <svg> tag. To use all this components we have to import it from “@chakra-ui/react” module.
We have used icons from Chakra UI Icons, so we have to install and import “@chakra-ui/icons” module to use different icons.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. gfg), move to it by using the following command:
cd gfg
Step 3: After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @chakra-ui/icons @emotion/react @emotion/styled framer-motion
Project Structure:
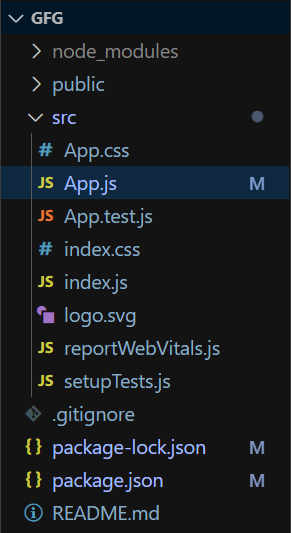
The updated dependencies in package.json file:
"dependencies": {
"@chakra-ui/icons": "^2.1.1",
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.3",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^11.0.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: The below example is demonstrating the use of Different type of List in Chakra UI.
Javascript
import {
SettingsIcon, EmailIcon,
PhoneIcon
} from '@chakra-ui/icons' ;
import {
List, ListItem, ListIcon,
OrderedList, UnorderedList,
Box, Heading
} from '@chakra-ui/react' ;
function App() {
return (
<>
<Box textAlign= "center" >
<Heading ams= "h1" color= "green" >
Chakra UI List | GeeksForGeeks
</Heading>
</Box>
<Box display= 'flex'
justifyContent= 'center'
alignItems= 'center' >
<h4>Ordered List</h4>
<OrderedList>
<ListItem>First</ListItem>
<ListItem>Second</ListItem>
<ListItem>Third</ListItem>
<ListItem>Fourth</ListItem>
</OrderedList>
</Box>
<Box display= 'flex'
justifyContent= 'center'
alignItems= 'center' >
<h4>Unordered List</h4>
<UnorderedList>
<ListItem>First</ListItem>
<ListItem>Second</ListItem>
<ListItem>Third</ListItem>
<ListItem>Fourth</ListItem>
</UnorderedList>
</Box>
<Box display= 'flex'
justifyContent= 'center'
alignItems= 'center' >
<h4>Icon List</h4>
<List>
<ListItem>
<ListIcon as={SettingsIcon}
marginX= "5px" />
Settings
</ListItem>
<ListItem>
<ListIcon as={EmailIcon}
marginX= "5px" />
Email
</ListItem>
<ListItem>
<ListIcon as={PhoneIcon}
marginX= "5px" />
Phone
</ListItem>
</List>
</Box>
</>
);
}
export default App;
|
To run the application use the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
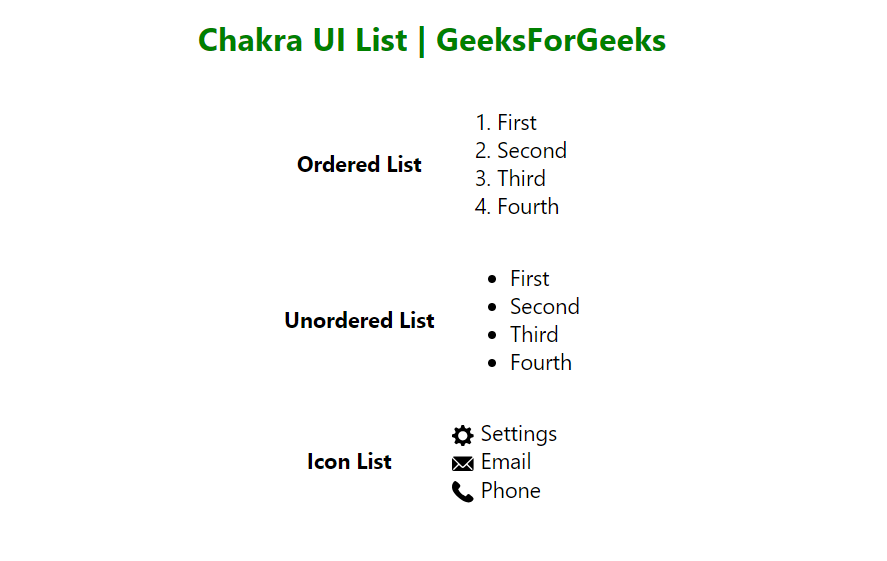
Share your thoughts in the comments
Please Login to comment...