Next.js Migrating from React Router
Last Updated :
26 Apr, 2023
Are you using React Router in your web application and planning to switch to Next.js? Don’t worry, the migration process is easy and we’ve got you covered! This blog post will guide you through the process and help you understand the benefits of doing so.
What is Next.js?
Next.js is a React-based framework used for building server-side rendered (SSR) applications. It provides a lot of features out of the box, such as automatic code splitting, server-side rendering, and static site generation. Next.js also comes with built-in support for TypeScript, CSS modules, and API routes.
Â
Why migrate from React Router to Next.js?
While React Router is a great library for client-side routing, it doesn’t provide server-side rendering out of the box. This means that when a user visits a page, the browser has to wait for the JavaScript to load before rendering the page. This can lead to slower page load times and poor user experience.
On the other hand, Next.js provides server-side rendering by default, which can improve page load times and provide a better user experience. Additionally, Next.js provides automatic code splitting, which means that only the necessary JavaScript code is downloaded when a user visits a page, further improving performance.
Create NextJS Application:
Install Next.js:
npx create-next-app@latest
After the installation is complete start the development server:
npm run dev
Open to http://localhost:3000 to view your application
Migrating to Next.js Routing: We will do it in 2 steps:
Step 1: Creating pages: The first step in migrating your app from React Router to NextJS is to convert your existing code. The good news is Next.js uses the file system to define routes. When you create a file in the pages directory, Next.js automatically creates a route based on the file name. For example, if you create a file named about.js in the pages directory, Next.js will automatically create a route for /about.
To migrate your React Router app to Next.js, you need to create a page for each route in your app. For example, if you have a route in your React Router app defined like this:
Syntax:
<Route path="/about" component={About} />
For example, you may have the following directory or project structure:
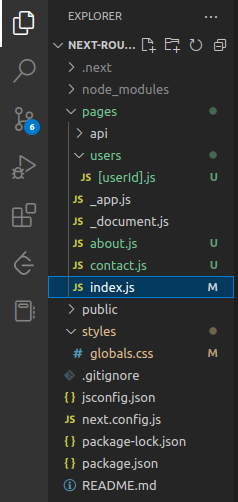
Â
Create a file named about.js & contact.js in the pages directory and define the About & Contact component in that file:
about.js
Javascript
const About = () => {
return (
<div>
<h1>About</h1>
<p>This is the about page.</p>
</div>
);
};
export default About;
|
contact.js
Javascript
function Contact() {
return (
<div>
<h1>Contact</h1>
<p>This is the contact page.</p>
</div>
);
};
export default Contact;
|
NextJS will automatically handle routing based on the file names in your pages directory.Â
File |
Routes |
about.js |
/about |
contact.js |
/contact |
index.js |
/ |
 In each file, you’ll need to export a React component that represents your page.Â
Step 2: Adding links: In a React Router app, you would use the Link component to create links to other pages in your app. In Next.js, you can use the Link component as well, but instead of using the to prop, you use the href prop. For example, if you have a link in your React Router app defined like this:
Syntax:
<Link to="/about">About</Link>
You would define the link in your Next.js app like this:
index.js
Javascript
import Link from "next/link" ;
const Home = () => {
return (
<div>
<h1>Welcome to my home page</h1>
<ul>
<li><Link href= "/about" >About</Link></li>
<li><Link href= "/contact" >Contact</Link></li>
</ul>
</div>
);
};
export default Home;
|
Output:
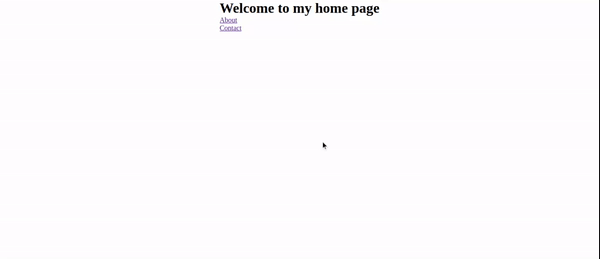
Â
Dynamic Routing: NextJS provides a range of routing features that make it easier to handle complex routing scenarios. In a React Router app, you can define dynamic routes using a colon followed by a parameter name.
Syntax:
<Route path="/users/:userId" component={User} />
In Next.js, you can define dynamic routes in a similar way, but using square brackets instead of a colon. For example in the pages directory create a new directory user, inside it creates a new file [userId].js
pages/users/[userId].js
Javascript
import { useRouter } from "next/router" ;
const User = () => {
const router = useRouter();
const { userId } = router.query;
return (
<div>
<h1>User {userId}</h1>
</div>
);
};
export default User;
|
Note that in the above code, we use the useRouter hook from Next.js to access the userId parameter from the query string.
The route /users/123 will have the following query object:
{ "userId": "123" }
To test the dynamic routes you may make the following changes in the index.js file
Javascript
import Link from "next/link" ;
const Home = () => {
return (
<div>
<h1>Welcome to my home page</h1>
<ul>
<li><Link href= "/about" >About</Link></li>
<li><Link href= "/contact" >Contact</Link></li>
</ul>
{ }
<br />
<ul>
<li><Link href= "/users/1" >User 1</Link></li>
<li><Link href= "/users/2" >User 2</Link></li>
<li><Link href= "/users/3" >User 3</Link></li>
</ul>
</div>
);
};
export default Home;
|
Output:
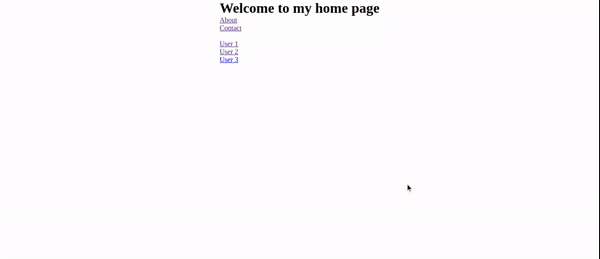
Â
In conclusion, migrating an app from React Router to Next.js can simplify the routing and navigation process and improve the performance of the application. To learn more check out this official Next.js documentation. I hope this article helped you migrate your app from React Router to Next.js.
Share your thoughts in the comments
Please Login to comment...