Implementing React Router with Redux in React
Last Updated :
22 Mar, 2024
This article explores implementing React Router with Redux for enhanced state management and routing in React applications.
What is React Router and Redux?
React Router facilitates routing in React apps, managing component rendering based on URL. Redux serves as a state container, aiding centralized state management. Integrating both enables developers to build SPAs with intricate routing and predictable state flow. Redux manages the application state, including route-related data, ensuring consistency across the app.
Approach to implement React Router in Redux:
- Component Creation:
- Create functional components for Home, About, and NotFound.
- Each component returns JSX representing its content.
- Redux Integration:
- Define Redux store with initial state and reducer.
- Connect Home component to Redux store to manage count state.
- Routing Setup:
- Use ‘react-router-dom’ for routing.
- Define routes for Home, About, and NotFound.
- Render NotFound for undefined routes.
- Application Rendering:
- Wrap the app with Provider for Redux store access.
- Render the app with ReactDOM.render, using StrictMode.
Steps to Setup the React App:
Step 1: Initialize Project
npx create-react-app react-router-redux-app
cd react-router-redux-app
Step 2: Install Dependencies
npm install react-router-dom react-redux redux
Project Structure:
Updated Dependencies in package.json file:
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2",
"react-redux": "^7.2.6",
"react-router-dom": "^6.2.1",
"redux": "^4.1.2"
}
Example:
- create components folder inside src with About.js, Home.js and NotFound.js and use the code given below
- create files store.js and also replace the code of App.js and index.js with the code given below
JavaScript
// Home.js
import React from 'react';
import { connect } from 'react-redux';
const Home = ({ count, increment, decrement }) => {
return (
<div>
<h1>Home</h1>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = state => ({
count: state.count
});
const mapDispatchToProps = dispatch => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
});
export default connect(mapStateToProps, mapDispatchToProps)(Home);
JavaScript
// About.js
import React from 'react';
const About = () => {
return (
<div>
<h1>About</h1>
<p>This is the About page!</p>
</div>
);
};
export default About;
JavaScript
// store.js
import { createStore } from 'redux';
const initialState = {
count: 0
};
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
...state,
count: state.count + 1
};
case 'DECREMENT':
return {
...state,
count: state.count - 1
};
default:
return state;
}
};
const store = createStore(reducer);
export default store;
JavaScript
// NotFound.js
import React from 'react';
const NotFound = () => {
return (
<div>
<h1>404 Not Found</h1>
<p>Oops! Page not found.</p>
</div>
);
};
export default NotFound;
JavaScript
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import { Provider } from 'react-redux';
import store from './store';
import Home from './components/Home';
import About from './components/About';
import NotFound from './components/NotFound';
const App = () => {
return (
<Provider store={store}>
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="*" element={<NotFound />} />
</Routes>
</Router>
</Provider>
);
};
export default App;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Output:
- Navigating to “/” will display the Home component.
- Navigating to “/about” will display the About component.
- Navigating to any other route will display the NotFound component.
- The application integrates Redux for state management and React Router for client-side routing.
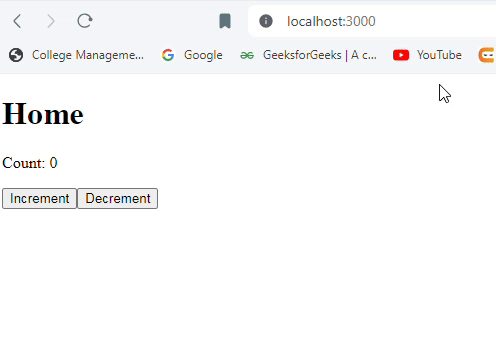
Share your thoughts in the comments
Please Login to comment...