Next.js Migrating from Gatsby
Last Updated :
06 Sep, 2023
If you use Gatsby and want to switch to the newest NextJs ecosystem, you’ve come to the right spot. Here, you can learn all about the differences between the two technologies and discover why it’s the best moment to switch from Gatsby to NextJs.
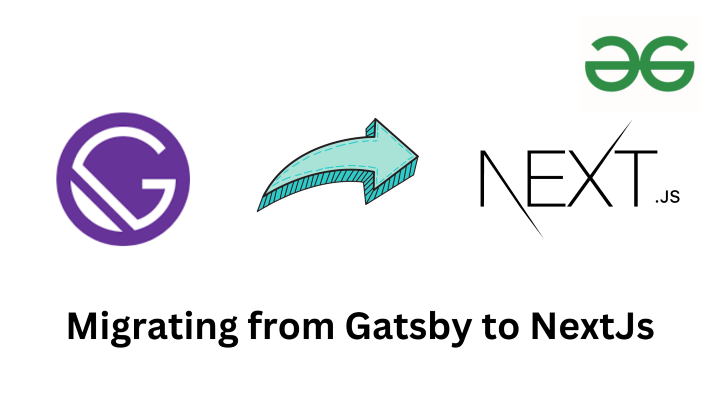
NextJS Migrating from Gatsby
Gatsby is an open-source framework used to build modern, high-performance websites and applications. It is based on React, a popular JavaScript library for building user interfaces which build by Facebook and published in 2013. With the help of Gatsby, static site generation can be used to build websites that load quickly and offer a positive user experience. Gatsby’s capacity to collect data from many sources, including content management systems (CMS), APIs, databases, or markdown files, and convert it into static HTML, CSS, and JavaScript throughout the build process is one of its most important capabilities. This facilitates quick initial page loads and makes it simple for search engines to index the material.
NextJs is a popular open-source framework for building modern web applications using React. It is built on top of React and provides additional features to enhance development and optimize performance. Next.js enables server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR) capabilities, giving developers flexibility in choosing the rendering strategy that best suits their needs.
Reasons for Migrating
- Developer Experience: There are many new frameworks and technologies available today that may be used to create static and dynamic websites, however not all of these frameworks and technologies provide a positive developer experience.
- Dynamic and real-time rendering: During the construction process, Gatsby primarily concentrates on server-side rendering (SSR) and static site generation (SSG). In addition to SSG and SSR, Next.js provides the flexibility of client-side rendering (CSR) if your application needs more dynamic and real-time rendering. Applications that significantly rely on real-time data updates or interactive user interfaces may find this handy.
- Routing: With the help of the built-in API routes functionality of Next.js, you may provide serverless API endpoints for use in your application. This can make it easier to manage server-side logic, fetch data, and communicate with external APIs. Next.js could provide a more user-friendly solution if your Gatsby application needs sophisticated server-side capabilities.
- Ecosystem and Open-Source Technology: Due to the size and activity of the Next.js community, there is frequently a thriving ecosystem of plugins, resources, and tools. It could be advantageous to switch to Next.js if you discover that it has certain plugins or integrations that meet the needs of your project in order to benefit from the ecosystem and community support. Additionally, because it is an open-source community, any developer may contribute, and if someone has issues, many people are willing to assist you.
- Performance: NextJs offers both Server-Side rendering as well as Client-Side rendering so the developers have the freedom to use this feature according to their need for the website. Although both Gatsby and Next.js have an emphasis on efficiency, Next.js by default enables automated code splitting, which can speed up initial page loads. Additionally, Next.js enables more precise control over data fetching techniques, which in some circumstances might be useful for performance optimization.
How to Migrate from Gatsby to NextJs?
Step 1: Change dependencies in the package.json:
{
"name": "my-gatsby-project",
"version": "1.0.0",
"description": "A Gatsby project",
"author": "Your Name",
"scripts": {
"develop": "gatsby develop",
"build": "gatsby build",
"serve": "gatsby serve"
},
"dependencies": {
"gatsby": "^3.0.0",
"react": "^17.0.0",
"react-dom": "^17.0.0"
},
"devDependencies": {
"gatsby-plugin-react-helmet": "^4.0.0",
"gatsby-plugin-sass": "^4.0.0",
"gatsby-source-filesystem": "^4.0.0",
"gatsby-transformer-remark": "^4.0.0"
},
"keywords": [
"gatsby"
],
"license": "MIT"
}
This is a Gatsby package.json file and we have to change it for NextJs, for that we need to use a command in our terminal:
npx create-next-app@latest
Now the package.json file looks like this:
{
"name": "next_app",
"version": "0.1.0",
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
},
"dependencies": {
"next": "13.4.5",
"react": "18.2.0",
"react-dom": "18.2.0"
},
"devDependencies": {
"autoprefixer": "^10.4.14",
"postcss": "^8.4.24",
"tailwindcss": "^3.3.2"
}
}
Changes and Benefits of Migration
Routing Changes:
We can easily set routing pages in NextJs, in two different ways:-
- We can create a pages folder in the root directory and create a file for each of our pages.
pages/blog/post.js
Or we can represent a dynamic route with a slug variable. Link this:
pages/blog/[slug].js
The value slug can be accessed as a parameter in the component using NextJs’s “userRouter“.
Data Fetching
There are many ways to acquire data in NextJs, but in this section, we’ll look at the most popular ones.
Static Generation (getStaticProps): Here NextJs run the “getStaticProps
” to fetch the data at the build time of the web app.
Javascript
export async function getStaticProps() {
const data = await res.json();
return {
props: {
data,
},
};
}
function MyPage({ data }) {
return (
<div>
{ }
</div>
);
}
export default MyPage;
|
Server-side Rendering (getServerSideProps): This is the most popular method in NextJs, and most developers use NextJs for this feature. Here “getServerSideProps” function runs on the server for every request and fetches the data, which is then passed as props to the page component. Example:
Javascript
export async function getServerSideProps() {
const data = await res.json();
return {
props: {
data,
},
};
}
function MyPage({ data }) {
return (
<div>
{ }
</div>
);
}
export default MyPage;
|
Client-side Rendering (useEffect/useSWR): Sometimes developers need their project to load data on the client side for that NextJs uses the “useEffect“.It is very similar to how we fetch data in ReactJs. Example:
Javascript
import { useState, useEffect } from 'react' ;
function MyPage() {
const [data, setData] = useState( null );
useEffect(() => {
async function fetchData() {
const res =
const data = await res.json();
setData(data);
}
fetchData();
}, []);
return (
<div>
{ }
</div>
);
}
export default MyPage;
|
Image components and their optimization:
NextJs optimizes the image as users request them with the <Image/> component.
Javascript
function MyComponent() {
return (
<div>
<Image
src= "/path/to/image.jpg"
alt= "Description of the image"
width={500}
height={300}
layout= "responsive"
priority={ true }
objectFit= "cover"
objectPosition= "center"
loading= "lazy"
blurDataURL= "/path/to/placeholder.png"
placeholder= "blur"
/>
</div>
);
}
|
SEO (Search Engine Optimization)
Today one of the major reasons to migrate from Gatsby to NextJs is the SEO(Search Engine Optimization), in NextJs we can create a separate SEO component `<SEO/>` like this with an SEO.js file, where we can add `<head><head/>` tag, `<meta/>` tag and add the important data of our website or web app.
Both Next.js and Gatsby are popular frameworks for building modern web apps, however, NextJs offers clear benefits over Gatsby. NextJs offers superior performance, SEO, and overall platform flexibility and capabilities. Due to its focus on the developer experience and continual improvements from the Vercel team, NextJs is an obvious choice for developers seeking to create high-quality, scalable apps. The benefits of migrating from Gatsby to NextJs are clear, and your forthcoming project would benefit greatly from NextJs’ variety and power.
Share your thoughts in the comments
Please Login to comment...