How To Implement Navigation In React JS ?
Last Updated :
22 Feb, 2024
React router is a powerful library that enables developers to manage navigation in a React application. It provides a way to define the routes and their corresponding components, allowing for a good user experience while navigating between different parts of any application. In this article, we will learn how to navigate routing in React apps.
Prerequisites
Approach to implement Navigation in React JS:
- Create a new react app as “npx create-react-app myapp“.
- Install react router dom as “npm i react-router-dom“.
- Create a Folder named “Components” inside “src” folder and create three files in it as “About.jsx“, “Contact.jsx” ans “Home.jsx“.
- Add provided code to these files.
Project Structure:
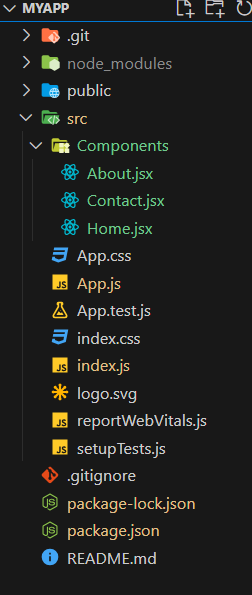
The updated package.json file will look like this
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.1",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Below is the code example of the Navigating Routing in MERN Apps:
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom/client' ;
import './index.css' ;
import App from './App' ;
import reportWebVitals from './reportWebVitals' ;
import { BrowserRouter } from 'react-router-dom' ;
const root = ReactDOM.createRoot(document.getElementById( 'root' ));
root.render(
<React.StrictMode>
<BrowserRouter>
<App />
</BrowserRouter>
</React.StrictMode>
);
reportWebVitals();
|
Javascript
import './App.css' ;
import {
Link,
Route,
Routes
} from 'react-router-dom' ;
import Home from './Components/Home' ;
import About from './Components/About' ;
import Contact from './Components/Contact' ;
function App() {
return (
<div className= "App" >
<div>
<div style={{
display: 'flex' ,
justifyContent: 'space-evenly' ,
background: 'green' ,
fontSize: '20px' ,
}}>
<Link to={ '/' }
style={{ color: 'white' }}>
Home
</Link>
<Link to={ '/about' }
style={
{
color: 'white'
}}>
About
</Link>
<Link to={ '/contact' }
style={
{
color: 'white'
}}>
Contact
</Link>
</div>
</div>
<Routes>
<Route path= "/"
element={<Home />} />
<Route path= "/about"
element={<About />} />
<Route path= "/contact"
element={<Contact />} />
</Routes>
</div>
);
}
export default App;
|
Javascript
import React from 'react'
import { Link } from 'react-router-dom'
const Home = () => {
return (
<div>
This is home page
</div>
)
}
export default Home
|
Javascript
import React from 'react'
import { Link } from 'react-router-dom'
const About = () => {
return (
<div>
This is about page
</div>
)
}
export default About
|
Javascript
import React from 'react'
import { Link }
from 'react-router-dom'
const Contact = () => {
return (
<div>
This is contact page
</div>
)
}
export default Contact
|
Step to run the App:
npm start
Output: Open browser and enter the url http://localhost:3000/
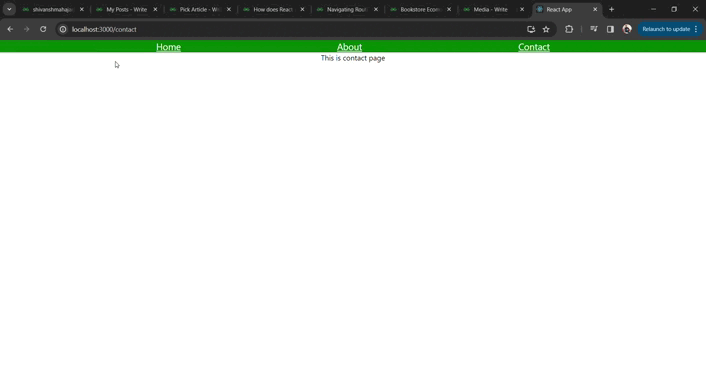
Share your thoughts in the comments
Please Login to comment...