How to Create Custom Router with the help of React Router ?
Last Updated :
18 Mar, 2024
To create a custom Router using React Router, you can define a new component that utilizes the Router’s ‘BrowserRouter’ along with ‘Route’ components to handle different paths and render corresponding components. Use ‘Link’ components for navigation within your application, providing a seamless routing experience in your React application.
What is a React Router?
React Router is a popular library for implementing routing in React applications. It allows developers to manage navigation and rendering of components in response to browser URL changes. React Router enables the creation of single-page applications (SPAs) by providing declarative routing capabilities within the React ecosystem.
Steps to Create React App And Installing React Router:
Step 1: Create a New React Project: Set up a new React project using
npx create-react-app my-react-app
Step 2: Navigate to Your Project Directory
cd my-react-app
Step 3: Install following modules
npm install react react-router-dom
Project Structure:
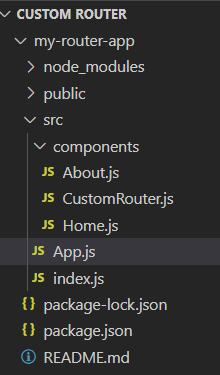
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^6.22.3",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach to create Custom Router using React Router:
- First define the routes in the main App component using React Router.
- Using Links for navigation between different routes.
- Creating a CustomRouter component that utilizes React Router’s useLocation hook to track the current path and render the corresponding component based on the route path.
- Using useEffect hook to update the current path state when the location changes.
Example: This example shows implementation to create a custom router.
Javascript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Javascript
// App.js
import React from 'react';
import { BrowserRouter as Router, Link } from 'react-router-dom';
import Home from './Components/Home';
import About from './Components/About';
import CustomRouter from './Components/CustomRouter';
const App = () => {
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About }
];
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
<hr />
<h1>Custom Router Example</h1>
<CustomRouter initialPath=
{window.location.pathname} routes={routes} />
</div>
</Router>
);
};
export default App;
Javascript
// Home.js
import React from 'react';
const Home = () => {
return (
<div>
<h2>
Welcome to Home Page
</h2>
</div>
);
}
export default Home;
Javascript
// About.js
import React from 'react';
const About = () => {
return (
<div>
<h2>About Us</h2>
<p>
This is the About page.
</p>
</div>
);
}
export default About;
Javascript
// CustomRouter.js
import React, { useState, useEffect } from 'react';
import { useLocation } from 'react-router-dom';
const CustomRouter = ({ routes }) => {
const location = useLocation();
const [currentPath, setCurrentPath] =
useState('');
useEffect(() => {
setCurrentPath(location.pathname);
}, [location]);
const RouteComponent =
routes.find(route => route.path === currentPath)?.component;
return RouteComponent ? <RouteComponent /> : null;
};
export default CustomRouter;
Start your application using the following command.
npm start
Output:
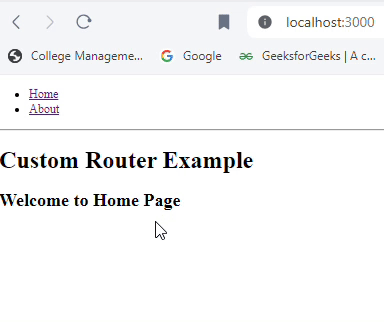
Share your thoughts in the comments
Please Login to comment...