What are the React Router hooks in React v5?
Last Updated :
03 Apr, 2024
React Router hooks perform client-side single-page routing that provides a functional and streamlined approach to managing navigation in React applications. It provides a way to directly access the state of the router from functional components, this simplifies tasks like retrieving information about the desired URL and navigating through web pages.
We will discuss the different React Router hooks in React v5:
Prerequisites:
useParams
useParams hook is a functional component hook that is used to extract dynamic route parameters from the current or active URL. The term Params in ‘useParams’ defines parameters that are used to retrieve data from a URL. useParams retrieves an object containing key-value pairs where the keys are parameter names defined in the route path and the values are corresponding parameter values from that URL.
It’s important to define the expected parameters in the route path using colons (:). If a parameter is not present in the URL its respective value in the object will be “undefined“.
Example: Below is an example of useParams. In the below code the useParams() retrieves the parameters or values of the URL and destructures them as “productId” and “color”. Parameters of URL are ‘123’ and ‘blue’.
JavaScript
import {
BrowserRouter,
Routes,
Route
} from 'react-router-dom';
import MyComponent from './MyComponent';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/products/:productId/:color"
element={<MyComponent />} />
</Routes>
</BrowserRouter>
);
}
function MyComponent() {
const { productId, color } = useParams();
return (
<div>
<p>Product ID: {productId}</p>
<p>Color: {color}</p>
</div>
);
}
export default App;
Output :
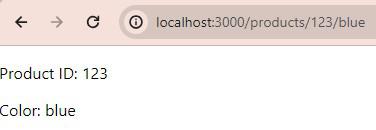
useLocation
useLocation retrieves the current URL information as an object, this object changes whenever the user navigates to a new URL. Object provides properties like pathname, search string and a hash fragment. useLocation hook can also be used in cases like triggering events by a change in URL.
useLocation only reflects the current URL and doesn’t triggger re-renders when the URL changes on its own. If you need to respond to your URL changes , consider using the “useEffect” hook along with the “useLocation” hook.
Example: Below is an example of useLocation. In the below code the “location.search() ” returns the entire string after ” ? ” from the URL.
JavaScript
import {
BrowserRouter,
Routes,
Route
} from 'react-router-dom';
import MyComponent from './MyComponent';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<MyComponent />} />
<Route path="/products/:productId"
element={<MyComponent />} />
</Routes>
</BrowserRouter>
);
}
function MyComponent() {
const location = useLocation();
return (
<div>
<p>Current Path: {location.pathname}</p>
<p>Search String: {location.search}</p>
</div>
);
}
export default App;
Output:
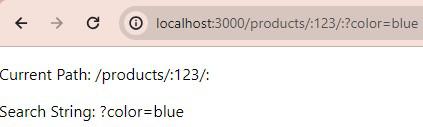
useHistory
useHistory hook provides access to history object that manages the history stack. History stack contains all the URL’s visited by the user. “useHistory” hook grants access to history instance which is created by React router. Using the history instance users can navigate from one URL to another , it also allows user to move back and forth using browser buttons or custom logic.
Methods of useHistory hook :
- history.push(path) : Navigates to a new URL by pushing it onto the history stack.
- history.replace(path) : Replaces the current URL in the history stack with the provided path. This can be useful in cases when we don’t want to revisit the same page again by navigating backwards.
- history.goBack() : Navigates to the previous URL in the history stack, similar to brower’s backward button.
- history.goForward() : Navigates to next URl in the history stack, similar to browser’s forward button
*Note : “useHistory()” hook will only work in the React-router-dom v5 but not in v6 , as it is replaced with “useNavigate()” hook.
Example: Below is an example of useHistory. Clicking the “Logout ” button displays a message (“User logged out “) on to the console and also redirects you to the ‘login page’.
JavaScript
import React from 'react';
import { useHistory } from 'react-router-dom';
function Dashboard() {
const history = useHistory();
const handleLogout = () => {
// Simulate some logout logic (e.g., clear user data)
console.log('User logged out');
// Redirect user to login page after logout
history.push('/login');
};
return (
<div>
<h1>Welcome to the Dashboard</h1>
<button onClick={handleLogout}>Logout</button>
</div>
);
}
export default Dashboard;
Output :
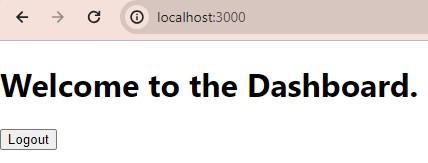
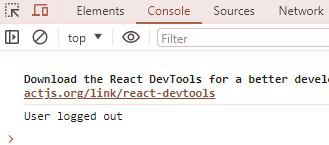
Console view
useRouteMatch
The “useRouteMatch” hook tries to find a match with the current URL and returns an object through which you can access information of the matched route. This information includes paths, url parameters and more.
*Note : “useRouteMatch()” hook will only work in the React-router-dom v5 but not in v6 , as it is replaced with “useMatch()” hook.
Example: Below is an example of useRouteMatch In the above example I’ve used a URL that is “/posts/:123” which is being compared with the ‘/posts/:postId ‘ using “useRouteMatch()” and returns the data present in it.
JavaScript
// App.js
import * as React from "react";
import {
BrowserRouter,
Route,
Routes
} from "react-router-dom";
import MyComponent from './MyComponent';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/posts/:postId"
element={<MyComponent />} />
</Routes>
</BrowserRouter>
);
}
export default App;
JavaScript
// MyComponent.js
import React from 'react';
import { useParams, useRouteMatch } from 'react-router-dom';
function MyComponent() {
const { postId } = useParams(); // Access URL parameter
const match = useRouteMatch('/posts/:postId'); // Match route pattern
// Display message if route doesn't match pattern
if (!match) {
return <div>This route does not match a blog post.</div>;
}
// Simulate fetching post data (replace with your actual logic)
const postData = {
id: postId,
title: `Blog Post`,
content: 'This is the content of the blog post.'
};
return (
<div>
<h1>PostId {postData.id}</h1>
<h1>{postData.title}</h1>
<p>{postData.content}</p>
</div>
);
}
export default MyComponent;
Output :
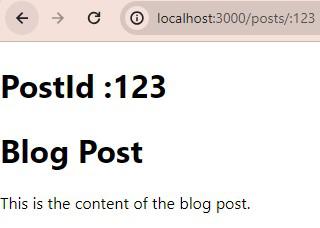
Share your thoughts in the comments
Please Login to comment...