JavaScript Strings
Last Updated :
12 Mar, 2024
What is String in JavaScript?
JavaScript String is a sequence of characters, typically used to represent text. It is enclosed in single or double quotes and supports various methods for text manipulation.
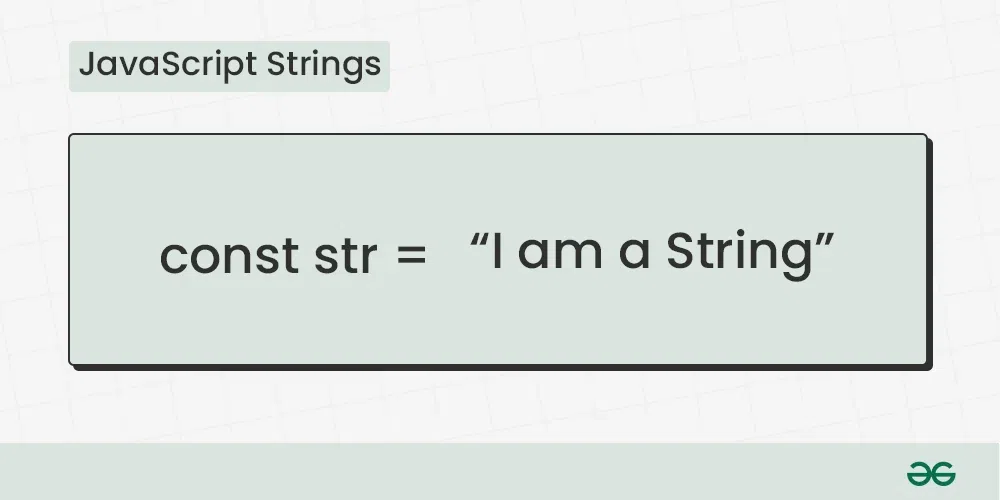
JavaScript String
You can create JavaScript Strings by enclosing text in single or double quotes. String literals and the String constructor provide options. Strings allow dynamic manipulation, making it easy to modify or extract elements as needed.
Basic Terminologies of JavaScript String
- String: A sequence of characters enclosed in single (‘ ‘) or double (” “) quotes.
- Length: The number of characters in a string, obtained using the length property.
- Index: The position of a character within a string, starting from 0.
- Concatenation: The process of combining two or more strings to create a new one.
- Substring: A portion of a string, obtained by extracting characters between specified indices.
Declaration of a String
1. Using Single Quotes
Single Quotes can be used to create a string in JavaScript. Simply enclose your text within single quotes to declare a string.
Syntax:
let str = 'String with single quote';
Example:
Javascript
let str = 'Create String with Single Quote' ;
console.log(str);
|
Output
Create String with Single Quote
2. Using Double Quotes
Double Quotes can also be used to create a string in JavaScript. Simply enclose your text within double quotes to declare a string.
Syntax:
let str = “String with double quote”;
Example:
Javascript
let str = "Create String with Double Quote" ;
console.log(str);
|
Output
Create String with Double Quote
3. String Constructor
You can create a string using the String Constructor. The String Constructor is less common for direct string creation, it provides additional methods for manipulating strings. Generally, using string literals is preferred for simplicity.
Javascript
let str = new String( 'Create String with String Constructor' );
console.log(str);
|
Output
[String: 'Create String with String Constructor']
4. Using Template Literals (String Interpolation)
You can create strings using Template Literals. Template literals allow you to embed expressions within backticks (`) for dynamic string creation, making it more readable and versatile.
Syntax:
let str = 'Template Litral String';
let newStr = `String created using ${str}`;
Example:
Javascript
let str = 'Template Litral String' ;
let newStr = `String created using ${str}`;
console.log(newStr);
|
Output
String created using Template Litral String
5. Empty String
You can create an empty string by assigning either single or double quotes with no characters in between.
Syntax:
// Create Empty Strign with Single Quotes
let str1 = '';
// Create Empty Strign with Double Quotes
let str2 = "";
Example:
Javascript
let str1 = '' ;
let str2 = "" ;
console.log( "Empty String with Single Quotes: " + str1);
console.log( "Empty String with Double Quotes: " + str2);
|
Output
Empty String with Single Quotes:
Empty String with Double Quotes:
6. Multiline Strings (ES6 and later)
You can create a multiline string using backticks (“) with template literals. The backticks allows you to span the string across multiple lines, preserving the line breaks within the string.
Syntax:
let str = `
This is a
multiline
string`;
Example:
Javascript
let str = `
This is a
multiline
string`;
console.log(str);
|
Output
This is a
multiline
string
Basic Operations on JavaScript Strings
1. Finding the length of a String
You can find the length of a string using the length property.
Example: Finding the length of a string.
Javascript
let str = 'JavaScript' ;
let len = str.length;
console.log( "String Length: " + len);
|
2. String Concatenation
You can combine two or more strings using + Operator.
Example:
Javascript
let str1 = 'Java' ;
let str2 = 'Script' ;
let result = str1 + str2;
console.log( "Concatenated String: " + result);
|
Output
Concatenated String: JavaScript
3. Escape Characters
We can use escape characters in string to add single quotes, dual quotes, and backslash.
Syntax:
\' - Inserts a single quote
\" - Inserts a double quote
\\ - Inserts a backslash
Example: In this example we are using escape characters
Javascript
const str1 = "\'GfG\' is a learning portal" ;
const str2 = "\"GfG\" is a learning portal" ;
const str3 = "\\GfG\\ is a learning portal" ;
console.log(str1);
console.log(str2);
console.log(str3);
|
Output
'GfG' is a learning portal
"GfG" is a learning portal
\GfG\ is a learning portal
4. Breaking Long Strings
We will use a backslash to break a long string in multiple lines of code.
Javascript
const str = "'GeeksforGeeks' is \
a learning portal" ;
console.log(str);
|
Output
'GeeksforGeeks' is a learning portal
Note: This method might not be supported on all browsers.
Example: The better way to break a string is by using the string addition.
Javascript
const str = "'GeeksforGeeks' is a"
+ " learning portal" ;
console.log(str);
|
Output
'GeeksforGeeks' is a learning portal
5. Find Substring of a String
We can extract a portion of a string using the substring() method.
Javascript
let str = 'JavaScript Tutorial' ;
let substr = str.substring(0, 10);
console.log(substr);
|
6. Convert String to Uppercase and Lowercase
Convert a string to uppercase and lowercase using toUpperCase() and toLowerCase() methods.
Javascript
let str = 'JavaScript' ;
let upperCase = str.toUpperCase();
let lowerCase = str.toLowerCase();
console.log(upperCase);
console.log(lowerCase);
|
Output
JAVASCRIPT
javascript
7. String Search in JavaScript
Find the index of a substring within a string using indexOf() method.
Javascript
let str = 'Learn JavaScript at GfG' ;
let searchStr = str.indexOf( 'JavaScript' );
console.log(searchStr);
|
8. String Replace in JavaScript
Replace occurrences of a substring with another using replace() method.
Javascript
let str = 'Learn HTML at GfG' ;
let newStr = str.replace( 'HTML' , 'JavaScript' );
console.log(newStr);
|
Output
Learn JavaScript at GfG
9. Trimming Whitespace from String
Remove leading and trailing whitespaces using trim() method.
Javascript
let str = ' Learn JavaScript ' ;
let newStr = str.trim();
console.log(newStr);
|
10. Access Characters from String
Access individual characters in a string using bracket notation and charAt() method.
Javascript
let str = 'Learn JavaScript' ;
let charAtIndex = str[6];
console.log(charAtIndex);
charAtIndex = str.charAt(6);
console.log(charAtIndex);
|
11. String Comparison in JavaScript
There are some inbuilt methods that can be used to compare strings such as the equality operator and another like localeCompare() method.
Javascript
let str1 = "John" ;
let str2 = new String( "John" );
console.log(str1 == str2);
console.log(str1.localeCompare(str2));
|
Note: The Equality operator returns true, whereas the localeCompare method returns the difference of ASCII values.
12. Passing JavaScript String as Objects
We can create a JavaScript string using the new keyword.
Javascript
const str = new String( "GeeksforGeeks" );
console.log(str);
|
Output
[String: 'GeeksforGeeks']
Are the strings created by the new keyword is same as normal strings?
No, the string created by the new keyword is an object and is not the same as normal strings.
Javascript
const str1 = new String( "GeeksforGeeks" );
const str2 = "GeeksforGeeks" ;
console.log(str1 == str2);
console.log(str1 === str2);
|
JavaScript String Complete Reference
We have created a complete list of article based on JavaScript String. Please go and check JavaScript String Reference for more detail. This article contains all string properties and methods with descriptions and running code examples.
Share your thoughts in the comments
Please Login to comment...