Reverse a String in JavaScript
Last Updated :
08 Feb, 2024
We have given an input string and the task is to reverse the input string in JavaScript. It is a very common question asked in a JavaScript interview. There are various methods to reverse a string in JavaScript, which are described below with examples.
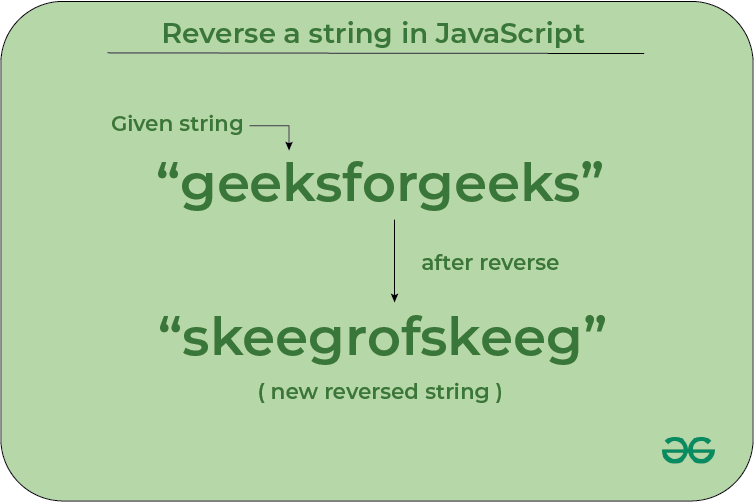
Reverse a String in JavaScript
Examples:
Input: str = "GeeksforGeeks"
Output: "skeeGrofskeeG"
Input: str = "Hello"
Output: "olleH"
Reverse a String in JavaScript
There are the common approaches to reverse a string in JavaScript. These are:
Reverse a String using reduce() and split() Methods
The split() method divides the string into an array of characters, and reduce() combines the characters in reverse order using the accumulator, effectively reversing the original string.
Example: The below code explains the use of the reduce and split method to reverse a string.
Javascript
function reverseString(str){
const reversedString =
str.split( "" ).reduce((acc, char) => char + acc, "" );
console.log(reversedString);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
The split() method divides the string into an array of characters, reverse() reverses the array, and join() combines the reversed characters into a new string, effectively reversing the original string.
Example: The below code uses the split(), reverse() and join() method to reverse a string in JavaScript.
javascript
function reverseString(str) {
const strRev = str.split( '' ).reverse().join( '' );
console.log(strRev);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
The spread operator(…) is used to spread the characters of the string str into individual elements. The reverse() method is then applied to reverse the order of the elements, and join() is used to combine the reversed elements back into a string.Â
Example: The below code uses the spread operator to split the string into a series of characters and reverse it.
javascript
function reverseString(str) {
const strRev = [...str].reverse().join( "" );
console.log(strRev);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
The Array.from() is used to convert the string into an array of individual characters. The reverse() method is then applied to reverse the order of the elements in the array. Finally, the join() is used to combine the reversed elements back into a string.
Example: The below code example explains the above approach practically.
Javascript
function reverseString(str) {
const strRev = Array.from(str).reverse().join( "" );
console.log(strRev);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
The spread operator can be used to convert a string into an array of characters and use reduce() function in JavaScript to make a reverse string from an array by concatenating the string in the forward direction.
Example: This code implements the spread operator and the reduce method to reverse a string.
Javascript
function reverseString(str) {
const strRev = [...str].reduce((x, y) => y.concat(x));
console.log(strRev);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
Reverse a String using for Loop
The for loop is used to iterate through the characters of the string in reverse order. Starting from the last character (str.length – 1) and character pushed to the new reverse string one by one.
Example: The below code implements the for loop to reverse a string.
Javascript
function reverseString(str) {
let strRev = "" ;
for (let i = str.length - 1; i >= 0; i--) {
strRev += str[i];
}
console.log(strRev);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
Reverse a String using substring() and a Decrementing Index
The substring() method is used to extract the character at index i and append it to the reversed string. The index i is then decremented.
Example: The below code implements the substring() method to reverse the string.
Javascript
function reverseString(str) {
let reversedStr = "" ;
let i = str.length - 1;
while (i >= 0) {
reversedStr += str.substring(i, i + 1);
i--;
}
console.log(reversedStr);
}
reverseString( "GeeksforGeeks" );
reverseString( "JavaScript" );
reverseString( "TypeScript" );
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
Reverse a String using Recursion
In a recursive approach a function repeatedly calls itself, taking the substring from the second character and concatenating it with the first character, until the base case is reached, reversing the string.
Example: This code implements the recursion to reverse a string in JavaScript.
Javascript
function strReverse(str) {
if (str === "" ) {
return "" ;
} else {
return strReverse(str.substr(1)) + str.charAt(0);
}
}
console.log(strReverse( "GeeksforGeeks" ));
console.log(strReverse( "JavaScript" ));
console.log(strReverse( "TypeScript" ));
|
Output
skeeGrofskeeG
tpircSavaJ
tpircSepyT
Share your thoughts in the comments
Please Login to comment...