How to read command line arguments in Node ?
Last Updated :
25 Jan, 2024
Command-line arguments (CLI) consist of text strings utilized to provide extra information to a program during its execution via the command line interface of an operating system. Node facilitates the retrieval of these arguments through the global object, specifically the process object.
Below are the examples that give a better understanding:
Example 1: The code captures and logs the command-line arguments passed to a Nodejs script using `process.argv`. It outputs the array, including the script name and additional arguments, with `console.log(arguments);`.
Javascript
var arguments = process.argv;
console.log(arguments);
|
Steps to run the app:
node index.js
Output: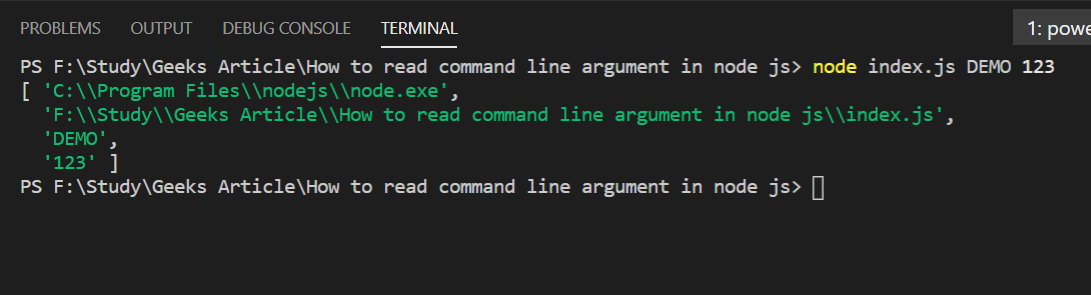
The process.argv
 contains an array where the 0th index contains the node executable path, 1st index contains the path to your current file and then the rest index contains the passed arguments.
Example 2: Program to add two numbers passed as arguments:
Javascript
var arguments = process.argv
function add(a, b) {
return parseInt(a)+parseInt(b)
}
var sum = add(arguments[2], arguments[3])
console.log("Addition of 2, 3 is ", sum)
|
Steps to run the app:
node index1.js 2 3
Output: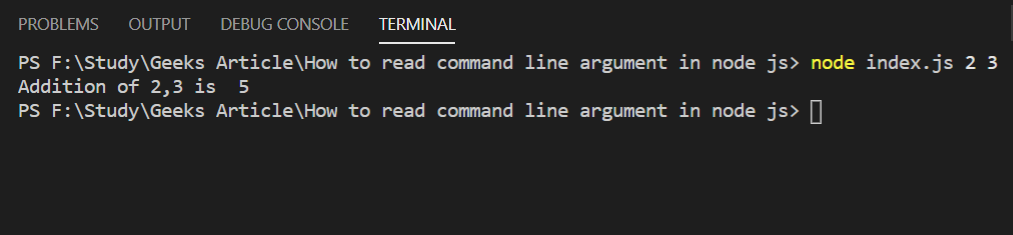
So this is how we can handle arguments in Node. The args module is very popular for handling command-line arguments. It provides various features like adding our own command to work and so on.
Share your thoughts in the comments
Please Login to comment...