How to build your own CLI (Command Line Interface) with Node.js ?
Last Updated :
25 Mar, 2022
Introduction: A command-line interface (CLI) is a text-based user interface (UI) for running programs, managing files, and interacting with computers. Building your own CLI is easier than you might think with Node.js. There are a bunch of open-source packages that can handle color, animation, and user input with ease. In this article, we will be building a simple CLI tool with the help of Node.js.
Prerequisites:
Steps to create the CLI:
Step 1: Create a folder for the project and open your favorite IDE (eg- VS Code, Atom, etc) within that folder.
Step 2: Open a terminal within the project folder and type npm init -y which will simply generate an empty npm project without going through an interactive process.
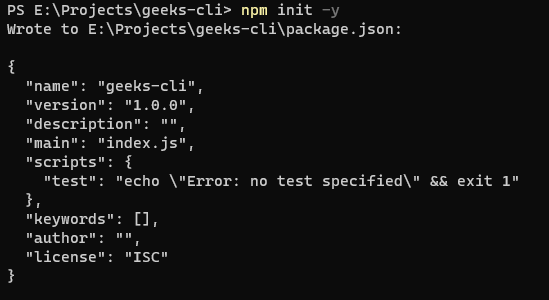
Step 3: Let’s install some open-sourced npm packages required for this project:
npm install figlet
npm install inquirer
npm install gradient-string
To know more about these packages, check out their official documentation.
Step 4: Create a file named index.js inside the project folder.
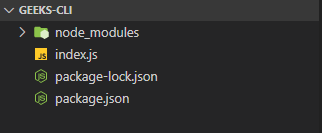
Step 5: Now, let’s write the following code inside the index.js file:
Approach: We will create only a single function to greet the user. Within the greet() function there will be three sections. First, we will display the “Geeks CLI” welcome message on the terminal. For this, we will be using the figlet package which is a program that generates texts based on ASCII characters. Secondly, we will prompt the user to enter his/her name in the terminal using the inquirer package and store it in a variable. Lastly, we will greet the user with his/her name and also color the message using the gradients-string package.
Javascript
import figlet from "figlet" ;
import inquirer from "inquirer" ;
import gradient from "gradient-string" ;
let userName;
const greet = async () => {
figlet('Geeks CLI ', function (err, data) {
console.log(data)
});
// Wait for 2secs
await new Promise(resolve => setTimeout(resolve, 2000));
// Ask the user' s name
const { name } = await inquirer.prompt({
type: "input" ,
name: "name" ,
message: "Enter your name?"
});
userName = name;
const msg = `Hello ${userName}!`;
figlet(msg, (err, data) => {
console.log(gradient.pastel.multiline(data));
});
}
greet();
|
Step 6: Let’s run the application. Type node index.js in the terminal.

Output:
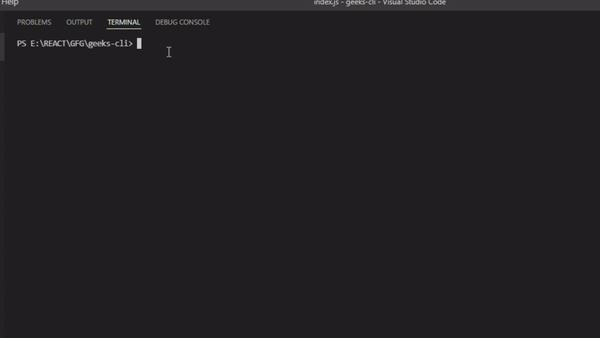
Geeks CLI Demo
Share your thoughts in the comments
Please Login to comment...