Node.js assert.match() Function
Last Updated :
21 Jun, 2022
The assert module provides a set of assertion functions for verifying invariants. The assert.match() function expects the string input to match the regular expression. If the condition is true it will not produce an output else an assertion error is raised.
Syntax:
assert.match(string, regexp[, message])
Parameters: This function accepts the following parameters as mentioned above and described below:
- string: This parameter holds the string value that need to be evaluated. It is of string type.
- regexp: This parameter is the regular expression which is matched against the given string value.
- message: This parameter holds the error message of string or error type. It is an optional parameter.
Return Value: This function returns assertion error of object type.
Installation of assert module:
npm install assert
- Note: Installation is an optional step as it is inbuilt Node.js module.
- After installing the assert module, you can check your assert version in command prompt using the command.
npm version assert
- After that, you can just create a folder and add a file for example, index.js as shown below.
Example 1: Filename: index.js
javascript
const assert = require( 'assert' ).strict;
try {
assert.match( 'I will try to pass' , /fail/);
} catch (error) {
console.log("Error:", error)
}
|
Steps to run the program:
- The project structure will look like this:
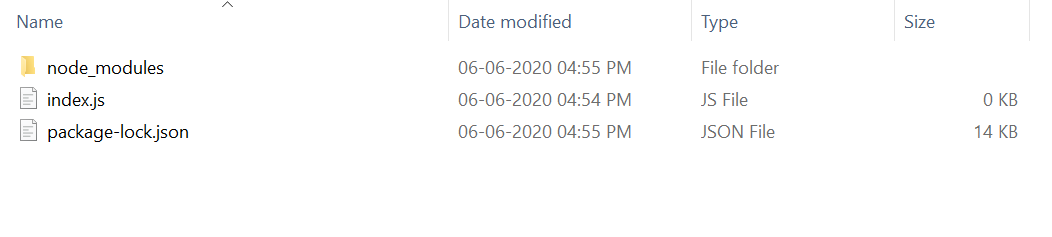
- Run index.js file using below command:
node index.js
Output:
Error: AssertionError [ERR_ASSERTION]: The input did not match the regular expression /fail/. Input: ‘I will try to pass’ at Object. (C:\Users\Lenovo\Downloads\index.js:14:12) at Module._compile (internal/modules/cjs/loader.js:1138:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1158:10) at Module.load (internal/modules/cjs/loader.js:986:32) at Function.Module._load (internal/modules/cjs/loader.js:879:14) at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:71:12) at internal/main/run_main_module.js:17:47 { generatedMessage: true, code: ‘ERR_ASSERTION’, actual: ‘I will try to pass’, expected: /fail/, operator: ‘match’ }
Example 2: Filename: index.js
javascript
const assert = require( 'assert' ).strict;
try {
assert.match( 'I am good' , /good/);
console.log("No Error Occurred")
} catch (error) {
console.log("Error:", error)
}
|
Steps to run the program:
- The project structure will look like this:
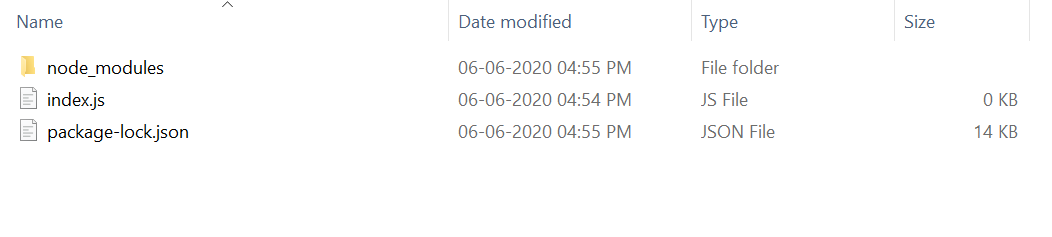
- Run index.js file using below command:
node index.js
Output:
No Error Occurred
Reference: https://nodejs.org/dist/latest-v12.x/docs/api/assert.html#assert_assert_match_string_regexp_message
Share your thoughts in the comments
Please Login to comment...