How to Fix – Page not found 404 django
Last Updated :
09 Oct, 2023
In the world of web development, handling these errors gracefully is crucial for providing a good user experience. In this article, we’ll explore how to handle 404 errors in Django, covering different possibilities and best practices.
Understanding the 404 Error in Django
Django, a famous Python web framework, offers a sturdy gadget for dealing with 404 errors. When a person requests a URL that Django can’t fit into any described view, it raises a Http404 exception. Django then searches for a custom 404 error handler to provide an extra user-pleasant response.
Handling 404 Page Not Found errors in Django is essential for imparting user-satisfactory and expert net software programs. By customizing your 404 errors web page, using Django’s built-in shortcuts like get_object_or_404, and imposing custom mistakes-handling logic at the same time as vital, you may make certain that users have a continuing display, even if they stumble upon missing or non-existent pages.
Method to Fix Page Not Found 404 Error in Django
Here’s how to handle 404 errors effectively in Django:
For Customize 404 Page
One of the most common approaches to handling 404 errors in Django is to create a custom 404 page. Here we have to make sure that the path and function are correct.
views.py: This code defines three view functions for a Django web application:
- home(request): Returns a “Hello, World!” response for the root URL.
- error_404(request, exception): Handles HTTP 404 errors (Not Found) and renders a 404 template.
- error_500(request): Handles HTTP 500 errors (Internal Server Error) and renders a 500 template (though there’s a potential typo in the template name).
Python3
from django.http import HttpResponse
from django.shortcuts import redirect, render
from django.db import models
def home(request):
return HttpResponse( 'Hello, World!' )
def error_404(request, exception):
print (hh)
return render(request, 'templates/505_404.html' , status = 404 )
def error_500(request):
return render(request, 'templates/505_404.html' , status = 500 )
|
templates/505_404.html: Create custom error HTML templates in the templates directory of your app. In this case, let’s create error_505.html
HTML
<!DOCTYPE html>
< html >
< head >
< title >404 or 505 Error</ title >
</ head >
< body >
< h1 >Page Not Found (404) or (505)</ h1 >
< p >The requested page does not exist.</ p >
</ body >
</ html >
|
urls.py: We set handler404 and handler500 to the custom error view functions we defined earlier (error_404 and error_500). Now, when a 404 or 500 error occurs in your Django project, Django will use the custom error views and render the corresponding custom error pages you’ve defined.
Python3
from django.contrib import admin
from django.urls import path, include
handler404 = 'views.error_404'
handler500 = 'views.error_500'
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', views.home, name=' home')
]
|
For more clear understanding you can visit our article – Python Django Handling Custom Error Page
Configure DEBUG Mode
In Django, the behavior of 404 errors can differ between development (DEBUG=True) and production (DEBUG=False) modes. In development mode, Django displays a detailed error page with debugging information, while in production mode, it serves the custom 404 page. Ensure that you configure the DEBUG setting appropriately in your project’s settings file.
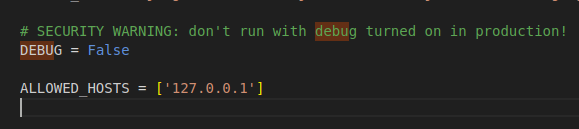
Using get_object_or_404
Django provides a helpful shortcut for handling 404 errors when querying objects in the database. If you expect a specific object to exist and want to return a 404 error if it doesn’t, you can use the get_object_or_404 function.
This function tries to retrieve the object with the given parameters. If it doesn’t find one, it raises a Http404 exception.
Python3
from django.shortcuts import get_object_or_404
from .models import YourModel
def detail_view(request, object_id):
obj = get_object_or_404(YourModel, pk = object_id)
|
Using raise Http404 Explicitly
In some cases, you may want to raise a 404 error explicitly within your views. You can do this by importing and raising the Http404 exception.
This allows you to handle 404 errors based on specific conditions within your views.
Python3
from django.http import Http404
def my_view(request):
if some_condition:
raise Http404( "This page does not exist." )
|
You can also use – Django shortcuts: get_list_or_404()
Share your thoughts in the comments
Please Login to comment...