How to create a Functional Component in React?
Last Updated :
06 Mar, 2024
To create a functional component in React, you define a JavaScript function that returns JSX. These components are stateless and rely on props for data input.
Functional components are concise, easy to read, and can utilize React Hooks for managing state and side effects, making them a fundamental building block for React applications.
What is a Functional Component?
In modern React development, a prevalent approach involves managing state using Hooks, such as useState. This method tends to result in shorter, more concise code. Unlike traditional approaches, it gives up the use of this keyword for accessing props and state, leading to cleaner and more readable code.
Steps to Create a Functional Component in React:
Step 1: Create a React application using the following command:
npx create-react-app my-app
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd my-app
Example of creating a Functional Component
Example 1: Below is an example to show the functional component in React.
Javascript
import React from "react" ;
function GeeksforGeeks() {
return (
<div>
<h2>
How to create a functional
component in React?
</h2>
<p>
GeeksforGeeks is a computer
science portal for geeks.
</p>
</div>
);
}
export default function App() {
return (
<div className= "App" >
<h2 style={{ color: 'green' }}>
GeeksforGeeks
</h2>
<GeeksforGeeks />
</div>
)
}
|
Output:

Example 2: In this example, we will create multiple functional components
Javascript
import React from "react" ;
function GeeksforGeeks() {
return (
<div>
<h2>
How to create a functional
component in React?
</h2>
</div>
);
}
function GeeksforGeeks2() {
return (
<div>
<p style={{ color: 'teal' }}>
GeeksforGeeks is a computer
science portal for geeks.
</p>
</div>
);
}
function GeeksforGeeks3() {
return (
<div>
This is Button coming from
Third functional component
<button>GFG</button>
</div>
);
}
export default function App() {
return (
<div className= "App" >
<h2 style={{ color: 'green' }}>
GeeksforGeeks
</h2>
<GeeksforGeeks />
<GeeksforGeeks2 />
<GeeksforGeeks3 />
</div>
)
}
|
Output:
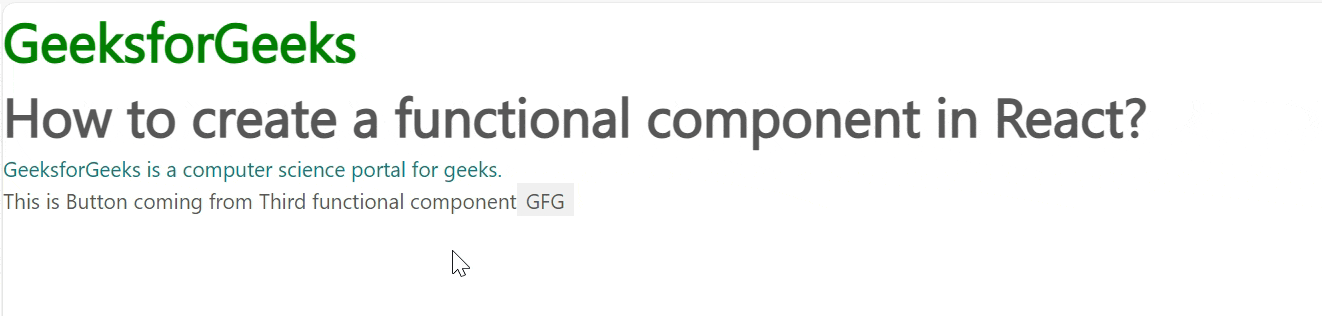
Share your thoughts in the comments
Please Login to comment...