How to Create Functional Components using Vue Loader ?
Last Updated :
21 Jun, 2023
Vue.js is a JavaScript framework used in building powerful and beautiful user interfaces. The key feature of Vue.js is its component-based architecture that allows the developers to create reusable and modular components. Functional components, in particular, provide a lightweight and efficient way to build UI elements.
In this article, we will learn how to create functional components using Vue Loader. Vue Loader is a webpack loader specifically designed to create the Vue.js functional components.
What are Functional Components?
Functional components in Vue.js are stateless which means they have only one file and are primarily used for rendering UI elements based on the given props. The Functional Component is also Instanceless, which means that it doesn’t have any instances, so doesn’t point to specific objects with the help of this keyword. These components are simple, performant, and have no internal state or lifecycle methods. Functional components are used when there is only a need to present data or UI elements without actually adding complex logic. To specify the template that is compiled as a functional component, we need to include the functional attribute of that specific template block, which allows us to remove the functional option in the <script> block.
Steps to Create Vue App:
Step 1: Install Vue modules using the below npm command
npm install vue
Step 2: Use Vue JS through CLI. Open your terminal or command prompt and run the below command.
npm install --global vue-cli
Step 3: Create the new project using the below command
vue init webpack vueproject
Step 4: Change the directory to the newly created project
cd vueproject
Project Structure: The following project structure will be generated after completing the above-required steps:
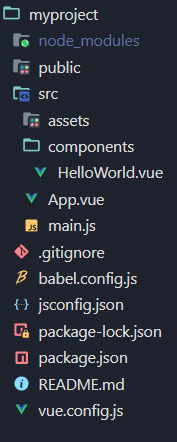
Steps to install the Vue Loader:
npm install --save-dev vue-loader
- Using Vue loader to create functional components:
<template>
<!-- Vue HTML template -->
</template>
<script>
export default {
// Logic for vue component
}
</script>
<style>
/* Add your CSS styles */
</style>
Example 1: Below example demonstrates a basic functional component created using Vue Loader.
Javascript
<template>
<div class= "func-loader-component" >
<h1 class= "header" >{{ header }}</h1>
<h3>{{ title }}</h3>
<h5>{{ about }}</h5>
</div>
</template>
<script>
export default {
name: 'ExampleComponent' ,
props: {
header: String,
title: String,
about: String,
},
}
</script>
<style scoped>
.func-loader-component {
padding: 10px;
}
.header {
color: green
}
</style>
|
Javascript
<template>
<div>
<example-component header= "GeeksforGeeks"
title= "Data Structures"
about= "Learn Data Structures and
Algorithms from India's best
coding platform." />
</div>
</template>
<script>
import ExampleComponent from
'./components/ExampleComponent.vue';
export default {
components: {
ExampleComponent,
},
}
</script>
|
Output:
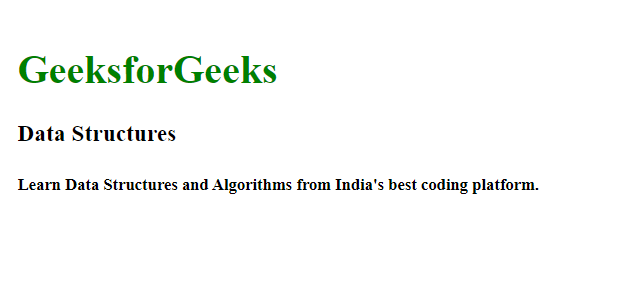
Example 2: Below example demonstrates a functional component having an external template and scoped styles created using the Vue Loader.
Javascript
<template src= "./MyTemplate.html" ></template>
<script>
export default {
name: 'ExampleComponent' ,
props: {
header: String,
title: String,
about: String,
},
}
</script>
<style scoped src= "./MyStyles.css" ></style>
|
HTML
< div class = "func-loader-component" >
< h1 class = "header" >{{ header }}</ h1 >
< h3 >{{ title }}</ h3 >
< h5 >{{ about }}</ h5 >
</ div >
|
CSS
.func-loader-component {
padding : 10px ;
background-color : rgb ( 231 , 231 , 237 );
}
.header {
color : green
}
|
Javascript
<template>
<div>
<example-component header= "GeeksforGeeks"
title= "Data Structures"
about= "Learn Data Structures and Algorithms
from India's best coding platform." />
</div>
</template>
<script>
import ExampleComponent from
'./components/ExampleComponent.vue';
export default {
components: {
ExampleComponent,
},
}
</script>
|
Output:
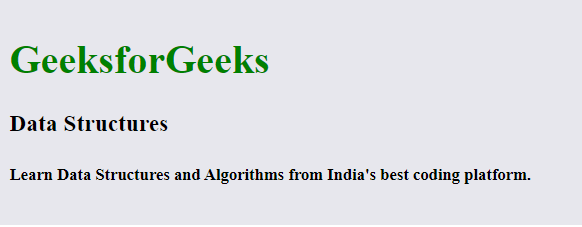
Reference: https://vue-loader.vuejs.org/guide/functional.html
Share your thoughts in the comments
Please Login to comment...