How does a pure component differ from a functional component?
Last Updated :
29 Feb, 2024
React provides two main types of components: pure components and functional components. Understanding the differences between these two types is important for effective React development.
In this article, we will learn about pure components and functional components, along with discussing the significant distinction that differentiates between pure components and functional components.
Pure Components:
A pure component is a class component that extends React’s PureComponent
class that automatically implements shouldComponentUpdate and performs a shallow comparison of props and state to determine if a re-render is necessary. Pure components are typically used to optimize performance by preventing unnecessary re-renders when the component’s props and state haven’t changed.
Syntax of Pure Components:
class PureExample extends React.PureComponent {
render() {
return <div>{this.props.text}</div>;
}
}
Features of Pure Components:
- Automatic Rendering Optimization: Pure components implement a shallow comparison of props and state. They only re-render when the data they receive changes, leading to improved performance by avoiding unnecessary renders.
- Immutable Props and State: Pure components treat props and state as immutable. This ensures that any changes to these values are detected accurately during the comparison process, facilitating efficient rendering updates.
- No Internal State: Pure components don’t manage internal state using the this.state mechanism. Instead, they rely solely on the props passed to them for rendering. This simplifies their behavior and makes them more predictable.
- Enhanced for Performance: Due to their inherent optimization features, pure components are well-suited for performance-critical scenarios. They are particularly beneficial in applications with large component trees or frequent updates to data.
Example: Write the following code in App.js file
Javascript
import React, { PureComponent } from 'react' ;
class MyPureComponent extends PureComponent {
render() {
return <div>Hello, I am a pure component!</div>;
}
}
export default MyPureComponent;
|
Output:
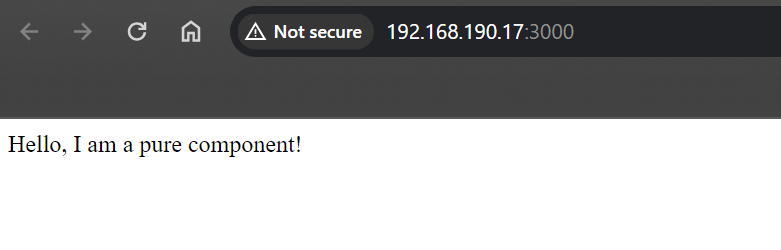
Functional Components:
A functional component is a JavaScript function that returns JSX (React elements). Functional components are primarily used for presentational purposes, and they are often referred to as “stateless” components because they don’t have their own internal state or lifecycle methods. With the introduction of React hooks, functional components can now also manage state and side effects using hooks like useState
, useEffect
, and others.
Syntax of Functional Components:
const FunctionalExample = ({ text }) => <div>{text}</div>;
Features of Functional Components:
- Simplicity: Functional components are simpler and more concise compared to class components. They rely solely on JavaScript functions for rendering UI elements, resulting in cleaner and easier-to-understand code.
- No ‘this’ Keyword: Functional components do not use the this keyword, eliminating the need for binding methods or accessing instance-specific data. This simplifies the component’s structure and reduces the potential for bugs related to this context.
- Hooks Support: Functional components fully support React Hooks, allowing developers to use state and other React features without writing class components. Hooks enable better code organization and reusability by promoting a more functional programming style.
- Improved Performance: With the introduction of React’s fiber architecture and the optimization of functional component rendering, functional components can offer comparable performance to class components. Additionally, functional components tend to have a smaller footprint,
Example: Write the following code in respective files
Javascript
import "./App.css" ;
import MyFunctionalComponent from "./MyFunctionalComponent" ;
export default function App() {
return (
<div className= "App" >
<MyFunctionalComponent />
</div>
);
}
|
Javascript
import React from 'react' ;
const MyFunctionalComponent = () => {
return <div>Hello, I am a functional component!</div>;
};
export default MyFunctionalComponent;
|
Output:
.png)
Functional Component Example output
Difference between Pure Components and Functional Components:
Feature
|
Pure Components
|
Functional Components
|
Implementation
|
Extends PureComponent class
|
Defined as JavaScript functions
|
Optimization
|
Automatically implements shouldComponentUpdate and shallow comparison
|
Doesn’t automatically optimize re-renders
|
State Management
|
Typically use props and state
|
Can use hooks for managing state and side effects
|
Re-render Behavior
|
Re-renders only when props or state change
|
Re-renders whenever props or state change
|
Conclusion:
While both pure components and functional components are important, they serve different purposes. Pure components focus on performance optimization through prop and state comparison, while functional components excel at simplicity, reusability, and now, with hooks, they offer powerful state management capabilities as well.
Share your thoughts in the comments
Please Login to comment...