How to use useMemo Hook in a Functional Component in React ?
Last Updated :
08 Mar, 2024
In React development, optimizing performance is crucial for delivering responsive and fast user experiences. One way to achieve this is by using the `useMemo` hook.
In this article, we’ll explore what `useMemo` is, why it’s beneficial, and how to use it effectively in functional components.
What is React useMemo?
`useMemo` is a React hook used for memoizing the result of expensive computations within functional components. It allows us to cache the value returned by a function and reuse it across renders, preventing unnecessary re-execution of the function.
Why do we use useMemo?
The primary purpose of `useMemo` is to optimize performance by avoiding redundant computations. When a component re-renders, any expressions within it, including function calls, are re-evaluated. If a function call involves expensive computations that don’t need to be recalculated on every render, using `useMemo` can significantly improve performance by memoizing the result and only recomputing it when dependencies change.
React useMemo: How to Cache the Value of Expensive Utilities?
To use `useMemo`, we provide it with a function and an array of dependencies. The function is called during rendering, and its return value is cached until one of the dependencies changes.
Syntax of useMemo Hook:
const memoizedValue = useMemo(() => {
// Expensive computation
}, [dependency1, dependency2, ...]);
Example: In this example we will see an optimizing expensive utility functions with React useMemo Hook.
Javascript
import React, { useState, useMemo, useEffect } from 'react' ;
const Memoized = () => {
const [count, setCount] = useState(0);
const expensiveOperation = useMemo(() => {
console.log( 'Creating expensive operation...' );
return () => {
console.log( 'Performing expensive operation...' );
let result = 0;
for (let i = 0; i < 1000000000; i++) {
result += i;
}
console.log( 'Expensive operation... Done' );
return result;
};
}, []);
useEffect(() => {
console.log( 'Component is rendered' );
});
useEffect(() => {
console.log( 'Executing expensive operation...' );
const result = expensiveOperation();
console.log( 'Expensive operation result:' , result);
}, [expensiveOperation]);
return (
<div>
<button onClick={() =>
setCount(count + 1)}>
Increment Count
</button>
<p>Count: {count}</p>
</div>
);
};
export default Memoized;
|
Output:
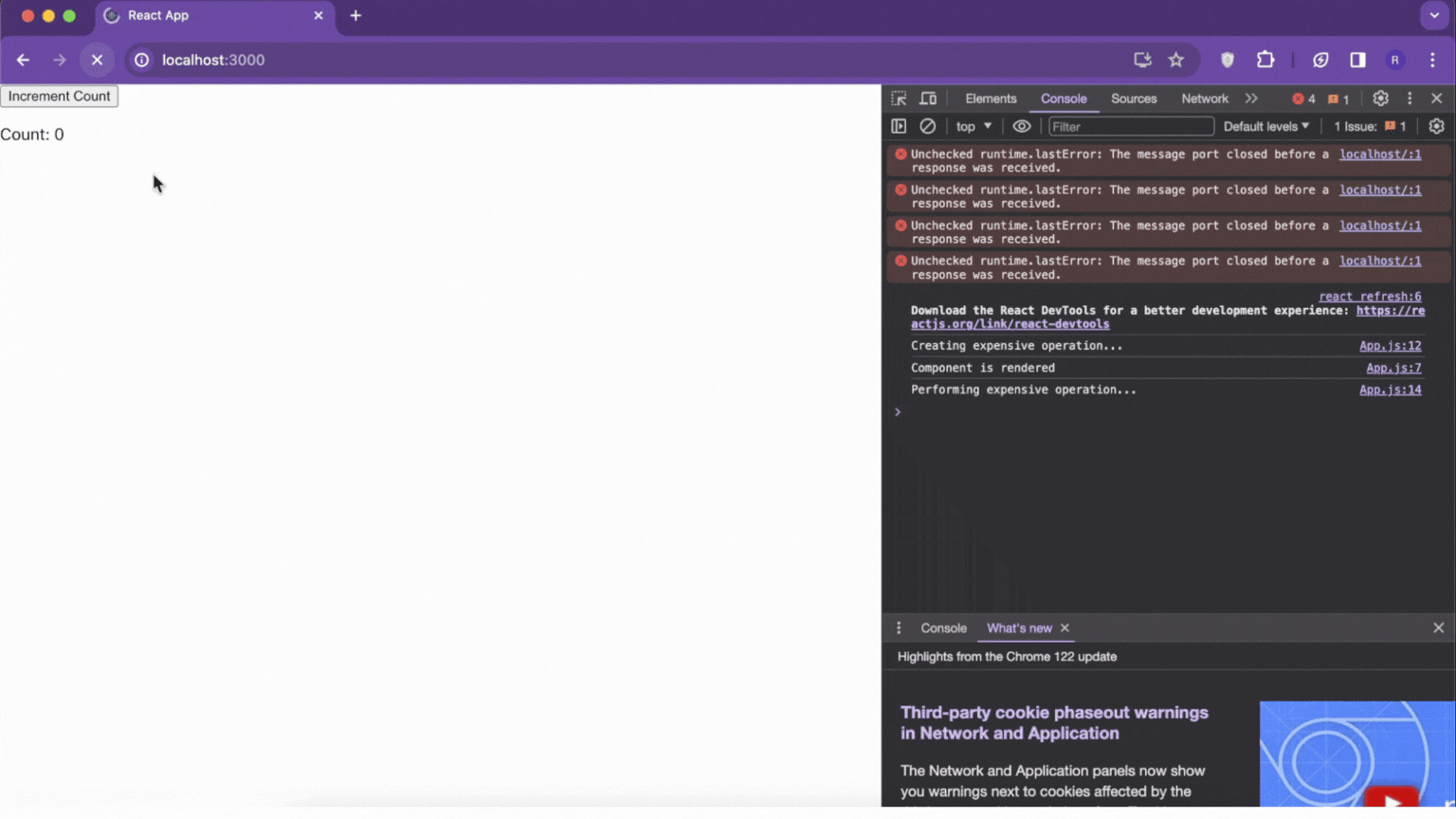
Output
Explanation of Output:
- We create a component called Memoized that keeps track of a count using useState.
- We use useMemo to remember the result of a big math calculation. This calculation happens only once due to empty dependency array.
- useEffect is used to trigger expensiveOperation for the calculation inside it.
- Now, whenever the component re-renders, the calculation is only done again if the expensiveOperation function changes.
Conclusion:
`useMemo` is a powerful tool in React for optimizing performance by memoizing the result of expensive computations within functional components. By caching the value of these computations and reusing them across renders, we can significantly improve the efficiency and responsiveness of our applications. When dealing with computationally intensive tasks or complex calculations, consider using `useMemo` to optimize your functional components and enhance the overall user experience.
Share your thoughts in the comments
Please Login to comment...