How to create Functional Components in React ?
Last Updated :
30 Jan, 2024
To create a functional component in React, you define a JavaScript function that returns JSX. These components are stateless and rely on props for data input. Functional components are concise, easy to read, and can utilize React Hooks for managing state and side effects, making them a fundamental building block for React applications.
Prerequisites:
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app my-app
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd my-app
Project Structure:
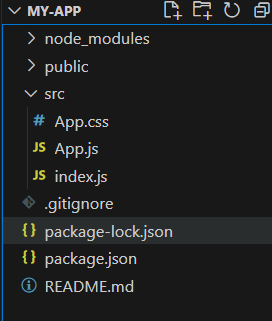
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Implementation to create a functional component.
Javascript
import React from "react" ;
that will be added to the application
function GeeksforGeeks() {
return (
<div>
<h1>
GeeksforGeeks
</h1>
<p>
GeeksforGeeks is a computer
science portal for geeks.
</p>
</div>
);
}
export default GeeksforGeeks;
|
Output:
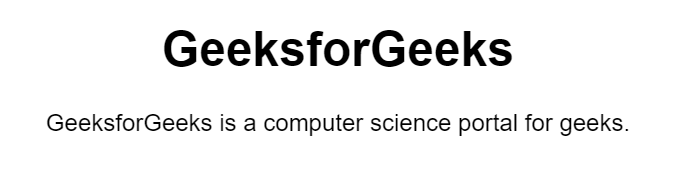
Example 2: Implemenattion to show how to add a functional component in our application with a custom input by changing the contents of the header in the App.js file.
Javascript
import React from "react" ;
function GeeksforGeeks(props) {
return (
<div>
<h1>
{props.name}
</h1>
</div>
);
}
export default GeeksforGeeks;
|
Javascript
import GeeksforGeeks from "./components/geeksforgeeks" ;
import "./styles.css" ;
export default function App() {
return (
<div className= "App" >
with different inputs
<GeeksforGeeks name= "GeeksforGeeks" />
<GeeksforGeeks name= "A computer science" />
<GeeksforGeeks name= "portal" />
<GeeksforGeeks name= "for geeks" />
</div>
);
}
|
Output:
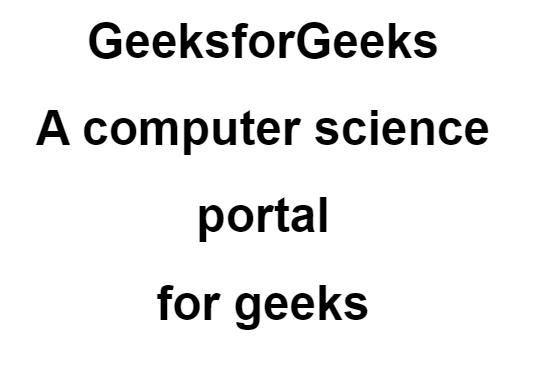
Step to run the application:Â Now to run the above code open the terminal and type the following command.
npm start
Share your thoughts in the comments
Please Login to comment...