How does useContext help with sharing data between components in React?
Last Updated :
19 Feb, 2024
useContext
is a hook in React that facilitates the sharing of data between components without the need to pass props manually through every level of the component tree. It allows components to consume data from a context that has been defined higher up in the component hierarchy.
How does it work?
- Centralized Data Storage:
- React context allows you to create a centralized data store that can hold values accessible by any component nested within the context provider.
- Avoiding Prop Drilling:
- With
useContext
, you can avoid “prop drilling” which is the process of passing props down through multiple levels of components just to reach a component that needs those values.
- Easy Access to Shared Data:
- Components can simply use the
useContext
hook to access the values stored in the context without needing to explicitly pass props through intermediate components.
- Simplifies Component Composition:
useContext
simplifies component composition by allowing you to separate concerns and keep your component tree clean and readable.
- Facilitates Global State Management:
- It’s particularly useful for managing global state or shared data that needs to be accessed by multiple components across your application.
- Dynamic Updates:
- When the context value changes, components that consume that context with
useContext
will automatically re-render to reflect the updated value.
useContext
promotes a more efficient and scalable way to share data between components by providing a simple and intuitive API for accessing shared values within the React context.
Example: Below is the example that sharing data between component in React with help of useContext.
Javascript
import React from 'react' ;
import AnotherComponent from './AnotherComponent' ;
import ThemedComponent from './ThemeComponent' ;
import ThemeProvider from './ThemeProvider' ;
const App = () => {
return (
<ThemeProvider>
<ThemedComponent />
<AnotherComponent />
</ThemeProvider>
);
};
export default App;
|
Javascript
import React, { createContext } from 'react' ;
const ThemeContext = createContext();
export default ThemeContext;
|
Javascript
import React, { useState } from 'react' ;
import ThemeContext from './ThemeContext' ;
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState( 'light' );
return (
<ThemeContext.Provider
value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
};
export default ThemeProvider;
|
Javascript
import React, { useContext } from 'react' ;
import ThemeContext from './ThemeContext' ;
const ThemedComponent = () => {
const { theme, setTheme } = useContext(ThemeContext);
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light' );
};
return (
<div style={{
backgroundColor: theme === 'dark' ?
'black' : 'white' , color: theme === 'dark' ?
'white' : 'black'
}}>
<p>Current theme: {theme}</p>
<button onClick={toggleTheme}>
Toggle Theme
</button>
</div>
);
};
export default ThemedComponent;
|
Javascript
import React, { useContext } from 'react' ;
import ThemeContext from './ThemeContext' ;
const AnotherComponent = () => {
const { theme } = useContext(ThemeContext);
return (
<div>
<p>This component also knows
the current theme: {theme}
</p>
</div>
);
};
export default AnotherComponent;
|
Output:
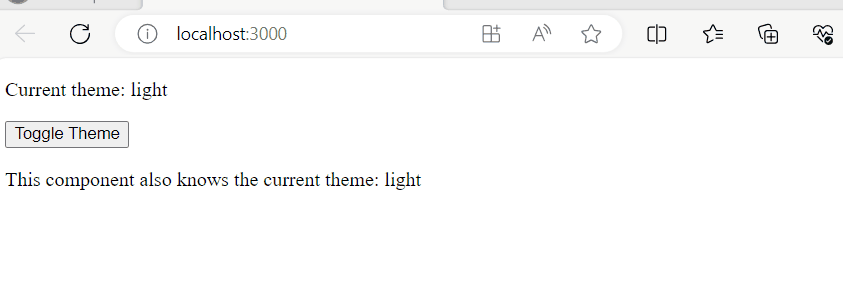
Output
Share your thoughts in the comments
Please Login to comment...