How does useImperativeHandle help parent and child components communicate?
Last Updated :
19 Feb, 2024
useImperativeHandle
in React allows parent and child components to communicate more effectively by enabling the child component to specify which methods and properties should be accessible to the parent through a ref.
How Does It Help:
- Customized Interaction: Child components can define a specific set of functions or data they want to share with their parent. This tailored approach ensures that only relevant information is exposed, enhancing clarity and simplicity in communication.
- Passing Refs: Parents can create a ref and pass it down to child components. With
useImperativeHandle
, child components can then determine what parts of their functionality or state should be accessible through this ref, granting parent-controlled access to specific features.
- Improved Performance: By limiting the exposed functionality to only what’s necessary, unnecessary re-renders and updates in the parent component can be avoided. This optimization helps maintain a smoother user experience, especially in situations where the child component’s internal state changes frequently.
- Encourages Encapsulation:
useImperativeHandle
encourages encapsulation by allowing child components to hide their implementation details while offering a clear and concise interface to the parent. This promotes modular and maintainable code, making it easier to manage and understand.
Example: Below is an example to help parent and child components communicate using useImperativeHandle.
Javascript
import React, {
useRef,
useImperativeHandle,
forwardRef
} from 'react' ;
const ChildComponent =
forwardRef((props, ref) => {
const inputRef = useRef();
useImperativeHandle(ref, () => ({
focusInput: () => {
inputRef.current.focus();
}
}));
return <input ref={inputRef} />;
});
const ParentComponent = () => {
const childRef = useRef();
const handleClick = () => {
childRef.current.focusInput();
};
return (
<div>
<ChildComponent ref={childRef} />
<button onClick={handleClick}>
Focus Input
</button>
</div>
);
};
export default ParentComponent;
|
Steps to Run the App:
npm start
Output:
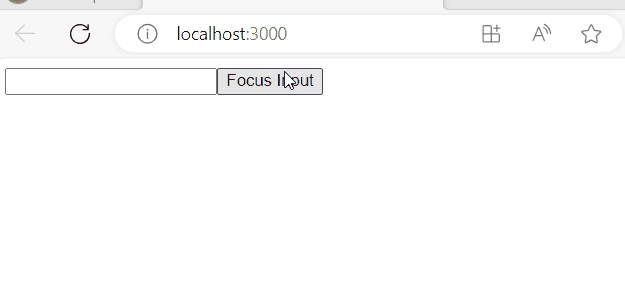
Output
Share your thoughts in the comments
Please Login to comment...