How to handle form data in Express ?
Last Updated :
04 Feb, 2024
Handling form data in Express involves setting up a route to manage incoming form submissions and using the body-parser middleware to extract the form data from the request body.
Steps to Handle form data in Express:
Step 1: Install the necessary package in your application using the following command.
npm install express body-parser
Step 2: Create your server file ‘server.js’ and html file ‘index.html’.
Example: Below is the example to show how you handle form data in ExpressJS.
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const app = express();
const PORT = 3000;
app.use(bodyParser.urlencoded({ extended: true }));
app.use(express.static( 'public' ));
app.get( '/' , (req, res) => {
res.sendFile(__dirname + '/index.html' );
});
app.post( '/submit' , (req, res) => {
const data = req.body;
console.log(data);
res.send(`<h1 style= "text-align: center;
margin-top: 50vh; transform: translateY(-50%);" >
Form submitted successfully!</h1>`);
});
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Handle Form Data</ title >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< h1 >Handle Form Data</ h1 >
< form action = "/submit" method = "post" >
< label for = "name" >Name:</ label >
< input type = "text" id = "name" name = "name"
placeholder = "Enter Your Name" required>
< br >
< label for = "email" >Email:</ label >
< input type = "email" id = "email" name = "email"
placeholder = "Enter Your Email" required>
< br >
< button type = "submit" >Submit</ button >
</ form >
</ body >
</ html >
|
CSS
body {
font-family : 'Arial' , sans-serif ;
background-color : #2bd279 ;
margin : 0 ;
padding : 0 ;
}
h 1 {
text-align : center ;
color : #333 ;
}
form {
max-width : 400px ;
margin : 20px auto ;
background-color : #fff ;
padding : 20px ;
border-radius: 5px ;
box-shadow: 0 0 10px rgba( 0 , 0 , 0 , 0.1 );
}
label {
display : block ;
margin-bottom : 8px ;
color : #333 ;
}
input {
width : 100% ;
padding : 8px ;
margin-bottom : 16px ;
box-sizing: border-box;
}
button {
background-color : #2822d1 ;
color : #fff ;
padding : 10px 15px ;
border : none ;
border-radius: 7px ;
cursor : pointer ;
}
button:hover {
background-color : #0f1e83 ;
}
|
Start your app using the following command.
node server.js
Output:
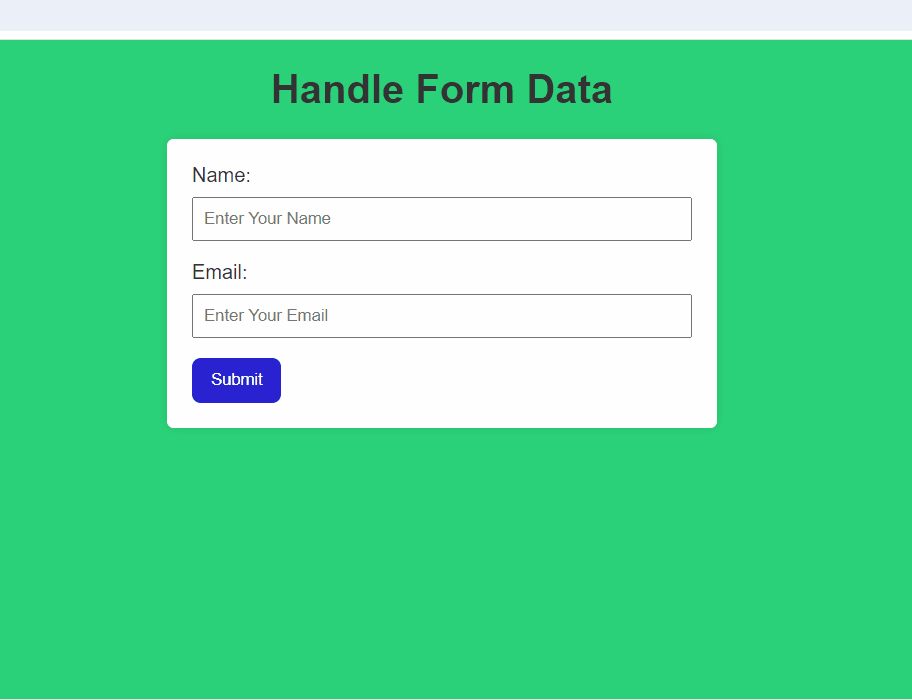
Share your thoughts in the comments
Please Login to comment...