How does useReducer help manage complex state in React components?
Last Updated :
22 Feb, 2024
useReducer
is a React hook that provides an alternative approach to managing state in components compared to useState
. While useState
is easy and suitable for managing simple state updates, useReducer
and is particularly helpful for managing more complex state logic.
Managing Complex State in React with useReducer:
- Centralized State Logic: With useReducer, complex state logic is consolidated into a single reducer function, enhancing code organization.
- Predictable State Updates: Actions dispatched to the reducer provide a clear and predictable way to modify the state, aligning with Redux principles for debuggable code.
- Complex State Structures: useReducer is well-suited for managing intricate state structures, offering flexibility in handling nested updates.
- Easier Testing: Encapsulating state logic within the reducer simplifies testing, enabling isolated testing of state modifications.
- Performance Optimization: useReducer may optimize performance, particularly with deep state nesting or frequent updates, by efficiently propagating state changes.
Example: Below is an example of managing complex states in React with useReducer.
Javascript
import React, {
useReducer
} from 'react' ;
import './App.css' ;
const reducer = (state, action) => {
switch (action.type) {
case 'INCREMENT' :
return {
...state,
count: state.count + 1
};
case 'DECREMENT' :
return {
...state,
count: state.count - 1
};
default :
return state;
}
};
const App = () => {
const initialState = { count: 0 };
const [state, dispatch] =
useReducer(reducer, initialState);
return (
<div className= "counter" >
<h2>Count: {state.count}</h2>
<button
onClick={() => dispatch({
type: 'INCREMENT'
})}>
Increment
</button>
<button
onClick={() => dispatch({
type: 'DECREMENT'
})}>
Decrement
</button>
</div>
);
}
export default App;
|
CSS
. counter {
font-family : Arial , sans-serif ;
text-align : center ;
margin : 20px auto ;
padding : 20px ;
border : 2px solid #ccc ;
border-radius: 5px ;
width : 200px ;
}
. counter h 2 {
font-size : 24px ;
margin-bottom : 10px ;
}
. counter button {
background-color : #007bff ;
color : #fff ;
border : none ;
padding : 8px 16px ;
margin : 2px 5px ;
cursor : pointer ;
border-radius: 5px ;
transition: background-color 0.3 s;
}
. counter button:hover {
background-color : #0056b3 ;
}
. counter button:active {
transform: translateY( 1px );
}
|
Output:
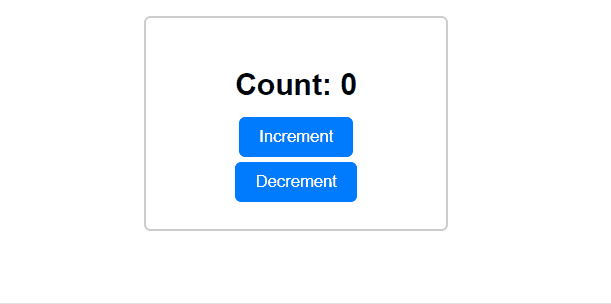
Output
Share your thoughts in the comments
Please Login to comment...