How is useRef different from using ‘ref’ with class components?
Last Updated :
22 Feb, 2024
In class components, ref
is a special attribute used to reference DOM elements or React components. You create a ref in the constructor, attach it to the element, and then manipulate it using the ref object. ReactJS
useRef
is a hook in functional components for referencing DOM elements or other values. It returns a mutable object that you attach to the element, allowing you to access and modify it directly.
Syntax:
- With class components, you use the
ref
attribute directly within JSX elements
<input ref={this.myRef} />
- With functional components, you use the
useRef
hook.
const myRef = useRef();
Ref Management in Class and Functional Components:
- Accessing the Ref Object:
- In class components, you access the
ref
object using this.myRef
.
- In functional components, you access the
ref
object directly as myRef.current
.
- Managing Lifecycle:
- In class components, you can access the lifecycle methods like
componentDidMount
, componentDidUpdate
, etc., to manipulate the ref.
- In functional components, you don’t have access to lifecycle methods directly. Instead, you might use
useEffect
hook to perform actions after render.
- Usage with DOM Elements:
- Both
useRef
and ref
can be used to reference DOM elements. However, with useRef
, you access the DOM element via current
property, while with ref
in class components, you directly access the DOM element via the ref object.
Example 1: Below is the example of ref in React using class component.
Javascript
import React,
{
Component
} from 'react' ;
class FocusInputClass extends Component {
constructor(props) {
super (props);
this .textInput = React.createRef();
}
focusInput = () => {
this .textInput.current.focus();
};
render() {
return (
<div>
<input type= "text"
ref={ this .textInput} />
<button onClick={ this .focusInput}>
Focus Input
</button>
</div>
);
}
}
export default FocusInputClass;
|
Output:
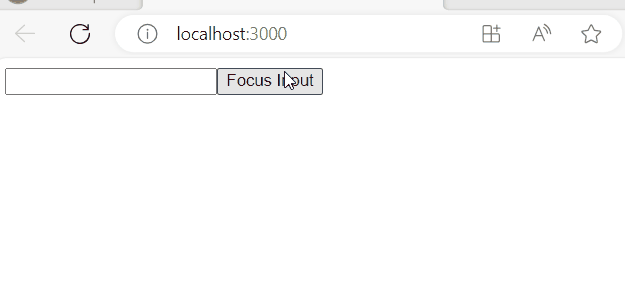
Output
Example 2: Below is the example of useRef in React using functional component.
Javascript
import React,
{
useRef
} from 'react' ;
const FocusInputFunctional = () => {
const textInput = useRef( null );
const focusInput = () => {
textInput.current.focus();
};
return (
<div>
<input type= "text"
ref={textInput} />
<button onClick={focusInput}>
Focus Input
</button>
</div>
);
};
export default FocusInputFunctional;
|
Output:
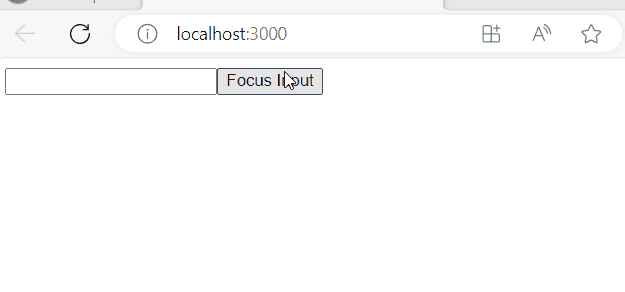
Output
Share your thoughts in the comments
Please Login to comment...