How can you use useContext to consume values from a React context?
Last Updated :
15 Apr, 2024
ReactJS provides Context API to manage the state at a global level in your application. It acts as a container where you can store multiple states, and functions and access them from anywhere in the application by using the useContext hook. In this article, we are going to learn how to use the useContext hook with examples and syntax.
What is useContext?
useContext is a hook that provides a way to pass data through the component tree without manually passing props down through each nested component. It is designed to share data that can be considered global data for a tree of React components, such as the current authenticated user or theme(e.g. color, paddings, margins, font-sizes).
Approach to use useContext:
We have created a Globaldata context using the createContext() function and exported it to be used in other components. We have created a state to store the data and stored that state and its function in a react context by using <Globaldata.Provider value={{….}}>.
We are accessing this react context in a child component by using useContext hook as a function and we are passing our Globaldata context that we have created in a parent component.
Syntax:
import { createContext } from "react";
export const contextName = createContext();
To provide a react Context Data to Application (create this at root level component):
Syntax:
<ContextName.Provider value={{....}}>
...other components...
</ContextName.Provider>
To access Context data:
Syntax:
import { useContext } from "react";
import { ContextName } from "./component";
const { ...... } = useContext(ContextName);
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app gfg
Step 2: After creating your project folder(i.e. gfg), move to it by using the following command:
cd gfg
Project Structure:

Example: The below example is demonstrating the use of useContext hook in ReactJS
Javascript
//src/App.js
import { createContext, useState } from "react";
import Child from "./Child";
export const Globaldata = createContext();
const App = () => {
const [name, setName] = useState("GFG");
return (
<Globaldata.Provider value={{ name, setName }}>
<span>Parent Component</span> <br />
<button onClick={() => setName("GeeksForGeeks")}>
Change Name to GeeksForGeeks
</button>
<hr />
<Child />
</Globaldata.Provider>
);
};
export default App;
JavaScript
//src/Child.js
import { useContext } from "react";
import { Globaldata } from "./App";
const Child = () => {
const { name, setName } = useContext(Globaldata);
return (
<>
<span>Child Component</span> <br />
<button onClick={() => setName("GFG")}>
Change Name to GFG
</button>
<h1>Context Data: {name}</h1>
</>
);
}
export default Child;
To run the application use the following command:
npm run start
Output: Now go to http://localhost:3000 in your browser:
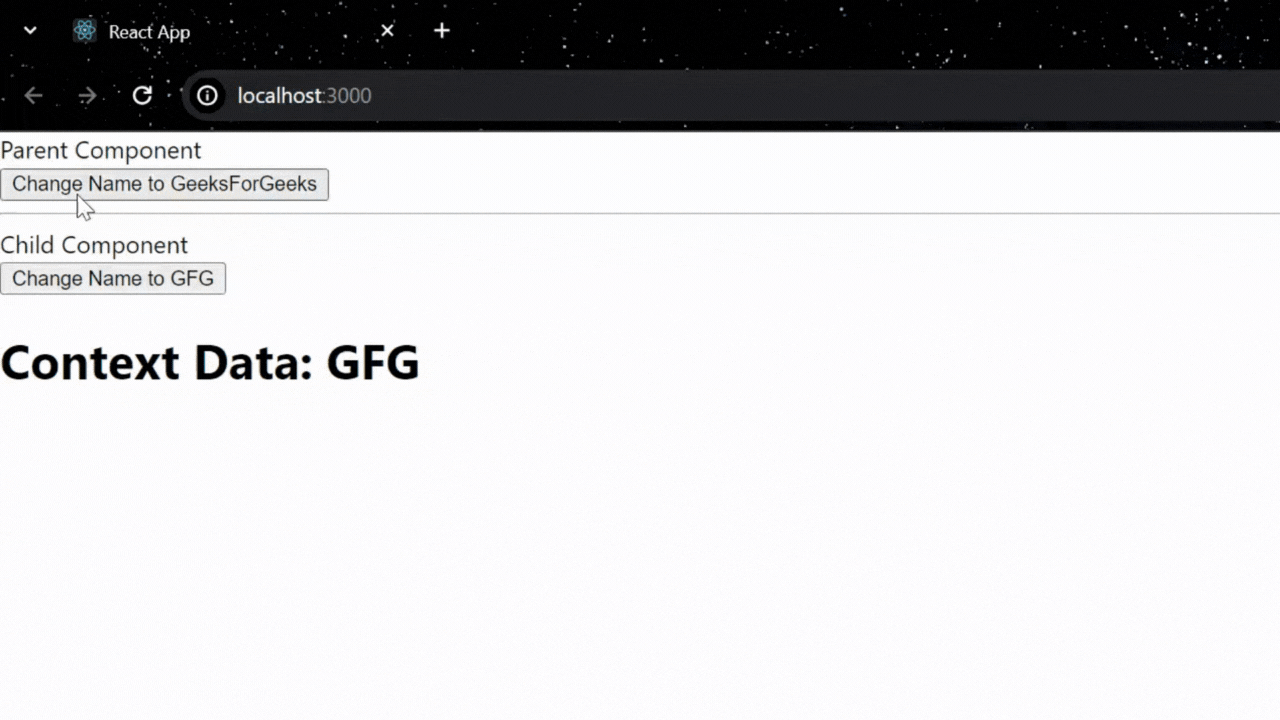
Share your thoughts in the comments
Please Login to comment...