Context API with useContext Hook
Last Updated :
19 Feb, 2024
React Context API is a very helpful feature that enables the sharing of state across components without the need for prop drilling. It simplifies state management and makes it easier to pass data down the component tree. In this article we will explore the Context API and demonstrate how to use it with the “useContext” hook through practical examples.
Prerequisites:
What is Context API?
Context in React provides a way to pass data through the component tree without having to pass props down manually at every level. It’s a state management solution that is very helpful but its only drawback is we can’t avoid extra re-rendering with this.
Key Features:
- Global State Management: Context allows you to create a global state that can be accessed by any component within the application.
- Avoids Prop Drilling: Prop drilling occurs when you pass down props through multiple levels of nested components even when intermediate components do not use the props. This makes the application fast.
- Provider-Consumer Model: Context provides a Provider component that wraps the part of the component tree where you want to share data and the Consumer component to access the shared data.
- Dynamic Updates: Context allows for dynamic updates of shared data. When the context value changes, components that depend on it will automatically re-render.
Syntax:
The Context API in React uses two main components “createContext” and the “useContext” hook.
- createContext Hook: It is used to create a new context object.
const YourContext = createContext(defaultValue);
- useContext Hook:It is used to access data from a context within a component.
const contextValue = useContext(YourContext);
Steps To Create React Application:
Step 1: Create a new react app using the following command.
npx create-react-app my-react-app
Step 2: Navigate to the root directory of your project using the following command.
cd my-react-app
Project Structure:
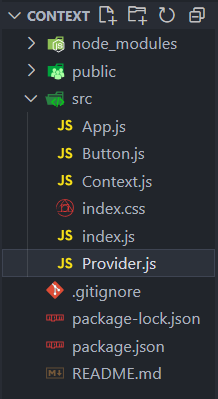
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: In this example we will create a theme-switching functionality where a button in one component can toggle between light and dark themes.
Javascript
import { createContext } from "react" ;
const Context = createContext();
export default Context;
|
Javascript
import React, {
useState
} from "react" ;
import Context from "./Context" ;
const Provider = ({ children }) => {
const [theme, setTheme] = useState( "light" );
const toggleTheme = () => {
setTheme((prevTheme) =>
(prevTheme === "light" ? "dark" : "light" ));
};
return (
<Context.Provider
value={{ theme, toggleTheme }}>
{children}
</Context.Provider>
);
};
export default Provider;
|
Javascript
import React, {
useContext
} from "react" ;
import Context from "./Context" ;
const Button = () => {
const { theme, toggleTheme } = useContext(Context);
return (
<button
style={{
backgroundColor: theme === "light" ?
"#ffffff" : "#333333" ,
color: theme === "light" ?
"#333333" : "#ffffff" ,
}}
onClick={toggleTheme}
>
Toggle Theme
</button>
);
};
export default Button;
|
Javascript
import React from "react" ;
import Provider from "./Provider" ;
import Button from "./Button" ;
const App = () => {
return (
<Provider>
<div>
<h1>Themed App</h1>
<Button />
</div>
</Provider>
);
};
export default App;
|
Output:
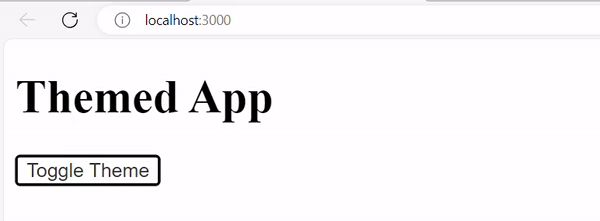
Share your thoughts in the comments
Please Login to comment...