Advantages of using Functional Components with React Hooks
Last Updated :
02 Apr, 2024
In the past, React mainly used class-based components as its building blocks. However, functional components, which are essentially JavaScript functions that receive props (inputs) and return JSX (rendered content), were limited in their capabilities.
With React 16.8 and the introduction of hooks, functional components gained access to features like managing state and handling side effects, previously exclusive to class components. This filled a gap in functionality and made functional components more powerful and versatile in React development.
What are React Hooks?
React Hooks introduced in React 16.8, developers can now access features like state and other functionalities (like handling side effects and context) in Functional Components, which were previously only available in class components. This allows for clearer and potentially more efficient code, as functional components can now have the same capabilities as class components.
Features of React Hooks:
- Improved Readability and Maintainability: Clear and easy-to-read code fosters better code health by enhancing readability, simplifying maintenance tasks, and lowering the risk of errors and bugs.
- Enhanced Reusability: Separate your code into specific hooks and reusable functions to simplify sharing logic across your application, promoting code reusability and easier maintenance.
- Simpler Component Structure: Centralized hooks streamline tasks like data fetching and side effect management, providing a structured approach that simplifies system organization and maintenance.
- Potential Performance Gains: Functional components using hooks can offer slightly better performance than class components in certain situations, contributing to a more efficient application.
- Easier Testing: It is usually easier to test functional components using standard testing libraries.
Advantages of Using React Hooks:
1. Simplified Code: Hooks simplify functional components by eliminating repetitive boilerplate code, making them easier to understand and maintain. This leads to clearer and more concise code, improving overall code quality and developer productivity.
Class component with state management:
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
// ... rest of component
}
Functional component with Hooks (useState) for the same functionality:
function Counter() {
const [count, setCount] = React.useState(0);
// ... rest of component
}
2. Improved Reusability: Custom hooks are reusable functions created using hooks in React. They encapsulate specific logic or functionality that can be shared across different components, reducing redundancy and promoting code reusability. By using custom hooks, developers can modularize their codebase, making it easier to maintain and scale their applications. This approach also helps in keeping the codebase organized and improving overall development efficiency.
Custom hook for data fetching:
function useFetch(url) {
const [data, setData] = React.useState(null);
const [loading, setLoading] = React.useState(true);
// ... implementation for fetching data
return { data, loading };
}
Using the custom hook in a component:
function ExampleComponent() {
const { data, loading } = useFetch('https://api.example.com/data');
// ... rest of component
}
3. Easier Testing and Debugging: Functional components with Hooks are simpler to test and debug due to their functional programming style and clear separation of concerns. This makes it easier to identify and fix issues during testing and debugging, improving overall code quality and development efficiency.
4. Reduced Bundle Size: Functional components using Hooks tend to have smaller file sizes, which means faster loading times for users compared to class components. This optimization can enhance the user experience by reducing waiting times and making the application feel more responsive overall.
Examples
Functional Component with Hooks
JavaScript
import React, { useState, useEffect } from 'react';
const FunctionalComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
};
export default FunctionalComponent;
Class Component(Equivalent)
JavaScript
import React, { Component } from 'react';
class ClassComponent extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
componentDidMount() {
document.title = `You clicked ${this.state.count} times`;
}
componentDidUpdate() {
document.title = `You clicked ${this.state.count} times`;
}
render() {
return (
<div>
<p>You clicked {this.state.count} times</p>
<button onClick={
() => this.setState({ count: this.state.count + 1 })
}>
Click me
</button>
</div>
);
}
}
export default ClassComponent;
Output:
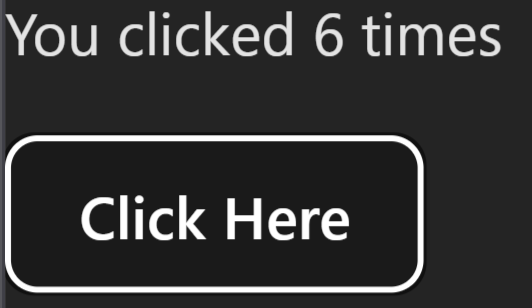
Output
Differences between class components vs functional components:
Feature
| Functional Component
| Class Component
|
---|
Definition
| JavaScript function.
| Extends React.Component.
|
---|
State Management
| Uses useState hook for state management.
| Uses this.state and this.setState .
|
---|
Lifecycle Methods
| No direct access.
| Has lifecycle methods like componentDidMount.
|
---|
Reusability
| Generally more reusable due to stateless nature.
| Can be reusable, but complexity might increase.
|
---|
Testing
| Easier to test due to simpler structure.
| Can be more challenging due to lifecycle methods.
|
---|
Context and Refs
| No bindings or this context needed for most cases.
| Requires bindings and this context .
|
---|
Error Handling
| Error boundaries and hooks like useEffect for errors.
| Error boundaries and lifecycle methods used.
|
---|
Conclusion
React Hooks simplify React app development by making code cleaner, encouraging code reuse, enabling better testing, and potentially reducing app size. These advantages make them crucial tools for modern React development, improving efficiency and code maintainability.
Share your thoughts in the comments
Please Login to comment...