How to handle sessions in Express ?
Last Updated :
19 Feb, 2024
ExpressJS is a small framework that works on top of Node web server functionality to simplify its APIs and add helpful new features. It makes it easier to organize your application’s functionality with middleware and routing. It adds helpful utilities to Node HTTP objects and facilitates the rendering of dynamic HTTP objects.
To handle sessions in Express JS:
- Use Session Middleware: Start by installing and configuring a session middleware for ExpressJS, such as
express-session
.
- Require the Middleware: In your ExpressJS application, require the session middleware and initialize it by passing a configuration object.
- Session Configuration: Set up the session configuration, including options like secret key, session expiration, and cookie settings.
- Middleware Integration: Add the session middleware to your ExpressJS middleware stack using the
app.use()
method. This ensures that session functionality is available throughout your application.
- Session Data Access: Access session data within your routes and middleware using the
req.session
object. You can store and retrieve user-specific data in the session object, such as user authentication status or preferences.
- Session Management: Implement logic to manage session data, such as creating a session upon user login, updating session data during user interactions, and destroying the session upon user logout or inactivity.
- Security Considerations: Ensure that session-related data, such as session IDs and sensitive user information, are handled securely to prevent session hijacking and other security vulnerabilities. Use secure cookies, HTTPS, and other best practices to protect session data.
- Testing: Test your session handling functionality thoroughly to ensure it works as expected. Use tools like Postman or browser testing to simulate user interactions and verify session behavior.
Syntax:
npm install express-session
- Set up the
express-session
middleware in your ExpressJS application. This middleware creates a session object on the req
(request) object, which you can use to store session data:
const express = require('express');
const session = require('express-session');
const app = express();
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: false
}));
- Once the session middleware is set up, you can access and modify session data in your route handlers:
app.get('/login', (req, res) => {
// Set session data
req.session.user =
{ id: 1, username: 'example' };
res.send('Logged in');
});
app.get('/profile', (req, res) => {
// Access session data
const user = req.session.user;
res.send(`Welcome ${user.username}`);
});
app.get('/logout',
(req, res) => {
// Destroy session
req.session.destroy((err) => {
if (err) {
console.error(err);
res.status(500).send('Error logging out');
} else {
res.send('Logged out');
}
});
});
- By default, sessions are stored in memory, which is not suitable for production use. You can use session stores like
connect-mongo
or connect-redis
to store sessions in MongoDB or Redis, respectively:
const session = require('express-session');
const MongoStore = require('connect-mongo')(session);
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: false,
store: new MongoStore(
{
url: 'mongodb://localhost/session-store'
}
)
}));
- By default,
express-session
uses cookies to store session IDs. Ensure that your application properly handles session cookies and sets appropriate security options, such as secure
, httpOnly
, and sameSite
, to prevent common security vulnerabilities like session hijacking and XSS attacks:
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: false,
cookie: {
secure: true,
// Enable only for HTTPS
httpOnly: true,
// Prevent client-side access to cookies
sameSite: 'strict'
// Mitigate CSRF attacks
}
}));
By following these steps, you can effectively handle sessions in your ExpressJS application, allowing you to maintain user state and provide personalized experiences for your users.
Example: Below is the example to handle session in ExpressJS.
Javascript
const express = require( 'express' );
const session = require( 'express-session' );
const app = express();
app.use(session({
secret: 'mySecretKey' ,
resave: false ,
saveUninitialized: false
}));
app.use((req, res, next) => {
console.log('Session: ', req.session);
next();
});
// Route to set session data
app.get(' /set-session ', (req, res) => {
req.session.user = { id: 1, username: ' GfG User ' };
res.send(' Session data set ');
});
// Route to get session data
app.get(' /get-session ', (req, res) => {
if (req.session.user) {
res.send(' Session data: '
+ JSON.stringify(req.session.user));
} else {
res.send(' No session data found ');
}
});
// Route to destroy session
app.get(' /destroy-session ', (req, res) => {
req.session.destroy((err) => {
if (err) {
console.error(' Error destroying session: ', err);
res.send(' Error destroying session ');
} else {
res.send(' Session destroyed');
}
});
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`);
});
|
Output:
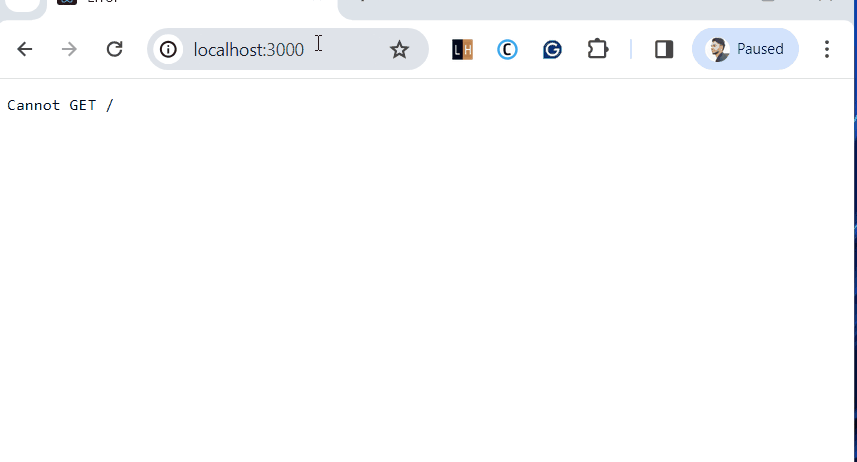
Output
Share your thoughts in the comments
Please Login to comment...