Do you want to improve your command on C++ language? Explore our vast library of C++ exercise questions, which are specifically designed for beginners as well as for advanced programmers. We provide a large selection of coding exercises that cover every important topic, including classes, objects, arrays, matrices, and pointers. Master C++ with our practical approach and practice C++ exercises online.
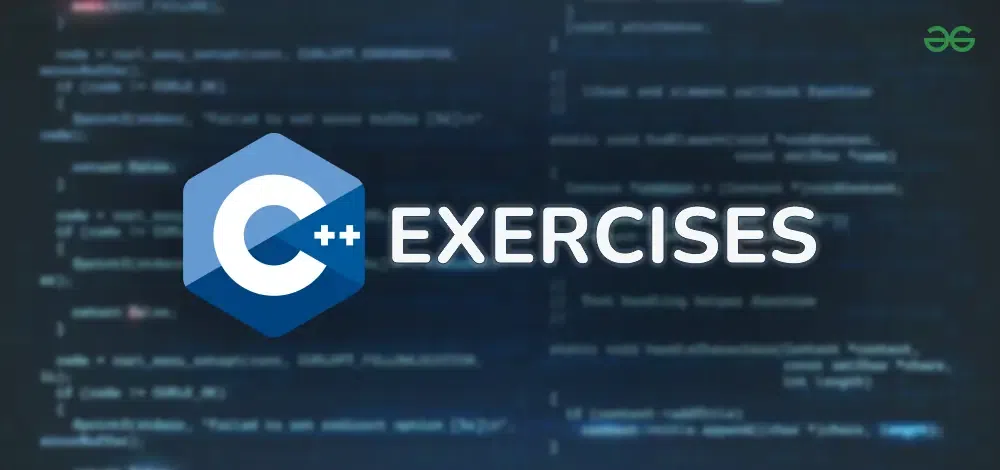
C++ Exercise Problems
There are over 50+ C Exercise questions for you to practice along with the solutions to every question for a better understanding. You can solve these questions online in GeeksforGeeks IDE.
Q1. Write a Program to Print “Hello World” in the Console Screen.
Write a simple program that prints the words “Hello World” on the console.
For Example,
Output: Hello World
Q2. Write a Program to Read and Print Number Input From the User.
In this problem, you have to create a simple program that reads a number that is entered by the user and prints it on the console screen.
For Example,
Input: Enter any Number: 25
Output: Entered Number: 25
Q3. Write a Program to Print the ASCII Value of a Character.
In C++, each character has some ASCII value associated with it. In this problem, we have to print the ASCII value of the character in the console.
For Example,
Character = A
Output: ASCII Value of 'A' = 65
Q4. Write a Program to Swap Two Numbers.
You have to create a program that swaps the value of two number variables. It means that the value of the first variable will be stored in the second variable and the value of the second variable should be stored in the first variable.
For Example,
// Variables initally
a = 10, b = 250
// After execution
Output: a = 250, b = 10
Q5. Write a Program to Find the Size of int, float, double, and char.
In C++, each datatype requires some memory to store data. In this program, you have to print the required memory (i.e. size in bytes) for int, float, double, and char data types on the console.
For Example,
Output
size of int: 10 bytes
size of float: 20 bytes
size of double: 30 bytes
size of char: 40 bytes
Q6. Write a Program to Find Compound Interest.
In this problem, you have to write a program that calculates and prints the compound interest for the given Principle, Rate of Interest, and Time.
For Example,
Principle = 25000
Rate = 12%
Time = 5 Years
Output: Compound Interest = 19058.54
Q7. Write a Program to Check Even or Odd Integers.
In this problem, we have to simply check whether the given integer is odd or even and print the output on the console.
For Example,
Number = 28
Output: 28 is Even Number.
Q8. Write a Program to Find the Largest Among 3 Numbers.
In this problem, you are given 3 numbers, and you have to find out which one is the largest.
For Example,
Given Numbers: a = 10, b = 21 and c = 4
Output: b is the largest.
Q9. Write a Program to Check if a Given Year Is a Leap Year.
In this problem, you have to write a program that takes a year as an input, and then checks whether it is a leap year or not.
For Example,
Input: 2020
Output: 2020 is a Leap Year
Q10. Write a Program to Check Whether a Number Is Prime or Not.
The number can be prime or non-prime based on the number of its factors. In this program, we have to check whether the given number is prime or not and print the result on the console screen.
For Example,
Number to Check = 29
Output: 29 is a prime number.
Q11. Write a Program to Check Whether a Number Is a Palindrome or Not.
A palindrome number is a number that is equal to itself even after reversing its digits. In this program, we have to check for palindrome numbers.
For Example,
Number to Check = 1231
Output: 1231 is not a palindrome number.
Q12. Write a Program to Make a Simple Calculator.
In this problem, you have to make a program that can perform addition, subtraction, multiplication, and division on two numbers entered by the user. The type of arithmetic operation can also be selected by the user.
For Example,
Input:
Enter the Number: 10 25
Enter a for addition,
s for substraction,
m for multiplication,
d for division.
{{if m is entered}}
Output: 250
Q13. Write a Program to Reverse a Sentence Using Recursion.
In this program, you have to simply reverse the sentence stored as a string.
For Example,
Sentence = "Quick brown fox";
Output: xof nworb kciuQ
Q14. Write a Program for Fibonacci Numbers Using Recursion.
The Fibonacci Series is a mathematical sequence in which the next number is the sum of the last two numbers in the sequence. In this program, you will have to print the Nth number in the sequence using recursion.
For Example,
N = 12
Output: 144
Q15. Write a Program to Swap Two Numbers Using a Function.
In this program, you have to swap the values of two variables using another function.
For Example,
// in main function
a = 10, b = 22
Output: a = 22, b = 10
Q16. Write a Program to Check if Two Arrays Are Equal or Not.
An array is said to be equal if the elements at the given index are equal in both arrays. In this program, you have to take two arrays, and then check whether the two arrays are equal.
For Example,
arr1[] = {5, 8, 3}
arr2[] = {5, 8, 11, 2}
Output: arr1[] and arr2[] are not equal.
Q17. Write a Program to Calculate the Average of All the Elements Present in an Array.
In this problem, you have to calculate the average of all the elements present in the array and print it on the console screen.
For Example,
arr[] = {10, 22, 45, 11}
Output: Average = 24.5
Q18. Write a Program to Find the Maximum and Minimum in an Array.
In this problem, you have to find both maximum(largest) and minimum(smallest) numbers in a numerical array.
For Example,
arr[] = {10, 12, 45, 48, 22, 18}
Output:
Maximum = 48
Minimum = 10
Q19. Write a Program to Search an Element in an Array (Linear Search).
In this program, you have to search for the given element in an array. If the element is found, you will print the index of the element. The array is unsorted.
For Example,
arr[] = {10, 11, 7, 8, 2, 9};
Element to be Searched: 19
Output: 19 not found in the arr[]
Q20. Write a Program to Print the Array After It Is Right Rotated K Times.
Array Rotation means shifting the elements n positions to left or right. In this problem, you will have to rotate the array in the right direction k number of times.
For Example,
arr[] = {10, 11, 7, 33, 5, 1};
K = 3
Output: Array after rotation: {33, 5, 1, 10, 11, 7}
Q21. Write a Program to Compute the Sum of Diagonals of a Matrix.
In this problem, you have to calculate the Sum of both the diagonal elements of a matrix. Matrix are generally represented as 2D arrays.
For Example,
matrix = 1 2 3
4 5 6
7 8 9
Output:
Principal Diagonal = 16
Secondary Diagonal = 15
Q22. Write a Program to Rotate the Elements of a Matrix.
We can rotate the matrix in two ways: clockwise and anticlockwise. In this problem, you have to rotate the elements of the matrix in the clockwise direction for K number of times.
For Example,
matrix = 1 2 3
4 5 6
7 8 9
K = 2
Output:
Matrix = 7 4 1
8 5 2
9 6 3
Q23. Write a Program to Find the Length of a String.
The length of the string is the number of characters in a string except the NULL character. Write a simple program to find the length of a string and print it on the console.
For Example,
str = "GeeksforGeeks"
Output: Length of str = 13
Q24. Write a Program to Compare Two Strings.
In this problem, you have to write a program to compare two strings to check whether they are the same or not.
For Examples,
str1 = "Geeks"
str2 = "geeks"
Output: str1 and str2 are not equal.
Q25. Write a Program to Check if the String Is Palindrome.
Similar to a palindrome number, a palindrome string is a string that is equal to its reverse. You have to write a program to check whether the given string is a palindrome string or not.
For Example,
str = "naman"
Output: str is a palindrome string.
Q26. Write a Program to Add 2 Binary Strings.
In some cases, numbers can also be represented as strings. In this problem, you have a string representation of a binary number and you have to convert this binary number string to the numerical integer form.
For Example,
str = "101011";
Output: Binary Number = 101011
Q27. Write a Program to Convert String to int.
Like the above problem, you have to create a program to convert the number in the string form to the integer form. But in this program, instead of binary, you have to work with a decimal number system.
For Example,
str = "69420"
Output: Number = 69420
Q28. Write a Program to Split a String into a Number of Sub-Strings.
In this problem, you will write a program to split the given string into substrings based on some delimiter. It is also called tokenization.
For Example,
str = "Welcome Geeks to the GeeksforGeeks portal."
Output:
Welcome
Geeks
to
the
GeeksforGeeks
portal.
Q29. Write a Program to Print a Simple Full Pyramid Pattern.
In this problem, you have to print the simple pyramid pattern shown below:
*
* *
* * *
* * * *
* * * * *
This pattern will vary with the number of rows specified.
Q30. Write a Program That Receives a Number and Prints It Out in Large Size.
In this problem, you will write a program to print the given numbers in large form as shown below:
Input: 12
Output:
# #####
## #
# #
# #####
# #
# #
##### #####
Q31. Write a Program to Print Pascal’s Triangle.
Pascal Triangle is a pattern in which binomial coefficients are used as the elements. In this problem, you have to write the program to print the Pascal triangle in the console.
For Example,
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Q32. Write a Program for Binary to Decimal Conversion.
In this problem, you will have to write a program that converts the number from the binary system to the decimal number system.
For Example,
Input: 101011
Output: 43
Q33. Write a Program for Decimal to Octal Conversion.
Similar to the above problem, in this problem, a number in the decimal system is to be converted to the octal conversion using a C++ program.
For Example,
Input: 100
Output: 144
Q34. Write a Program to Sort an Array(Bubble Sort).
Sorting algorithms are used to sort the data collection into some defined order. Write a program that sorts the array in increasing order using the bubble sort sorting algorithm.
For Example,
arr[] = {1, 85, 41, 23, 11}
Output: arr[] = {1, 11, 23, 41, 85}
Q35. Write a Program to Search an Element in an Array (Binary Search).
Binary Search is a search algorithm similar to linear search. In this problem, you have to search for a given element K in and print its index. If the element is not found, then print “K is not found”. You have to use binary search.
For Example,
arr[] = {1, 2, 3, 8, 9, 11}
K = 9
Output: 9 is at index 4
Q36. Write a Program of Merge Sort.
Merge Sort is an efficient sorting algorithm that can be used to sort the collection of values. In this problem, implement merge sort algorithm to sort the array in increasing order.
For Example,
arr[] = {1, 85, 41, 23, 11}
Output: arr[] = {1, 11, 23, 41, 85}
Q37. Write a Program to Sort a String.
Write a program to sort the array or vector of string in lexicographically ascending order. The sorting of strings is similar the numeric sorting but with some minor differences.
For Example,
Input: arr = {"Abhishek", "Ankur", "Satyam", "Ankit", "Yuvraj"}
Output: arr = {"Abhishek", "Ankit", "Ankur", "Satyam", "Yuvraj"}
Q38. Write a Program to Store Information of a Student in a Structure.
In this problem, you have to create a program to store the information of the individual student i.e. name, roll number, subjects enrolled, marks in each subject, and CGPA in a structure.
For Example,
Student Name: Aditya Arpan
Roll Number: 69420
Subjects Enrolled: {"DSA", "CN", "CO", "TOC", "DBMS"}
Marks: {92, 85, 89, 93, 99}
CGPA: 9.8
Q39. Write a Program to Add Two Complex Numbers.
In this problem, you have to create a complex number data type and create a method to add the two complex numbers.
For Example,
num1 = 2 + 3i
num2 = 5 + 11i
Output: 7 + 14i
Q40. Write a Program to Create an Interface.
Interfaces are not part of C++ but their behavior can be imitated. In this problem, you have to create a class that works in a similar way as the interface works in Java.
For Example,
class interfaceClass { // declartion }
class D: public interfaceClass { // own definition }
class X: public interfaceClass { // own definition }
Q41. Write a Program to Create a New File.
In this program, you have to create a new file using a C++ program. Also, check if the file is created. If failed, print “File Creation Failure”, otherwise print “File Creation Successful”.
For Example,
/* before execution */
/some_parent_directory/{empty directory}
//*after execution */
/some_parent_directory/new_file.txt
Output: File Creation Successful.
Q42. Write a Program to Append the Content of One Text File to Another.
In the problem, you have to write a program that copies the content of one file to another file using C++ such that it does not replace the existing content in the second file. After copying, print the contents of the second file.
file1.txt
This content will be copied.
file2.txt
This content will remain even after copying.
// After execution
Output
This content will remain even after copying.This content will be copied.
Q43. Write a Program to Get the List of Files in a Directory.
In this problem, you will have to print the list of files that are present in some directory using C++ language.
For Example, consider the following directory.
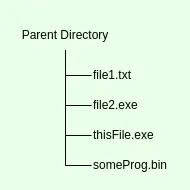
Output
Files in Parent Directory:
file1.txt
file2.exe
thisFile.exe
someProg.bin
Q44. Write a Program for Handling the Divide by Zero Exception.
Exception handling provides a defined way to deal with errors. In this problem, you will create a C++ program to handle one of the most commonly encountered errors which occurs when some numeric value is divided by zero.
For Example,
// somewhere in the code
num = 10 / 0
Output:
Math error: Attempted to divide by Zero
Q45. Write a Program to Copy a Vector Using STL.
There are many ways in C++ to copy the elements of a vector to another vector. In this problem, you have to copy the vector using the std::copy function of the STL.
For Example,
vec1 = {1, 5, 7 ,3 }
vec2 = {}
Output
vec2 = {1, 5, 7, 3}
Q46. Write a Program to Find the Maximum and Minimum Elements in a Set.
In this problem, you have to write a program to find the maximum and minimum elements in an STL Set container and print them in the console.
For Example,
set_obj = {1, 9, 3, 11, 24, 45};
Output:
Minimum = 1
Maximum = 45
Q47. Write a Program to Find Permutations of a Given String Using STL.
In this problem, you have to write a program to find and print all the possible permutations of a string using the methods provided in C++ STL.
For Example,
Input: str = "ABC"
Output:
"ABC",
"ACB",
"BAC",
"BCA",
"CBA",
"CAB"
Q48. Write a Program for Implementing Stack Using Class Templates.
In this problem, you have to create your own stack data structure that will be able to work for different data types. This stack should come with functions to perform the basic operations such as: push(), pop(), top(), and empty().
For Example, the following operations should be valid.
My_stack v;
v.push(89);
v.push(2);
cout << v.top();
Output
2
Q49. Write a Program to Create a Custom Vector Class.
In this problem, you have to create your own vector container that will be able to work for different data types. It will work as a dynamic array with some basic functions such push_back(), pop_back() and size().
For Example, the following operations should be valid.
My_vector v;
v.push_back(12);
v.push_back(22);
cout << v[1];
Output
22
Q50. Write a Program to Build a Custom Map Using Header File.
In this problem, you will create a header file that defines a container similar to std::map in C++ STL. Then you will use this header file in another C++ program. The container should contain the following functions:
- insert()
- find()
- update()
- accessing any key
- erase()
- count()
- size()
- empty()
- clear()
Q51. Write a Program for Finding the Middle Element of a Given Linked List.
In this program, you have to first implement a linked list and insert some elements. After that, you will have to find the middle element in the linked list.
For Example,
linkedList: 1 -> 5 -> 11 -> 3 -> 7
Output: Middle Element: 7
Q52. Write a Program to Print the Current Day, Date, and Time.
In this problem, you have to write the program that prints the current day, date, and time on the console in the format that is shown below.
Output
Thu Dec 28 12:25:08 2023
Q53. Write a Program to Print a Calendar for a Given Year.
In this program, the user will enter a year and you will have to print the calendar for the given year in a grid-like format with days and dates.
For Example, the month of February 2016 looks like this:
------------February-------------
Sun Mon Tue Wed Thu Fri Sat
1 2 3 4 5 6
7 8 9 10 11 12 13
14 15 16 17 18 19 20
21 22 23 24 25 26 27
28 29
Q54. Write a Program to Generate Random Double Numbers.
C++ provides different methods to generate some random numbers. In this problem, you have to use one of these methods to generate 10 random numbers of type double.
For Example,
10.125
120.44
211534.4123
123216484.11111
Conclusion
We hope you found these C++ exercises useful in advancing your knowledge of C++ programming language. Our C++ exercise sheet is designed to help you at every stage of the learning process.
Solving C++ exercises can help you practice different C++ concepts in codes, and understand their workings. Each problem you solve brings you one step closer to your goal of mastering C++.
Frequently Asked Questions (FAQs)
Q1. What topics should I cover in C++ exercises to prepare for interviews?
Answer:
Some of the most common topics of C++ programming that are asked in interviews are:
- Data Structures
- Algorithms
- Object-Oriented Programming,
- Pointers
- Dynamic Memory Management
Q2. Is it important to practice C++ exercises even if I know other programming languages?
Answer:
Yes, it is important to practice C++ exercises as it has unique features like memory management and object-oriented concepts that might differ from other languages.
Q3. What is C++ used in today?
Answer:
C++ is used in many fields and industries like:
- Gaming
- Operating Systems
- Embedded Systems
- Automotive Systems
- Telecommunications
- Web Browsers
Share your thoughts in the comments
Please Login to comment...