The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
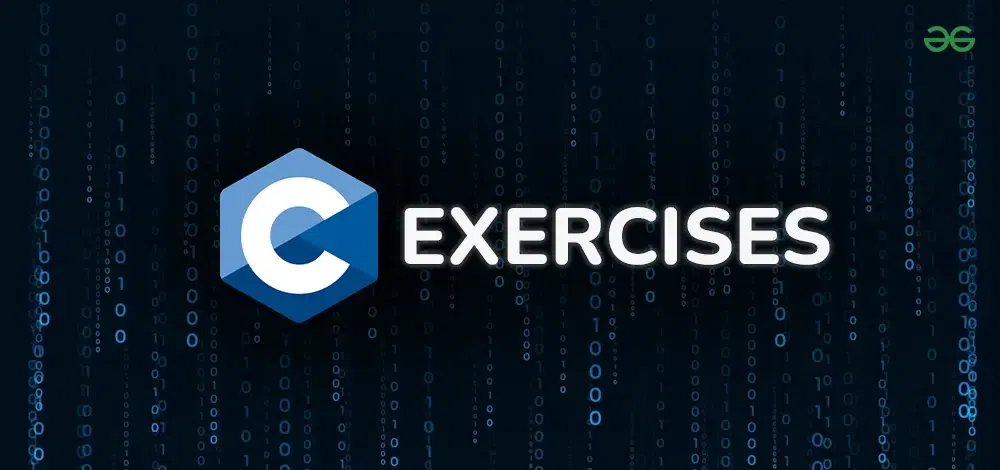
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Output: Hello World!
Q2: Write a Program to find the Sum of two numbers entered by the user.
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
For Example,
Input: Enter two numbers A and B : 5 2
Output: Sum of A and B is: 7
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
For Example,
Output: Size of int = 4
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
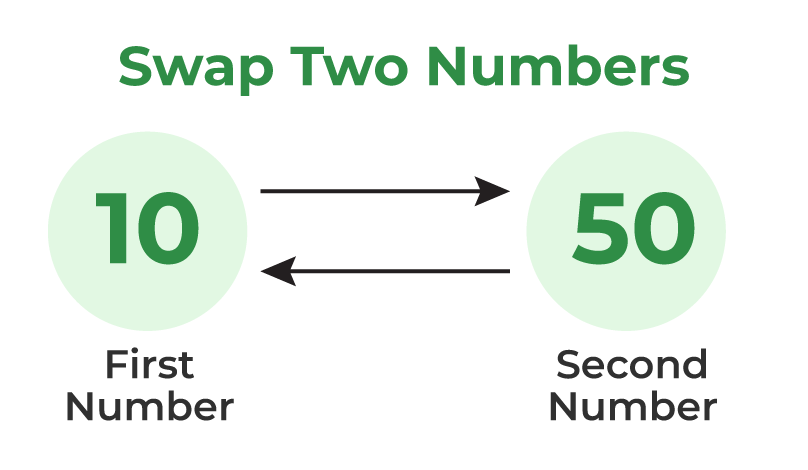
Swap two numbers
For Example,
Input: Enter Value of x: 5
Enter Value of y: 10
Output: After Swapping: x = 10, y = 5
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
For Example,
Input: Enter Principal (amount): 1200
Enter Time: 2
Enter Rate: 5.4
Output: Compound Interest is: 133.099243
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
For Example,
Input: Enter a Number: 2
Output: even
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
For Example,
Input: Enter a Number1: 5
Enter a Number2: 50
Enter a Number3: 10
Output: Largest number is: 50
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
For Example,
Input: Enter an operator (+, -, *, /): +
Enter operand 1: 7
Enter operand 2: 8
Output: 15.0
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
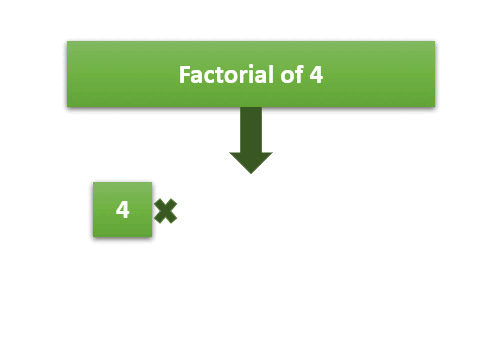
Factorial
For Example,
Input: Enter a number: 5
Output: Factorial of 5 is: 120
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
For Example,
Input: Enter a binary number: 10101001
Output: Decimal number is: 169
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
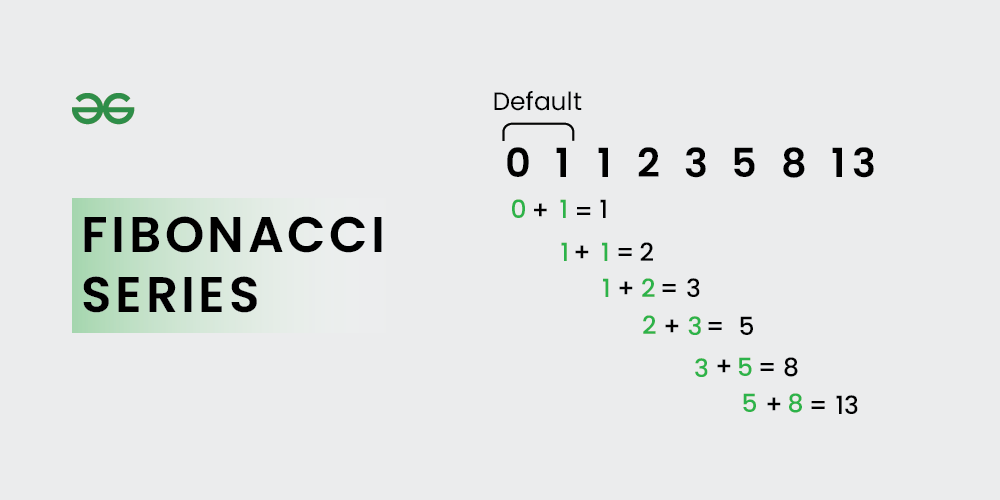
Fibonacci Series
For Example,
Input: Enter value of n:
Output: 0 1 1 2 3 5 8 13 21
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
For Example,
Input: Enter value of n: 10
Output: 55
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
For Example,
Input: arr[] = {4, 7, 2, 1, 9}
Output:Â Minimum element is: 1
Maximum element is: 9
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
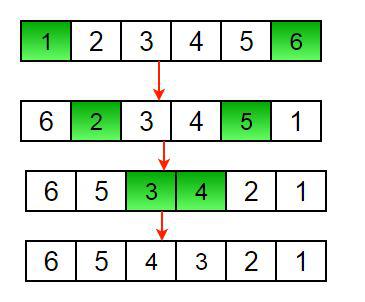
Reverse an array
For Example,
Input: arr[] = {1, 2, 3, 4, 5 ,6} , n = 6
Output:Â Reversed array is: 6 5 4 3 2 1
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.Â
For Example,
Input: arr[] = {1, 2, 3, 4, 5}, D = 2
Output:Â After rotation: 3 4 5 1 2
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
For Example,
Input: arr[] = {1, 2, 2, 3, 4, 4, 4, 5, 5}
Output:Â array after removal of duplicates: 1 2 3 4 5
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array‘.
For Example,
Input: arr[] = { 2, 3, 4, 10, 40 }
target: 10
Output: Element is present at index 3
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
For Example,
Input : 1->2->3->4->NULL
Output : Reversed Linked list: 4->3->2->1->NULL
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
For Example,
Output : Elements of the array are: 1, 2, 3, 4, 5,
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
For Example,
Input : matrix A:
{ {1, 1, 1, 1},
{2, 2, 2, 2},
{3, 3, 3, 3},
{4, 4, 4, 4} }
Output : Result matrix is :
1 2 3 4
1 2 3 4
1 2 3 4
1 2 3 4
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
For Example,
Input: str1 = "hello", str2 = "world"
Output: helloworld
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
For Example,
Input: Enter str: "abbba"
Output: abbba is a palindrome
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
For Example,
Input: Enter str: Geeks for Geeks
Output: G f G
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.Â
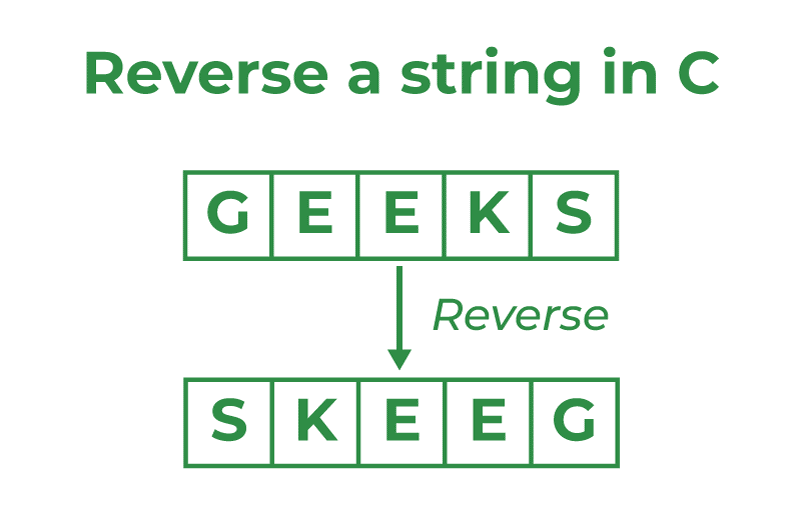
reverse a string
For Example,
Input: Enter str: Geeks
Output: Reversed string is: skeeG
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
For Example,
Input: Enter number of rows: 5
Output:
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
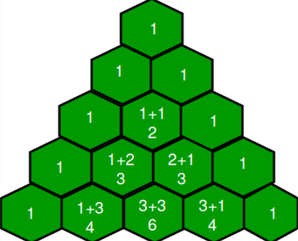
Pascal’s Triangle
For Example,
Input: Enter number of rows: 5
Output:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
For Example,
Input: arr[] = {12, 11, 13, 5, 6} , N = 5
Output: 5 6 11 12 13
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
For Example,
Input: arr[] = { 19, 17, 15, 12, 16, 18, 4, 11, 13 } , N = 9
Output: 4 11 12 13 15 16 17 18 19
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.Â
For Example,
Input: arr[] =
Output: After sorting: clanguage , geeksforgeeks , geeksquiz
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
For Example,
Input: Enter the filename you want to copy: a.txt
Output: File after copying content: b.txt
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
For Example,
Output: Student Records:
Name = Student1
Roll Number = 1
Age = 12
Total Marks = 78.50
Name = Student2
Roll Number = 5
Age = 10
Total Marks = 56.84
Conclusion
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. What are some common mistakes to avoid while doing C programming exercises?
Answer:
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Answer:
Best practices for beginners starting with C programming exercises:
- Start with easy codes
- Practice consistently
- Be creative
- Think before you code
- Learn from mistakes
- Repeat!
Q3. How do I debug common errors in C programming exercises?
Answer:
You can use the following methods to debug a code in C programming exercises
- Read the error message carefully
- Read code line by line
- Try isolating the error code
- Look for Missing elements, loops, pointers, etc
- Check error online
Share your thoughts in the comments
Please Login to comment...