C++ program to create a file
Last Updated :
28 Sep, 2018
Problem Statement: Write a C++ program to create a file using file handling and check whether the file is created successfully or not. If a file is created successfully then it should print “File Created Successfully” otherwise should print some error message.
Approach: Declare a stream class file and open that text file in writing mode. If the file is not present then it creates a new text file. Now check if the file does not exist or not created then return false otherwise return true.
Below is the program to create a file:
#include <bits/stdc++.h>
using namespace std;
int main()
{
fstream file;
file.open( "Gfg.txt" ,ios::out);
if (!file)
{
cout<< "Error in creating file!!!" ;
return 0;
}
cout<< "File created successfully." ;
file.close();
return 0;
}
|
Output:
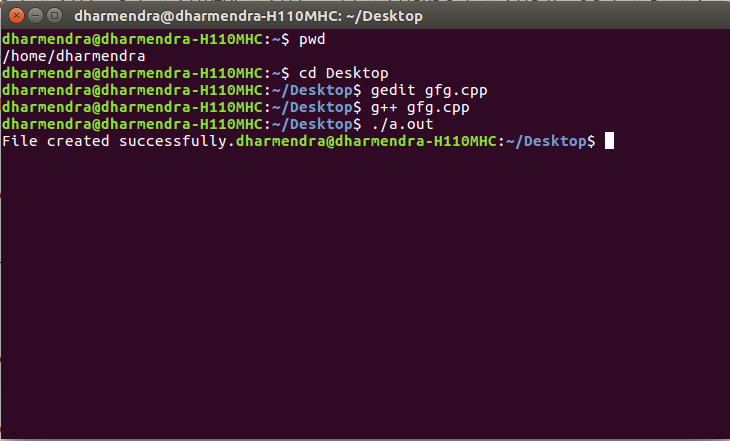
Share your thoughts in the comments
Please Login to comment...