TCS Coding Practice Question | Average of 2 Numbers
Last Updated :
16 Feb, 2023
Given two numbers, the task is to find the average of two numbers using Command Line Arguments. Examples:
Input: n1 = 10, n2 = 20
Output: 15
Input: n1 = 100, n2 = 200
Output: 150
Approach:
- Since the numbers are entered as Command line Arguments, there is no need for a dedicated input line
- Extract the input numbers from the command line argument
- This extracted numbers will be in String type.
- Convert these numbers into integer type and store it in variables, say num1 and num2
- Find the average of the numbers using the formula ((num1 + num2)/2)
- Print or return the average
Program:
C
#include <stdio.h>
#include <stdlib.h> /* atoi */
int average( int a, int b)
{
return (a + b) / 2;
}
int main( int argc, char * argv[])
{
int num1, num2;
if (argc == 1)
printf ( "No command line arguments found.\n" );
else {
num1 = atoi (argv[1]);
num2 = atoi (argv[2]);
printf ( "%d\n" , average(num1, num2));
}
return 0;
}
|
Java
class GFG {
static int average( int a, int b)
{
return (a + b) / 2 ;
}
public static void main(String[] args)
{
if (args.length > 0 ) {
int num1 = Integer.parseInt(args[ 0 ]);
int num2 = Integer.parseInt(args[ 1 ]);
int res = average(num1, num2);
System.out.println(res);
}
else
System.out.println( "No command line "
+ "arguments found." );
}
}
|
Python
def average(n1,n2):
return ( int ((n1 + n2) / 2 ))
n1,n2 = input ().split()
n1 = int (n1)
n2 = int (n2)
result = (average((n1),(n2)))
print (result)
|
Output:
- In C:
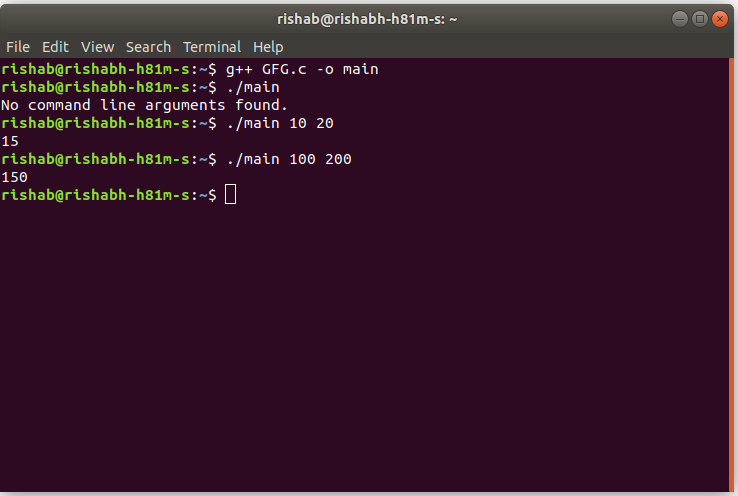
- In Java:
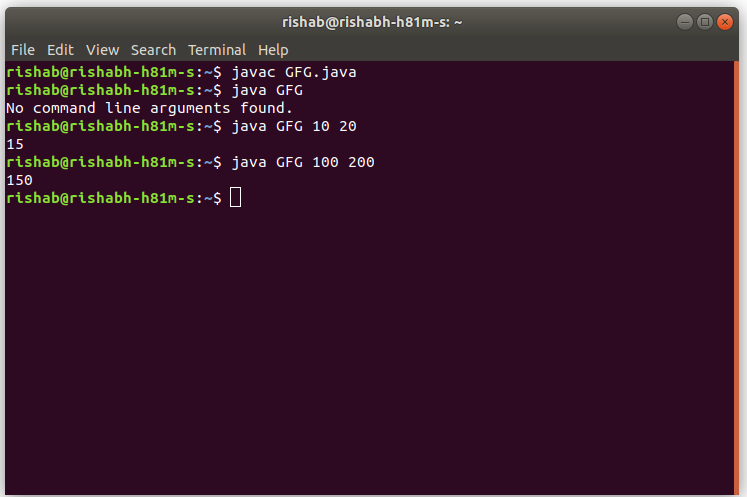
- in Python
Share your thoughts in the comments
Please Login to comment...