What is Array in JavaScript?
JavaScript Array is a data structure that allows you to store and organize multiple values within a single variable. It is a versatile and dynamic object. It can hold various data types, including numbers, strings, objects, and even other arrays. Arrays in JavaScript are zero-indexed i.e. the first element is accessed with an index 0, the second element with an index of 1, and so forth.
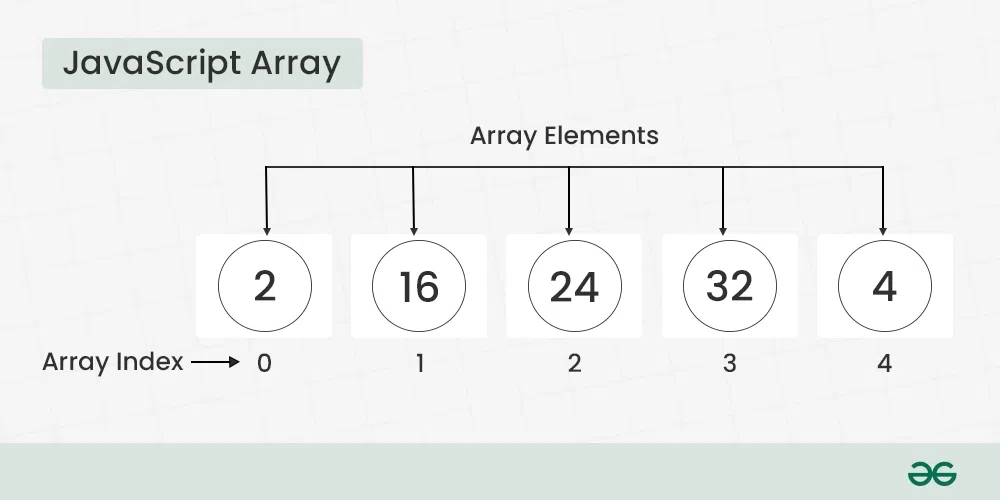
JavaScript Array
You can create JavaScript Arrays using the Array constructor or using the shorthand array literal syntax, which employs square brackets. Arrays can dynamically grow or shrink in size as elements are added or removed.
Basic Terminologies of JavaScript Array
- Array: A data structure in JavaScript that allows you to store multiple values in a single variable.
- Array Element: Each value within an array is called an element. Elements are accessed by their index.
- Array Index: A numeric representation that indicates the position of an element in the array. JavaScript arrays are zero-indexed, meaning the first element is at index 0.
- Array Length: The number of elements in an array. It can be retrieved using the length property.
Declaration of an Array
There are basically two ways to declare an array i.e. Array Literal and Array Constructor.
1. Creating an Array using Array Literal
Creating an array using array literal involves using square brackets [] to define and initialize the array. This method is concise and widely preferred for its simplicity.
Syntax:
let arrayName = [value1, value2, ...];
Example:
Javascript
let names = [];
console.log(names);
let courses = [ "HTML" , "CSS" , "Javascript" , "React" ];
console.log(courses);
|
Output
[]
[ 'HTML', 'CSS', 'Javascript', 'React' ]
2. Creating an Array using Array Constructor (JavaScript new Keyword)
The “Array Constructor” refers to a method of creating arrays by invoking the Array constructor function. This approach allows for dynamic initialization and can be used to create arrays with a specified length or elements.
Syntax:
let arrayName = new Array();
Example:
javascript
let names = new Array();
console.log(names);
let courses = new Array( "HTML" , "CSS" , "Javascript" , "React" );
console.log(courses);
let arr = new Array(3);
arr[0] = 10;
arr[1] = 20;
arr[2] = 30;
console.log(arr);
|
Output
[]
[ 'HTML', 'CSS', 'Javascript', 'React' ]
[ 10, 20, 30 ]
Note: Both the above methods do exactly the same. Use the array literal method for efficiency, readability, and speed.
Basic Operations on JavaScript Arrays
1. Accessing Elements of an Array
Any element in the array can be accessed using the index number. The index in the arrays starts with 0.
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" ];
console.log(courses[0]);
console.log(courses[1]);
console.log(courses[2]);
console.log(courses[3]);
|
Output
HTML
CSS
Javascript
React
2. Accessing the First Element of an Array
The array indexing starts from 0, so we can access first element of array using the index number.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
let firstItem = courses[0];
console.log( "First Item: " , firstItem);
|
3. Accessing the Last Element of an Array
We can access the last array element using [array.length – 1] index number.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
let lastItem = courses[courses.length - 1];
console.log( "First Item: " , lastItem);
|
4. Modifying the Array Elements
Elements in an array can be modified by assigning a new value to their corresponding index.
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" ];
console.log(courses);
courses[1]= "Bootstrap" ;
console.log(courses);
|
Output
[ 'HTML', 'CSS', 'Javascript', 'React' ]
[ 'HTML', 'Bootstrap', 'Javascript', 'React' ]
5. Adding Elements to the Array
Elements can be added to the array using methods like push() and unshift().
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" ];
courses.push( "Node.js" );
courses.unshift( "Web Development" );
console.log(courses);
|
Output
[ 'Web Development', 'HTML', 'CSS', 'Javascript', 'React', 'Node.js' ]
6. Removing Elements from an Array
Remove elements using methods like pop(), shift(), or splice().
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" , "Node.js" ];
console.log( "Original Array: " + courses);
let lastElement = courses.pop();
console.log( "After Removed the last elements: " + courses);
let firstElement = courses.shift();
console.log( "After Removed the First elements: " + courses);
courses.splice(1, 2);
console.log( "After Removed 2 elements starting from index 1: " + courses);
|
Output
Original Array: HTML,CSS,Javascript,React,Node.js
After Removed the last elements: HTML,CSS,Javascript,React
After Removed the First elements: CSS,Javascript,React
After Removed 2 elements starting from index 1: CSS
7. Array Length
Get the length of an array using the length property.
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" , "Node.js" ];
let len = courses.length;
console.log( "Array Length: " + len);
|
8. Increase and Decrease the Array Length
We can increase and decrease the array length using the JavaScript length property.
Javascript
let courses = [ "HTML" , "CSS" , "Javascript" , "React" , "Node.js" ];
courses.length = 7;
console.log( "Array After Increase the Length: " , courses);
courses.length = 2;
console.log( "Array After Decrease the Length: " , courses)
|
Output
Array After Increase the Length: [ 'HTML', 'CSS', 'Javascript', 'React', 'Node.js', <2 empty items> ]
Array After Decrease the Length: [ 'HTML', 'CSS' ]
9. Iterating Through Array Elements
We can iterate array and access array elements using for and forEach loop.
Example: It is the example of for loop.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
for (let i = 0; i < courses.length; i++) {
console.log(courses[i])
}
|
Output
HTML
CSS
JavaScript
React
Example: It is the example of Array.forEach() loop.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
courses.forEach( function myfunc(elements) {
console.log(elements);
});
|
Output
HTML
CSS
JavaScript
React
10. Array Concatenation
Combine two or more arrays using the concat() method. Ir returns new array conaining joined arrays elements.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
let otherCourses = [ "Node.js" , "Expess.js" ];
let concateArray = courses.concat(otherCourses);
console.log( "Concatenated Array: " , concateArray);
|
Output
Concatenated Array: [ 'HTML', 'CSS', 'JavaScript', 'React', 'Node.js', 'Expess.js' ]
11. Conversion of an Array to String
We have a builtin method toString() to converts an array to a string.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
console.log(courses.toString());
|
Output
HTML,CSS,JavaScript,React
12. Check the Type of an Arrays
The JavaScript typeof operator is used ot check the type of an array. It returns “object” for arrays.
Javascript
let courses = [ "HTML" , "CSS" , "JavaScript" , "React" ];
console.log( typeof courses);
|
Difference Between JavaScript Arrays and Objects
- JavaScript arrays use indexes as numbers.
- objects use indexes as names..
When to use JavaScript Arrays and Objects?
- Arrays are used when we want element names to be numeric.
- Objects are used when we want element names to be strings.
Recognizing a JavaScript Array
There are two methods by which we can recognize a JavaScript array:
Below is an example showing both approaches:
Javascript
const courses = [ "HTML" , "CSS" , "Javascript" ];
console.log( "Using Array.isArray() method: " , Array.isArray(courses))
console.log( "Using instanceof method: " , courses instanceof Array)
|
Output
Using Array.isArray() method: true
Using instanceof method: true
Note: A common error is faced while writing the arrays:
const numbers = [5]
// and
const numbers = new Array(5)
Javascript
const numbers = [5]
console.log(numbers)
|
The above two statements are not the same.
Output: This statement creates an array with an element ” [5] “.
[5]
Javascript
const numbers = new Array(5)
console.log(numbers)
|
Output
[ <5 empty items> ]
JavaScript Array Complete Reference
We have a complete list of Javascript Array, to check those please go through this JavaScript Array Reference article. It contains detailed description and examples of all Array Properties and Methods.
JavaScript Array Examples
JavaScript Array examples contains list of questions that are majorily asked in interview. Please check this article JavaScript Array Examples for more detail.
JavaScript CheatSheet
We have a Cheat Sheet on Javascript where we have covered all the important topics of Javascript to check those please go through Javascript Cheat Sheet-A Basic guide to JavaScript.
Share your thoughts in the comments
Please Login to comment...