React Events Reference
Last Updated :
05 Dec, 2023
When the user interacts with the web application events are fired. That event can be a mouse click, a keypress, or something rare like connecting the battery with a charger. From the developer side, we need to ‘listen’ to such events and then make our application respond accordingly. This is called event handling which provides a dynamic interface to a webpage.
Syntax:
onEvent={function}
Now we will look at certain rules to keep in mind while creating events in React.
- camelCase Convention: Instead of using lowercase we use camelCase while giving names of the react events. That simply means we write ‘onClick’ instead of ‘onclick’.
- Pass the event as a function: In React we pass a function enclosed by curly brackets as the event listener or event handler, unlike HTML where we pass the event handler or event listener as a string.
- Prevent the default event: Just returning false inside the JSX element does not prevent the default behavior in react. Instead, we have to call the ‘preventDefault’ method directly inside the event handler function.
Example : In this example we implemented an onClick Event in it.
Javascript
import "./App.css"
const App = () => {
const function1 = (event) => {
alert( "Click Event Fired" )
}
return (
<div className= "App" >
<h1>GeeksforGeeks</h1>
<button onClick={function1}>
Click Me!
</button>
</div>
);
}
export default App;
|
CSS
.App {
min-width : 30 vw;
margin : auto ;
text-align : center ;
color : green ;
font-size : 23px ;
}
button {
background-color : lightgray;
height : 50px ;
width : 150px ;
margin : auto ;
border-radius: 6px ;
cursor : pointer ;
}
|
Output:
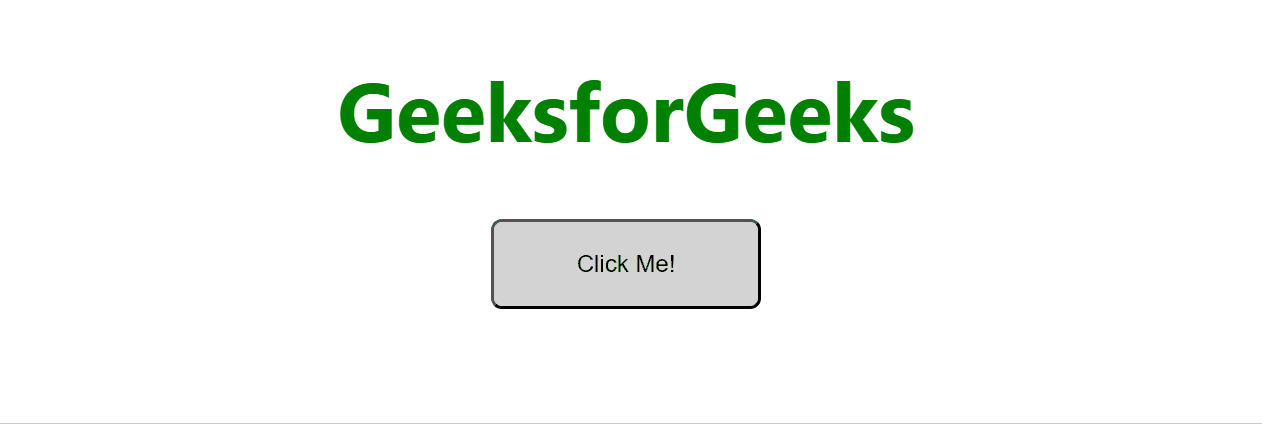
The Complete list of Events are listed below:
Mouse Events:
In React, handling mouse events is important for creating interactive user interfaces.
onClick |
This event fires when user click mouse left button.
|
onDoubleClick |
This event triggers when user click mouse button twice.
|
onMouseDown |
This event occurs when mouse button pressed on any tag.
|
onMouseUp |
This event triggers when button released after press.
|
onMouseMove |
This event occurs when mouse cursor move on a particular tag or element.
|
onMouseEnter |
This event fires when a mouse cursor move inside in a HTML Element.
|
onMouseLeave |
This event occurs when mouse cursor leaves HTML Element boundaries.
|
Form Event:
In React, handling form events is a crucial part of creating interactive and dynamic user interfaces.
onChange |
This event triggers when the value of input tag changes.
|
onInput |
This event fires when the value of input field gets changed.
|
onSubmit |
This event triggers when user submit a form using Submit button.
|
onFocus |
This event fires when user click on any input tag and that tag active to enter data.
|
onBlur |
This event occurs when element is not longer active.
|
Clipboard Events:
Clipboard events in React allow you to interact with the user’s clipboard, providing a way to handle copy, cut, and paste operations.
onCopy |
This event occurs when user copy data from any particular element.
|
onCut |
This event occurs when user cut data from any particular element.
|
onPaste |
This event occurs when user paste data from any particular element.
|
Touch Events:
In React, you can handle touch events to create touch-friendly and mobile-responsive user interfaces.
onTouchStart
|
This event occurs when a user touches the screen.
|
onTouchMove
|
This event fires when user touch and move their finger without removing finger.
|
onTouchEnd
|
This event occurs one touch released by user.
|
onTouchCancel
|
This event hits when touch cancels by user.
|
Pointer Events:
Pointer events in React are a set of events that handle different input devices, including mouse, touch, and pen interactions.
onPointerDown |
This event works when a pointing device inititates pointing screen.
|
onPointerMove |
This event works when pointing device starts initiating and moving the pointer.
|
onPointerUp |
This event triggers when user released the button from pointing device after pointing a tag.
|
onPointerCancel |
This event occurs when user cancels their touch on screen.
|
UI Events:
It seems there might be a bit of confusion in your question. The term “UI event” is quite broad and could refer to various types of events that occur in a user interface.
onScroll |
This event occurs when user start scrolling the page.
|
onResize |
This event occurs when the size of browser is changed.
|
Keyboard Events:
In React, you can handle keyboard events using synthetic events provided by React.
onKeyDown |
This event occurs when a user presses the key from keyboard.
|
onKeyPress |
This event occurs when a user presses the key from keyboard.
|
onKeyUp |
This event occurs when a user presses and released the key from keyboard.
|
Share your thoughts in the comments
Please Login to comment...