React onKeyUp Event
Last Updated :
29 Nov, 2023
React onKeyUp is an event listener that is used to detect the key release event in a browser using JavaScript. This event occurs once after the key is pressed and released.
It is similar to the HTML DOM onkeyup event but uses the camelCase convention in React.
Syntax:
<input onKeyUp={keyUpFunction}/>
Parameter :
- KeyUpFunction is a function that will call once any key is released from Keyboard.
Return type:
- event: It is an event object containing information about the event like target element and values
Example 1: In this example, we implemented an input field in which we press and release any key from the keyboard, and on each key, we will check the onKeyUp Event listener using JSX. We will print the key in the user interface so that the user can understand which key is released.
Javascript
import { useState } from "react" ;
import './App.css' ;
function App() {
const [key, setkey] = useState();
const keyUp = (event) => {
setkey(event.key)
}
return (
<div className= "App" >
<h1>GeeksforGeeks</h1>
{key ? <h2>Key UP : {key}</h2> : null }
<input type= 'text'
onKeyUp={keyUp}
placeholder= 'Press & Release Key here...' />
</div>
);
}
export default App;
|
Output:
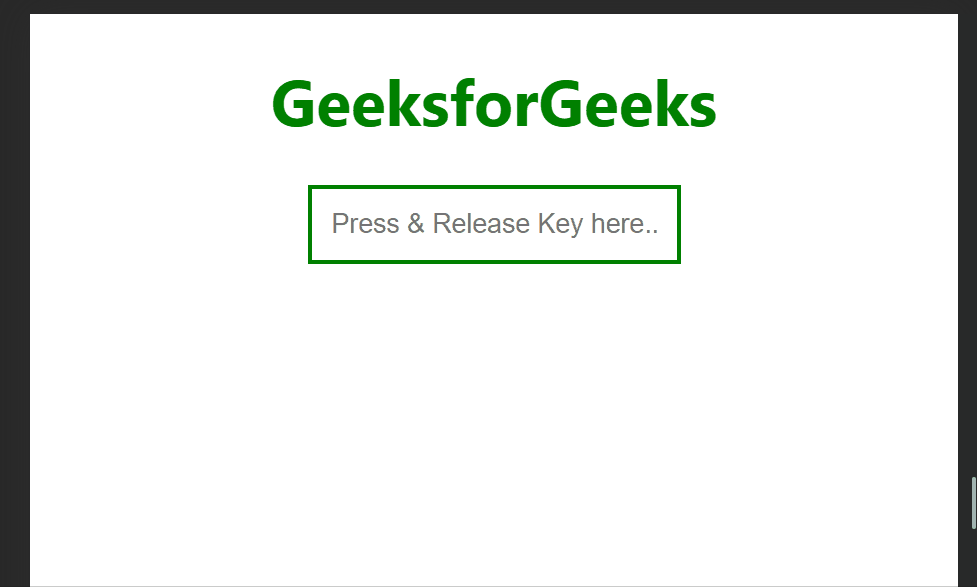
Example 2: In this example, we implemented onKeyUp functionality in the input field. When the user releases any key, they can see which key was released in the console of the browser.
Javascript
import './App.css' ;
function App() {
const keyUp = (event) => {
console.log(event.key)
}
return (
<div className= "App" >
<h1>GeeksforGeeks</h1>
<input type= 'text'
onKeyDown={keyUp}
placeholder= 'Press & Release here...' />
</div>
);
}
export default App;
|
Output:
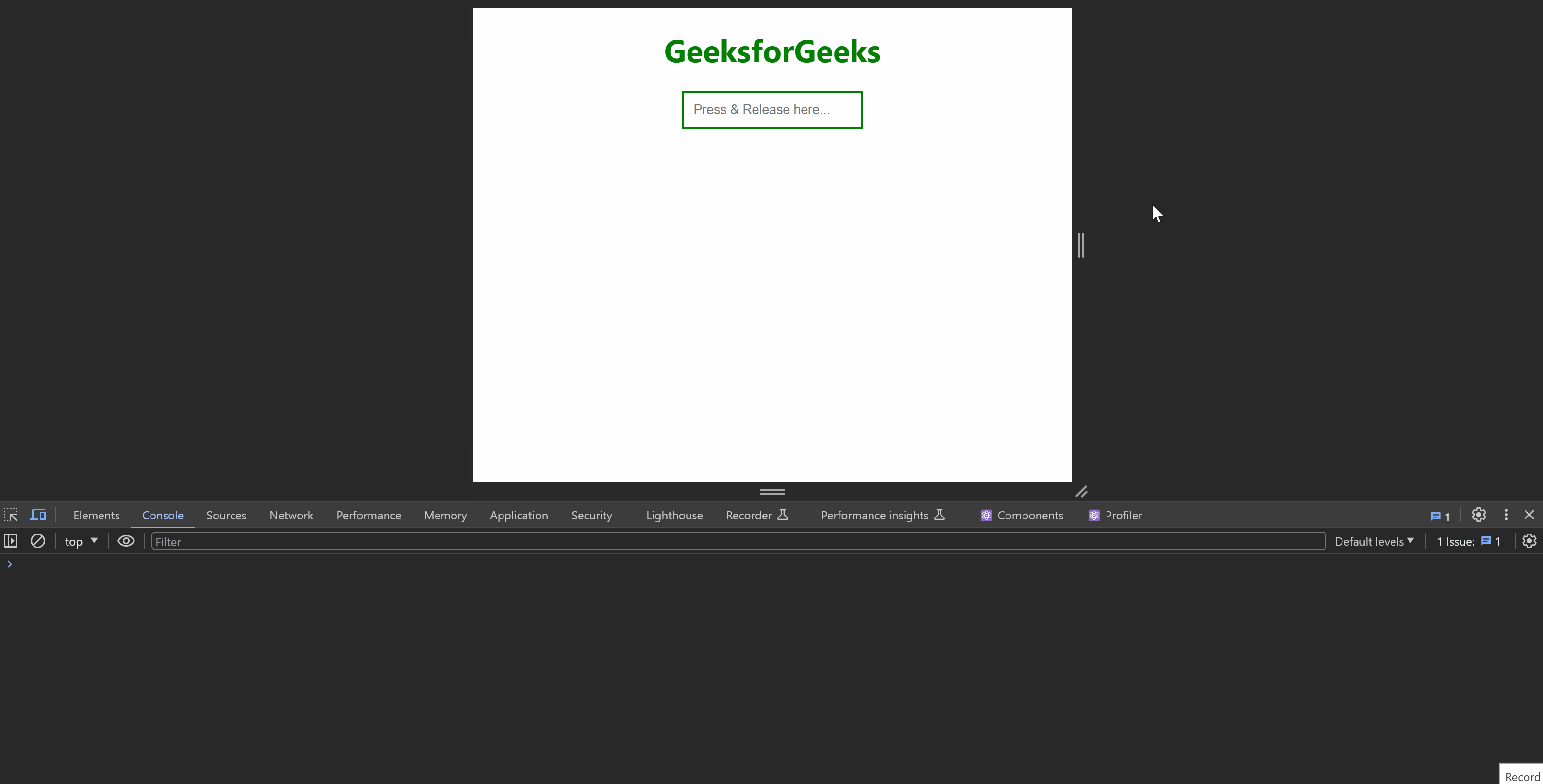
Share your thoughts in the comments
Please Login to comment...