React onChange Event
Last Updated :
28 Feb, 2024
React onChange is an event handler that triggers when there is any change in the input field.
This event captures the changes in an Input Field and executes the handler function. It is fired when the input field is modified and loses focus. It is one of the form events that updates when the input field is modified.
It is similar to the HTML DOM onchange event but uses the camelCase convention in React.
Syntax:
<input onChange={handleChange} >
Parameter:
- handleChange: It is a function call that includes the code to be executed when an event triggers
Return Type:
- event: It is an event object containing information about the event like target element and values
React onChange Event Examples
Below are the simple implementations for React onChange Event.
Example 1: Updating state using onChange Event
This example demonstrates the use of the onChange event handler to update the states in React .
Javascript
import React, { useState } from "react";
function App() {
const [value, setValue] = useState("");
function handleChange(e) {
setValue(e.target.value);
}
return (
<div
style={{ textAlign: "center", margin: "auto" }}
>
<h1 style={{ color: "Green" }}>
GeeksforGeeks
</h1>
<h3>
Exemple for React onChange Event Handler
</h3>
<input value={value} onChange={handleChange} />
<br />
<div>User Input:- {value}</div>
</div>
);
}
export default App;
|
Output:
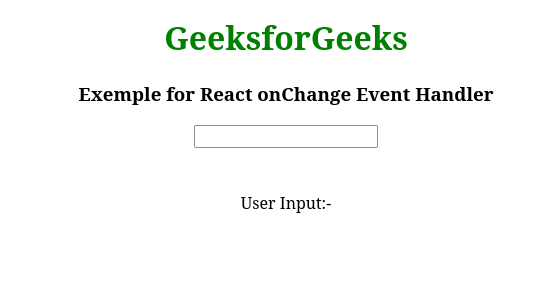
Example 2: Handlling select input using React onChange Event
This example demonstrate the use of the onChange event handler in select input.
Javascript
import React, { useState } from "react";
function App() {
const [value, setValue] = useState("HTML");
function handleChange(e) {
setValue(e.target.value);
}
return (
<div
style={{ textAlign: "center", margin: "auto" }}
>
<h1 style={{ color: "Green" }}>
GeeksforGeeks
</h1>
<h3>
Exemple for React onChange Event Handler
</h3>
<select value={value} onChange={handleChange}>
<option value={"HTML"}>HTML</option>
<option value={"CSS"}>CSS</option>
<option value={"JavaScript"}>
JavaScript
</option>
</select>
<br />
<div>User Input:- {value}</div>
</div>
);
}
export default App;
|
Output:
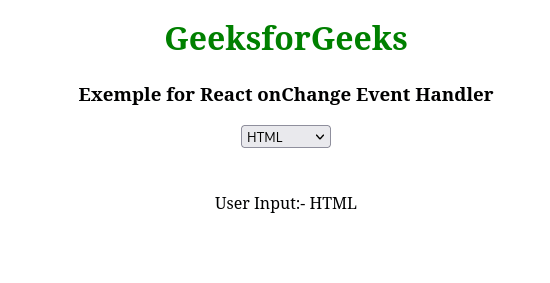
Share your thoughts in the comments
Please Login to comment...