React onBlur Event
Last Updated :
04 Dec, 2023
React onBlur
event is an event that triggers when an element loses focus. In web development, this event is commonly used with HTML form elements or in JSX syntax in React, such as input fields, text areas, and buttons.
It is similar to the HTML DOM onblur event but uses the camelCase convention in React.
Syntax:
<input onBlur={handleOnBlur}/>
Parameter: The function handleOnBlur which is invoked by onBlur when the event triggers.
Return Type:
It is an event object containing information about the methods and is triggered when an element comes in inactive state after active state
Example 1:
The code defines a React component with a controlled input field, utilizing state for user input. It logs a message when the input field loses focus (`onBlur`) and updates the state as the user types (`onChange`).
Javascript
import React, { useState } from 'react' ;
function App() {
const [value, setValue] = useState( '' );
const handleBlur = () => {
console.log( 'Input blurred' );
};
return (
<form action= "" >
<label htmlFor= "" >Name:</label>
<input
type= "text"
value={value}
placeholder= 'Write Your Name'
onChange={
(e) =>
setValue(e.target.value)}
onBlur={handleBlur}
/>
</form>
);
}
export default App;
|
Output:
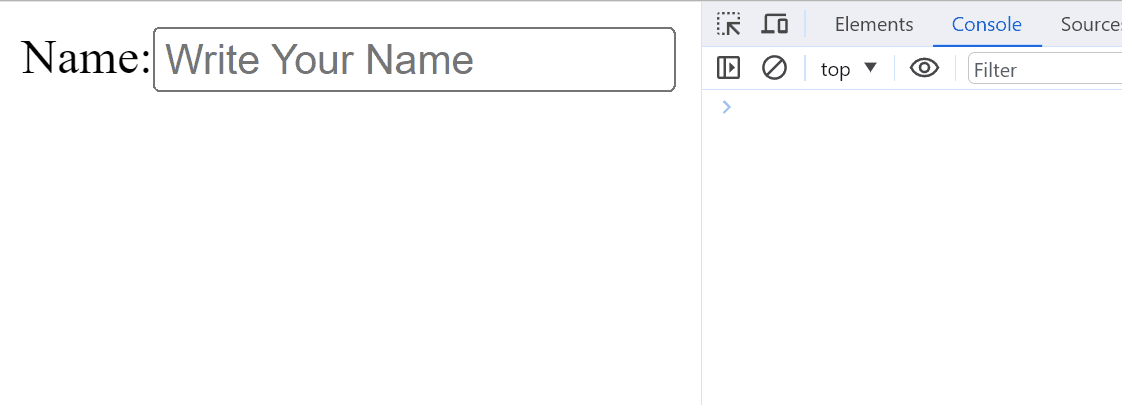
Output
Example 2: The React component uses a controlled textarea, managing its content in the component state. It simulates auto-saving the content by logging it when the textarea loses focus (`onBlur`).
Javascript
import React, { useState } from 'react' ;
function App() {
const [content, setContent] = useState( '' );
const handleBlur = () => {
console.log( 'Content auto-saved:' , content);
};
return (
<div>
<p>Remark:</p>
<textarea
value={content}
onChange=
{
(e) =>
setContent(e.target.value)
}
onBlur={handleBlur}
/>
</div>
);
}
export default App;
|
Output:
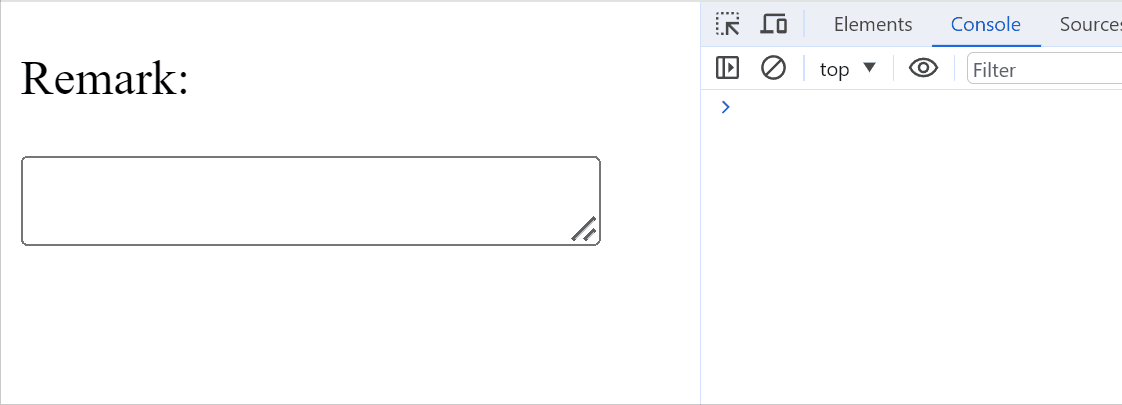
Output
Share your thoughts in the comments
Please Login to comment...