React onDoubleClick Event
Last Updated :
04 Dec, 2023
The onDoubleClick
event is a handler designed to capture and respond to double clicks on specific elements. It serves as an event listener that triggers a specified function when a user rapidly clicks on the associated element twice within a short time frame.
It is similar to the HTML DOM ondblclick event but uses the camelCase convention in React.
Syntax:
onDoubleClick={onDoubleClickHandler}
Parameter: The callback function onDoubleClickHandler which is invoked when onDoubleClick event is triggered.
Return Type:
It is an event object containing information about the methods and is triggered when mouse is double clicked.
Example 1:
This React functional component displays a greeting message and a button, and when the button is double-clicked, it triggers the `onDoubleClickHandler`, logging a message to the console.
Javascript
import React, { useState } from "react" ;
import "./App.css" ;
function App() {
const onDoubleClickHandler = () => {
console.log( "You have Clicked Twice" );
};
return (
<div className= "App" >
<h1> Hey Geek!</h1>
<button onDoubleClick={onDoubleClickHandler}>
Double Click Me!
</button>
</div>
);
}
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
.App>h 2 {
text-align : center ;
}
.App>button {
width : 17 rem;
font-size : larger ;
padding : 2 vmax auto ;
height : 2.6 rem;
color : white ;
background-color : rgb ( 34 , 34 , 33 );
border-radius: 10px ;
}
button:hover {
background-color : rgb ( 80 , 80 , 78 );
}
|
Output:
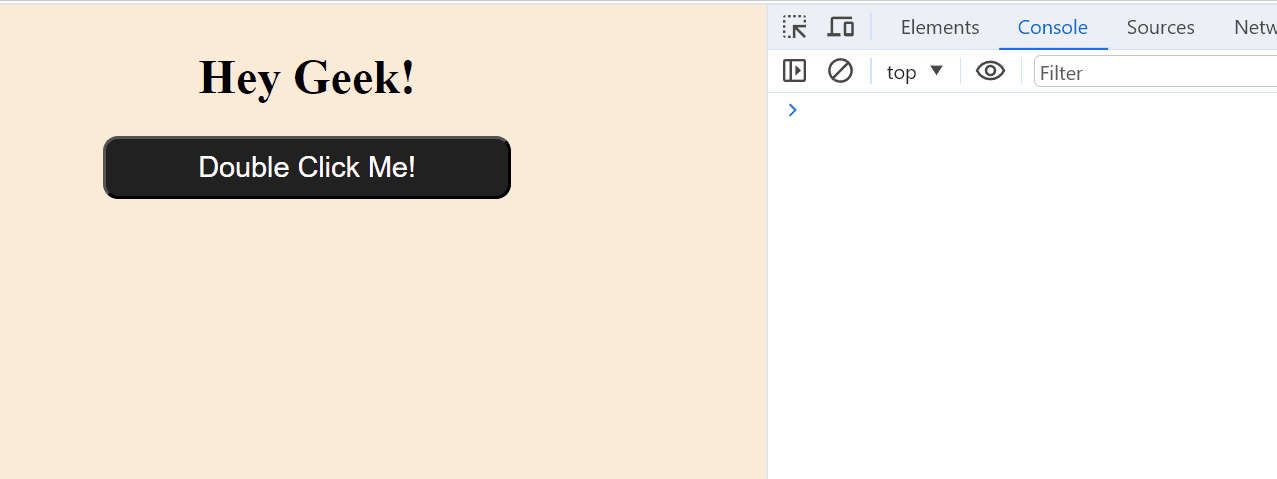
Output
Example 2: This React functional component initializes a state variable `num` and, when a button is double-clicked, invokes the `handleDoubleClick` function to update `num` by incrementing it by 2, displaying the updated value.
Javascript
import React, { useState } from 'react' ;
import './App.css'
const App = () => {
const [num, setNum] = useState(0);
const handleDoubleClick = () => {
setNum(num + 2);
};
return (
<div className= 'App' >
<h2> {num}</h2>
<button onDoubleClick={handleDoubleClick}>
Double Click me
</button>
</div>
);
};
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
.App>h 2 {
text-align : center ;
font-size : 2 rem;
}
.App>button {
width : 15 rem;
font-size : larger ;
padding : 2 vmax auto ;
height : 2.6 rem;
color : white ;
background-color : rgb ( 34 , 34 , 33 );
border-radius: 10px ;
}
button:hover {
background-color : rgb ( 80 , 80 , 78 );
}
|
Output:
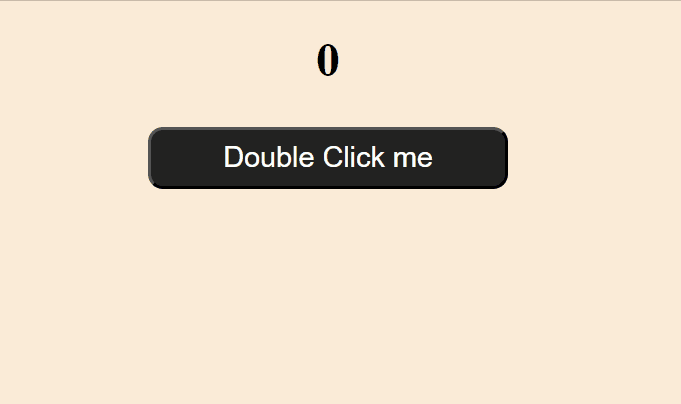
Output
Share your thoughts in the comments
Please Login to comment...