React onMouseDown Event
Last Updated :
04 Dec, 2023
React onMouseDown event serves as a handler designed to detect and respond to the action of pressing a mouse button while positioned over a particular element.
It is similar to the HTML DOM onmousedown event but uses the camelCase convention in React.
Syntax:
onMouseDown = {handleMouseDown}
Parameter: The callback function handleMouseDown which is triggered when onMouseDown event is fired.
Return Type:
It is an event object containing information about the methods and is triggered when the mouse is down.
Example 1:
This React functional component initializes a state variable `count` and increments it by 1 when a button is pressed down, displaying the updated count value.
Javascript
import React, { useState } from 'react' ;
import './App.css'
const App = () => {
const [count, setCount] = useState(0);
const handleMouseDown = () => {
setCount(count + 1);
};
return (
<div className= 'App' >
<h2>Count: {count}</h2>
<button onMouseDown={handleMouseDown}>
Increment on Mouse Down
</button>
</div>
);
};
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
.App>h 2 {
text-align : center ;
font-size : 2 rem;
}
.App>button {
width : 20 rem;
font-size : larger ;
padding : 2 vmax auto ;
height : 2.6 rem;
color : white ;
background-color : rgb ( 34 , 34 , 33 );
border-radius: 10px ;
}
button:hover {
background-color : rgb ( 80 , 80 , 78 );
}
|
Output:
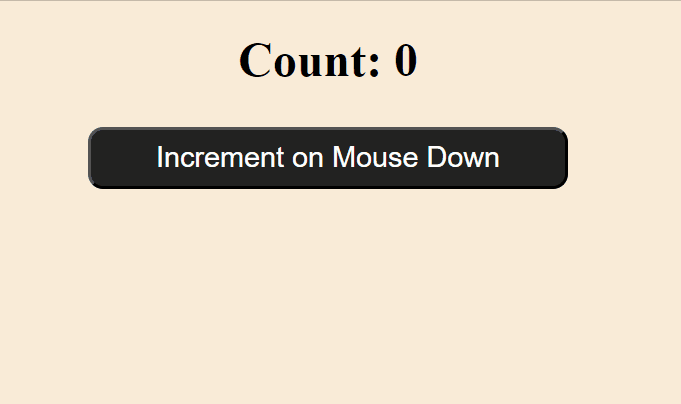
Output
Example 2: This React functional component creates a container that changes its background color to light blue when the mouse is pressed down and reverts to antique white when the mouse is released, providing a visual effect on click-and-hold interaction.
Javascript
import React, { useState } from 'react' ;
import './App.css'
const App = () => {
const [isMouseDown, setIsMouseDown] = useState( false );
const handleMouseDown = () => {
setIsMouseDown( true );
};
const handleMouseUp = () => {
setIsMouseDown( false );
};
const containerStyle = {
backgroundColor: isMouseDown ? 'lightblue' : 'antiquewhite' ,
padding: '20px' ,
};
return (
<div className= 'App' style=
{containerStyle}
onMouseDown={handleMouseDown}
onMouseUp={handleMouseUp}>
<h2>Click and hold to change color</h2>
</div>
);
};
export default App;
|
CSS
.App {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
}
body {
background-color : antiquewhite;
}
|
Output:
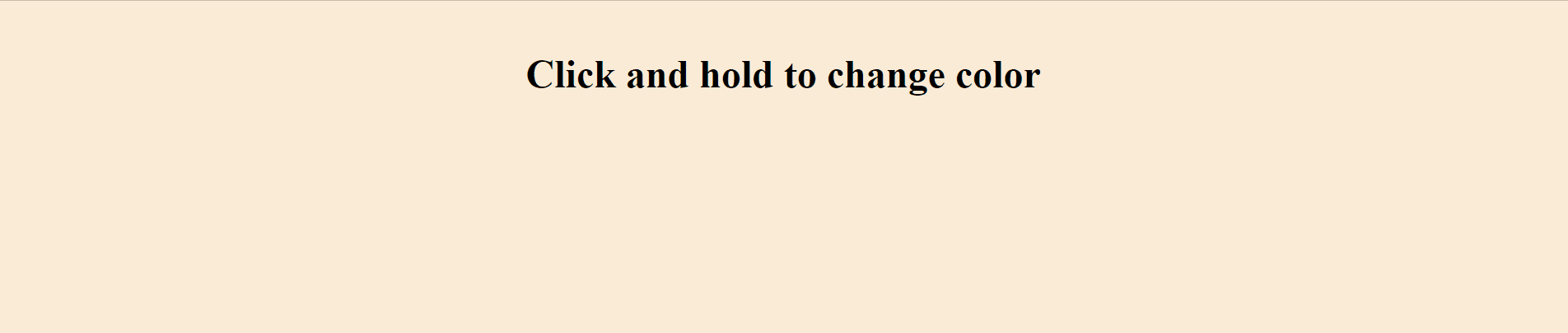
Output
Share your thoughts in the comments
Please Login to comment...