React Events
Last Updated :
06 Mar, 2024
React Events are user interactions with the web application, such as clicks, keyboard input, and other actions that trigger a response in the user interface. Just like HTML DOM, React also acts upon the events.
React Events
React events are the actions due to user interaction or system events. React allows developers to handle these events using a declarative approach, making it easier to create interactive and dynamic user interfaces.
Event declaration in react is different from HTML, React uses:
- the camelCase convention instead of lowercase letters.
- JSX is used in react instead of plain html.
Event Declaration in HTML
<button onclick="handleClick()">
Click Me!
</button>
Event Declaration in React:
<button onClick={handleClick}>
Click Me!
</button>
Commonly Used React Event Handlers:
React Event
|
Description
|
onClick
|
This event is used to detect mouse click in the user interface.
|
onChange
|
This event is used to detect a change in input field in the user interface.
|
onSubmit
|
This event fires on submission of a form in the user interface and is also used to prevent the default behavior of the form.
|
onKeyDown
|
This event occurs when user press any key from keyboard.
|
onKeyUp
|
This event occurs when user releases any key from keyboard.
|
onMouseEnter
|
This event occours when mouse enters the boundary of the element
|
Adding React Events
React events syntax is in camelCase, not lowercase. If we need to set events in our code, we have to pass them, just like props or attributes, to JSX or HTML. We have to pass a function regarding the process we want to do on fire of this event.
React Events Syntax:
onClick={function}
Passing Arguments in React Events
In React Events, we pass arguments with the help of arrow functions. Like functions, we create parenthesis and pass arguments, but here it is not possible; we have to use an arrow function to pass arguments.
React Events Arguments Syntax:
onClick={()=>{function(argument)}}
React Event Object
React Events handlers have an object called Events, which contains all the details about the event, like type, value, target, ID, etc. So we can pass that event as an argument and use that event object in our function.
React Event Object Syntax:
onClick={(event)=>{function(event)}}
React Events Example:
This example demonstrates the implementation of Event Handler in React.
Javascript
import { useState } from "react" ;
import "./App.css" ;
function App() {
const [inp, setINP] = useState( "" );
const [name, setName] = useState( "" );
const clk = () => {
setName(inp);
setINP( "" );
};
return (
<div className= "App" >
<h1>GeeksforGeeks</h1> \
{name ? <h2>Your Name:{name}</h2> : null }
<input
type= "text"
placeholder= "Enter your name..."
onChange={(e) => setINP(e.target.value)}
value={inp}
/>
<button onClick={clk}>Save</button>{ " " }
</div>
);
}
export default App;
|
Output:
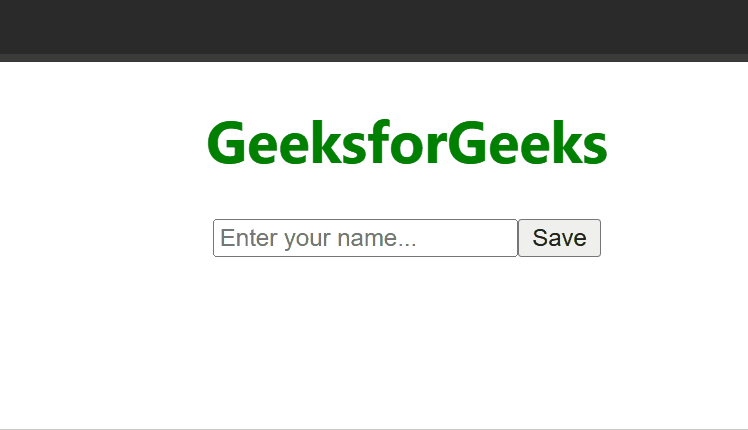
Share your thoughts in the comments
Please Login to comment...