Python Program to Delete Specific Line from File
Last Updated :
26 Sep, 2021
In this article, we are going to see how to delete the specific lines from a file using Python
Throughout this program, as an example, we will use a text file named months.txt on which various deletion operations would be performed.
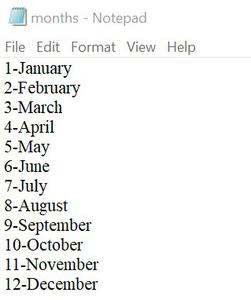
Method 1: Deleting a line using a specific position
In this method, the text file is read line by line using readlines(). If a line has a position similar to the position to be deleted, it is not written in the newly created text file.
Example:
Python3
try :
with open ( 'months.txt' , 'r' ) as fr:
lines = fr.readlines()
ptr = 1
with open ( 'months.txt' , 'w' ) as fw:
for line in lines:
if ptr ! = 5 :
fw.write(line)
ptr + = 1
print ( "Deleted" )
except :
print ( "Oops! something error" )
|
Output:
Deleted
‘5-May’ was written on the 5th line which has been removed as shown below:
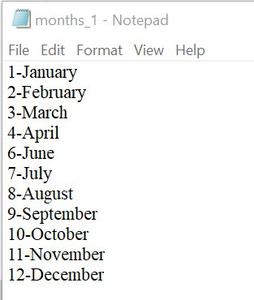
Method 2: Deleting a line using a text that matches exactly with the line
In this method, after reading the file, each line is checked if it matches with the given text exactly. If it does not match, then it is written in a new file.
Python3
try :
with open ( 'months.txt' , 'r' ) as fr:
lines = fr.readlines()
with open ( 'months_2.txt' , 'w' ) as fw:
for line in lines:
if line.strip( '\n' ) ! = '8-August' :
fw.write(line)
print ( "Deleted" )
except :
print ( "Oops! something error" )
|
Output:
Deleted
The line having ‘8-August’ exactly has been removed as shown:
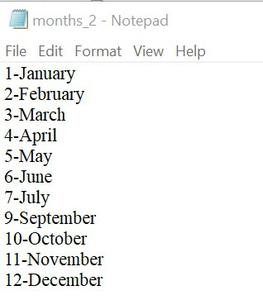
Method 3: Using custom-made logics
Example 1: Deleting lines containing a specified pattern
Here, the lines containing the specified string pattern are removed. The pattern may not be necessarily the whole exact line.
Python3
try :
with open ( 'months.txt' , 'r' ) as fr:
lines = fr.readlines()
with open ( 'months_3.txt' , 'w' ) as fw:
for line in lines:
if line.find( 'ber' ) = = - 1 :
fw.write(line)
print ( "Deleted" )
except :
print ( "Oops! something error" )
|
Output:
Deleted
All the lines containing the pattern ‘ber’ such as ‘9-September’, ’10-October’, ’11-November’, ’12-December’ have been removed.
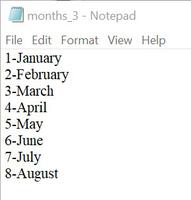
Example 2: Deleting lines with the condition
If we have a condition that the lines in our file must have a minimum length. So, the following example shows how to delete lines not having a minimum specified length.
Python3
try :
with open ( 'months.txt' , 'r' ) as fr:
lines = fr.readlines()
min_len = 7
with open ( 'months_4.txt' , 'w' ) as fw:
for line in lines:
if len (line.strip( '\n' )) > = min_len:
fw.write(line)
print ( "Deleted" )
except :
print ( "Oops! something error" )
|
Output:
Deleted
All the lines not having a length of greater than or equal to 7 have been removed:
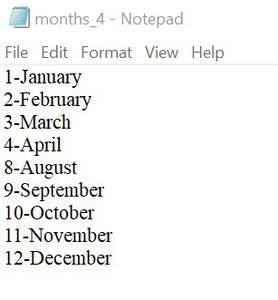
Share your thoughts in the comments
Please Login to comment...