Python is a very powerful programming language and it’s expanding quickly because of its ease of use and straightforward syntax. In this Python Automation Tutorial, we will explore various techniques and libraries in Python to automate repetitive tasks.Â
Automation can save you time and reduce errors in tasks such as data processing, file management, web scraping, Web Automation, API Automation and more.
This Python Automation tutorial will provide you with a step-by-step guide for beginners so that anyone can learn and use automation in Python. Let’s start right up!
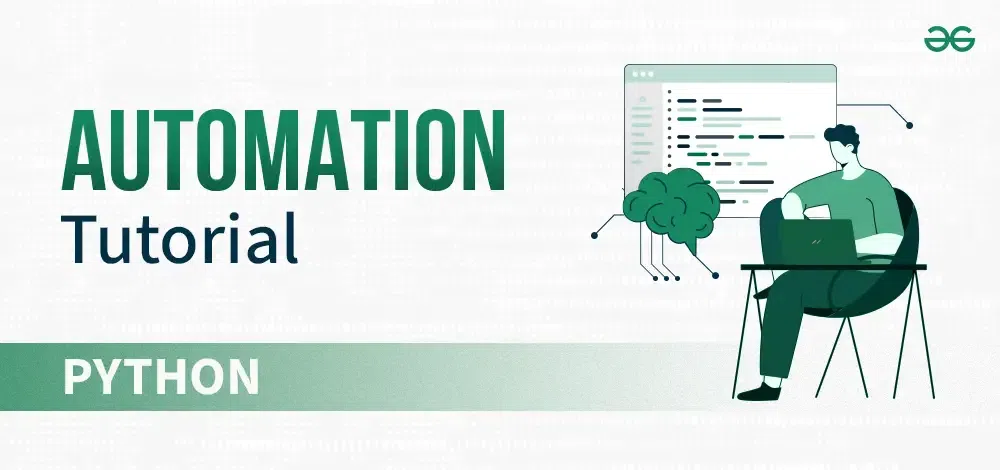
What is Automation?
Automation in Python may refer to the use of the Python programming language to create programs, scripts, or various tools that perform automatic tasks with no use of manual intervention.
Automation is used widely to perform tasks without needing manual intervention. Since, Python is used across various domains, including software development, data analysis, and more. So, there is a need for professionals familiar with automation.
Why is Automation Important?
Automation is a very important aspect of Python Programming and there are many applications and advantages of automation:
- Automation is crucial in today’s fast-paced world as it can help you save time by eliminating repetitive tasks.
- Reduce errors and improve accuracy.
- Increase productivity by allowing you to focus on more important work.
- Perform tasks at a consistent pace 24/7.
Where is Automation Required?
We can use automation in various domains such as:
- Data processing and analysis.
- File and folder management.
- Web scraping and data extraction.
- Task scheduling and reminders.
- GUI automation for repetitive user interactions.
Requirements for Python Automation
Here we have provided you with some of the topics and concepts you should know before starting to learn automation.
1. Python
Python is a high-level, general-purpose, and very popular programming language. Python programming language (latest Python 3) is being used in web development, machine learning applications, along with all cutting-edge technology in the Software Industry.
Python language is being used by almost all tech-giant companies like – Google, Amazon, Facebook, Instagram, Uber… etc.
Python Basics
Python Input/Output
Python Data Types
Python Control Flow
Python Modules for Automation
Here are some of the modules that are very useful for automation:
1. PyAutoGUI
PyAutoGUI is a Python library that allows you to automate tasks by controlling the mouse and keyboard. It’s a powerful tool for automating repetitive tasks, creating macros, and even testing GUI applications.
For more information refer to this article – PyAutoGUI
2. Selenium
Selenium is a powerful tool for automating interactions with web applications. It allows us to script actions that a user would typically perform on a website, such as clicking buttons, filling out forms, and navigating through web pages
For more information refer to this article – Selenium
3. Requests
Automation using the requests library in Python is typically used for making HTTP requests to interact with web services, APIs, or websites programmatically. You can send various types of HTTP requests, such as GET, POST, PUT, DELETE, etc. Once you send a request, you can handle the response, which includes the status code, headers, and content.
For more information refer to this article – Requests
4. BeautifulSoup
Automation using BeautifulSoup is typically used for web scraping tasks. BeautifulSoup is a Python library that makes it easy to extract data from HTML and XML documents. We can use Use BeautifulSoup’s methods to locate and extract specific elements from the HTML document.
For more information refer to this article – BeautifulSoup
4. Pandas
Automation using pandas typically involves automating tasks related to data manipulation, analysis, and processing. Once the data is obtained we want to automate tasks on it.
The data can come from various sources, including CSV files, Excel spreadsheets, databases, web APIs, or web scraping using libraries like BeautifulSoup. We can use pandas to conduct data analysis, including summary statistics, grouping, aggregation, and visualization.
For more information refer to this article – Pandas
How to Automate a Task?
Automating a task might look very complex, but if you know the basics of Python and you follow these steps. You can easily create an automation script.
Step 1: Identify the Task
Before writing the code, you should have it clear what you want your code to do. So having a clear picture of what the task is, will help you in finishing it.
Step 2: Divide the Task into Smaller Steps
Now break down the desired task into smaller tasks and achieve the initial goal. After achieving the initial goal you can then go to the next one. Breaking the main goal into smaller goals helps you in solving one problem at a time.
Step 3: Research Python Libraries
Now you need to find optimal Python libraries that are most suitable for the task. Using the right libraries will increase efficiency and decrease time consumption.
Step 4: Write the Code
Now you just need to write the Python script, that can automate your desired tasks. Use the right libraries and functions to make writing easier.
Step 5: Test the Code
After you have completed the writing part, test your code on different instances and variations. Testing your code will help you in finding the issues with your code. It also helps in building confidence in the results.
Step 6: Update the Code
If your code is not working properly or there are some instances of wrong results, you can update your code and make corrections. In cases where you might want to add additional features to your code, you can do it in this step.
Python Automation Examples
Let’s look at one simple example of automation. In this example, we are automating the task of creating a file and writing text in it.
Python3
file_name = "automated_file.txt"
text = "Hello! This is an automated file with text."
with open (file_name, 'w' ) as file :
file .write(text)
print (f "File '{file_name}' has been created and the message has been written." )
|
Output
File 'automated_file.txt' has been created and the message has been written.
After executing this code, you will find the file with text at the same location/directory as your Python file.
Try more Python automation scripts:
Automating Workflows with Python Scripts
Let’s look at some workflows that can be automated using Python scripts. We will also cover some examples of each workflow to understand the work.
1. Web scraping
Web scraping is a technique to fetch data from websites. While surfing on the web, many websites don’t allow the user to save data for personal use.
One way is to manually copy-paste the data, which is both tedious and time-consuming. Web Scraping is the automation of the data extraction process from websites. This event is done with the help of web scraping software known as web scrapers.
They automatically load and extract data from the websites based on user requirements. These can be custom-built to work for one site or can be configured to work with any website.
Some examples of Web scraping:
- Python Web Scraping Tutorial
- Scraping Reddit with Python and BeautifulSoup
- Scraping Covid-19 statistics using BeautifulSoup
- Web scraping from Wikipedia using Python – A Complete Guide
- Scrape IMDB movie rating and details using Python and saving the details of top movies to .csv file
2. GUI Automation
GUI (Graphical User Interface) automation is the process of automating interactions with graphical elements in the user interface of a software application or system.
GUI automation involves simulating user actions, such as clicking buttons, filling out forms, navigating menus, and interacting with windows, to perform tasks or tests without manual intervention.
GUI automation is commonly used in software testing, repetitive task automation, and robotic process automation (RPA).
Some examples of GUI Automation:
- GUI Automation using Python
- Mouse and keyboard automation using Python
3. Software Testing Automation
Software testing is the process in which a developer ensures that the actual output of the software matches the desired output by providing some test inputs to the software.
Software testing can be divided into two classes, Manual testing and Automated testing. Automated testing is the execution of your tests using a script instead of a human. Here we’ll discuss some of the methods of automated software testing with Python.
Some examples of Test Automation:
- Automated software testing with Python
- Automated Browser Testing with Edge and Selenium in Python
4. API Automation
API automation, often referred to as API testing or automated API testing, is the practice of using automation tools and scripts to test and validate the functionality of Application Programming Interfaces (APIs).
Some examples of API Automation:
5. Robotic Process Automation
Robotics Process Automation (RPA) is a useful and widely emerging technology in the business world nowadays. RPA is based on Machine Learning and Artificial Intelligence (AI) which uses various software robots to perform business-oriented tasks.
Previously, in many organizations, the large volume of data was handled by the humans themselves. But with the use of RPA, all the maintenance of data can be done easily with the help of a few software, and no manual or human interference is needed.
Some examples of Robotic Process Automation:
How to Automate Tasks using Python
Example of Python Automation
10 Projects on Automation for Beginners
Let’s look at some beginner automation project ideas using Python:
- Automating promotional or reminder emails.
- Automated web scraper to find insights from websites
- Automated file organizer
- Automated Excel/Google Sheets editor
- Automated forms
- Automated bot for social media profile handling
- Automated text sender
- Automated data backup tool
- Automated currency converter
- Automated games like flipping coins, rolling dice, etc.
You can try making any one of the projects, to test and enhance your skills in the field of Python automation. You can start from easy automation like flipping a coin and then move on to a harder one.
Conclusion
In conclusion, Python automation helps in various tasks and involves leveraging the capabilities of the Python programming language to streamline and automate various tasks. It improves efficiency and reduces the need for manual intervention.
With the increase in popularity of AI and ML automation tasks have also increased. There is a demand for automation experts who can create automated bots and simplify normal repetitive tasks.
FAQs – Python Automation
Q1. How is Python used for automation?
Python is used for automation by writing scripts. You can automate repetitive tasks like web scraping, file handling, data extraction etc using Python.
Q2. How to use Python for Web Automation?
To use Python for web automation, you need to to have knowledge of some Python modules like Selenium or BeautifulSoup. They can interact with web browser and automate tasks like form submissions.
Q3. What are some useful Python Scripts?
Some of the useful Python scripts are:
- File renamer
- Directory cleaner
- Web scraper
- E-mail sender
- Social media bot
- Automated bot to join calls
Q4. What is QA automation in Python?
QA automation means using automated testing tools to test the software in development. You can make scripts in Python to create a automation that tests softwares.
Q5. Is Python automation a good career?
Yes, it is a good career. Python automation is needed in multiple fields like software testing, web development, and data analysis. With increase in demand of automation professionals, it has become a good career path.
Q6. What is Google automation with Python?
Google automation in Python refers to as using Python scripts to automate tasks with the help of Google APIs, such as handling google sheets or automating google ads.
Q7. How much can a Python automation test engineer earn?
The average salary of a Python automation test engineer is $120,000 per year, which is considered a good salary in the tech industry.
Share your thoughts in the comments
Please Login to comment...