Python requests – POST request with headers and body
Last Updated :
23 Nov, 2021
HTTP headers let the client and the server pass additional information with an HTTP request or response. All the headers are case-insensitive, headers fields are separated by colon, key-value pairs in clear-text string format.
Request with headers
Requests do not change its behavior at all based on which headers are specified. The headers are simply passed on into the final request. All header values must be a string, bytestring, or Unicode. While permitted, it’s advised to avoid passing Unicode header values. We can make requests with the headers we specify and by using the headers attribute we can tell the server with additional information about the request.
Headers can be Python Dictionaries like, { “Name of Header”: “Value of the Header” }
The Authentication Header tells the server who you are. Typically, we can send the authentication credentials through the Authorization header to make an authenticated request.
Example:
Headers = { “Authorization” : ”our_unique_secret_token” }
response = request.post(“https://example.com/get-my-account-detail”, headers=Headers)
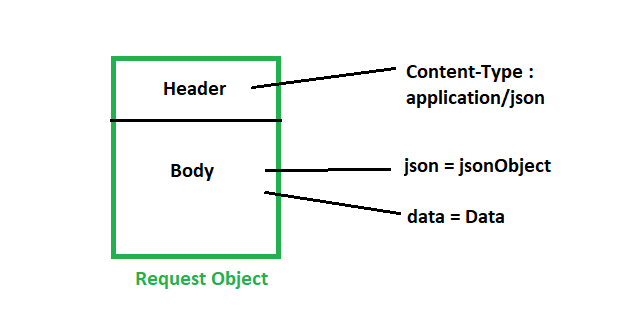
Request Object Structure
Request with body
POST requests pass their data through the message body, The Payload will be set to the data parameter. data parameter takes a dictionary, a list of tuples, bytes, or a file-like object. You’ll want to adapt the data you send in the body of your request to the specified URL.
Syntax:
requests.post(url, data={key: value}, json={key: value}, headers={key:value}, args) *(data, json, headers parameters are optional.)
Given below are few implementations to help understand the concept better.
Example 1: Sending requests with data as a payload
Python3
import requests
data = {
"id" : 1001 ,
"name" : "geek" ,
"passion" : "coding" ,
}
response = requests.post(url, json = data)
print ( "Status Code" , response.status_code)
print ( "JSON Response " , response.json())
|
Output:

Example 2: Sending requests with JSON data and headers
Python
import requests
import json
headers = { "Content-Type" : "application/json; charset=utf-8" }
data = {
"id" : 1001 ,
"name" : "geek" ,
"passion" : "coding" ,
}
response = requests.post(url, headers = headers, json = data)
print ( "Status Code" , response.status_code)
print ( "JSON Response " , response.json())
|
Output:

Share your thoughts in the comments
Please Login to comment...