Implementing Lazy Loading for Functional Components in React
Last Updated :
26 Mar, 2024
React is a popular library, maintained by Meta, which is used for building user interfaces and dynamic websites. It offers several techniques to optimize performance and enhance end-user experience. Lazy loading is one such technique that can be used to improve the performance of web applications by deferring the loading of resources until the browser needs them.
This article will dive deep into lazy loading and how to implement it in a React application.
Prerequisites:
Approach:
React.lazy() enables lazy loading of components, loading them only when needed, not with the initial bundle. Suspense, a built-in React component, manages the loading state, displaying a fallback UI during component fetch. Upon completion, Suspense replaces the fallback UI with the actual component, enhancing React app performance by asynchronously loading components.
Steps to Implement Lazy Loading in React:
Step 1: Set Up a New React App
Open your terminal and run the following commands to create a new React app:
npx create-react-app lazy-loading
cd lazy-loading
Step 2: Create Components
After setting up react environment on your system, we can start implementing components for lazy loading.
Folder Structure:
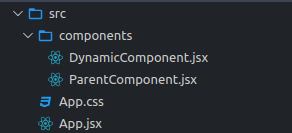
Project Structure for lazy loading project
The updated dependencies in package.json file will look like this:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0"
}
Example: `ParentLazyComponent` uses React.lazy() to lazily load `DynamicComponent` when it’s needed. The Suspense component is used to display a loading indicator while `DynamicComponent` is being loaded. This kind of approach simplifies makes sure that components like `DynamicComponent` are not loaded in initial application bundle and loaded only when needed in case of complex UI applications.
JavaScript
import ParentComponent from './components/ParentComponent';
function App() {
return (
<div className="App">
<ParentComponent />
</div>
);
}
export default App;
Javascript
import React from 'react';
const DynamicComponent = () => {
return (
<div>
<h1>GeeksForGeeks</h1>
</div>
);
};
export default DynamicComponent;
JavaScript
//ParentComponent.js
import React, { Suspense } from 'react';
const DynamicComponent =
React.lazy(() => import('./DynamicComponent'));
const ParentLazyComponent = () => (
<Suspense fallback={<div>Loading...</div>}>
<DynamicComponent />
</Suspense>
);
export default ParentLazyComponent;
Step 3: In your terminal, make sure you’re in the project directory and run below command:
npm start
Output:
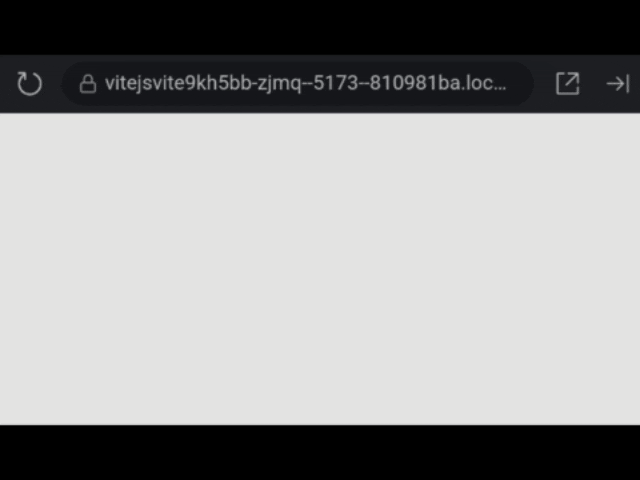
Lazy Loading Output
Share your thoughts in the comments
Please Login to comment...