Explain how useRef helps us work with elements in the DOM?
Last Updated :
15 Feb, 2024
useRef
is like having a magic pointer in your functional components that helps you interact with elements in the HTML DOM (Document Object Model), which is essentially the structure of your webpage.
How does useRef
works ?
- Creating a Reference: When you use
useRef
, it gives you a reference object. It is like a bookmark or sticky note that you can attach to a specific element on your webpage.
- Attaching to DOM Elements: Once you have this reference object, you can attach it to any element in your JSX code by using the
ref
attribute. It’s like sticking your bookmark directly onto that element.
- Accessing and Manipulating: Now that you’ve attached your reference to an element, you can access and manipulate that element directly using the reference. For example, you can focus on an input field, scroll to a specific position, or trigger animations on an element.
- Persisting Across Renders: The neat thing about
useRef
is that it persists across renders. So even if your component re-renders, the reference remains the same, allowing you to maintain consistent access to the element.
- Avoiding Re-renders: Unlike state variables, changing the value of a ref using
useRef
doesn’t trigger a re-render of the component. This makes it ideal for storing values that you want to change without affecting the rendering of your component.
- Preserving State between Renders: Since
useRef
maintains its value across renders, it’s useful for preserving the state between renders, especially if you need to keep track of something but don’t want it to cause your component to re-render unnecessarily.
Example: In this example:
- We create a ref called
inputRef
using the useRef
hook.
- We attach this ref to the input field using the
ref
attribute.
- When the button is clicked, the
focusInput
function is called, which programmatically focuses on the input field using inputRef.current.focus()
.
This example shows us how useRef
allows us to directly interact with DOM elements in a functional component, enabling us to manage focus on the input field without the need for a class component or maintaining state.
Javascript
import React,
{ useRef } from 'react' ;
const FocusInput = () => {
const inputRef = useRef( null );
const focusInput = () => {
inputRef.current.focus();
};
return (
<div>
{ }
<input type= "text" ref={inputRef} />
<button onClick={focusInput}>Focus Input</button>
</div>
);
};
export default FocusInput;
|
Output:
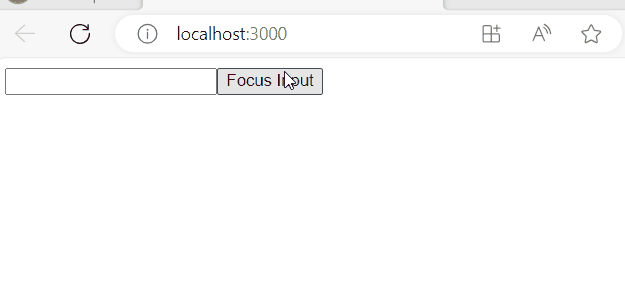
Output
Share your thoughts in the comments
Please Login to comment...