How to Call Function Inside Child Component from Parent Component using useRef hook ?
Last Updated :
30 Nov, 2023
In ReactJS, we can pass the function reference from parent components to child components. However, there are scenarios where you may need to call the function inside the child component from the parent component. It can be done by creating a reference using the useRef hook and createRef method as well.
Prerequisites :
Approach :
Following are the steps to call the function inside the child component from the parent component.
- In the parent component, create a useRef hook.
- Pass this reference to the child component as props.
- Use that reference in the child component wherever required.
Steps to create React Application
Step 1: Create a React application using the following command.
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Project Structure:
.png)
Example: This example demonstrate the use of useRef hook to call a parent component function inside child component by getting text input..
Javascript
import './App.css' ;
import Parent from './Parent' ;
function App() {
return (
<div>
{ }
<Parent />
</div>
);
}
export default App;
|
Javascript
import { useRef, useState } from 'react' ;
import Child from './Child' ;
function Parent() {
const [name, setname] = useState( "" );
const childref = useRef();
return (
<div style={{margin: "20px" }}>
<div>Parent Component</div>
{
}
{name !== '' && <div>{name}</div>}
{
}
<button onClick={() => { childref.current.click() }}
style={{ margin: "2px" }}>
Display name
</button>
{ }
<Child reference={childref} setname={setname} />
</div>
)
}
export default Parent;
|
Javascript
import { useState } from 'react' ;
function Child(props) {
const [input, setinput] = useState( "" );
return (
<div style={{ marginTop: "30px" }}>
<div>Child Component</div>
{
}
<input type= "text" onChange=
{(e) => { setinput(e.target.value) }}>
</input>
{
}
<button ref={props.reference}
style={{ display: "none" }}
onClick={() => { props.setname(input) }} >
</button>
</div>
)
}
export default Child;
|
Steps to Run the application: Use this command in the terminal inside the project directory.
npm start
Output: This output will be visible on the http://localhost:3000/ on the browser window.
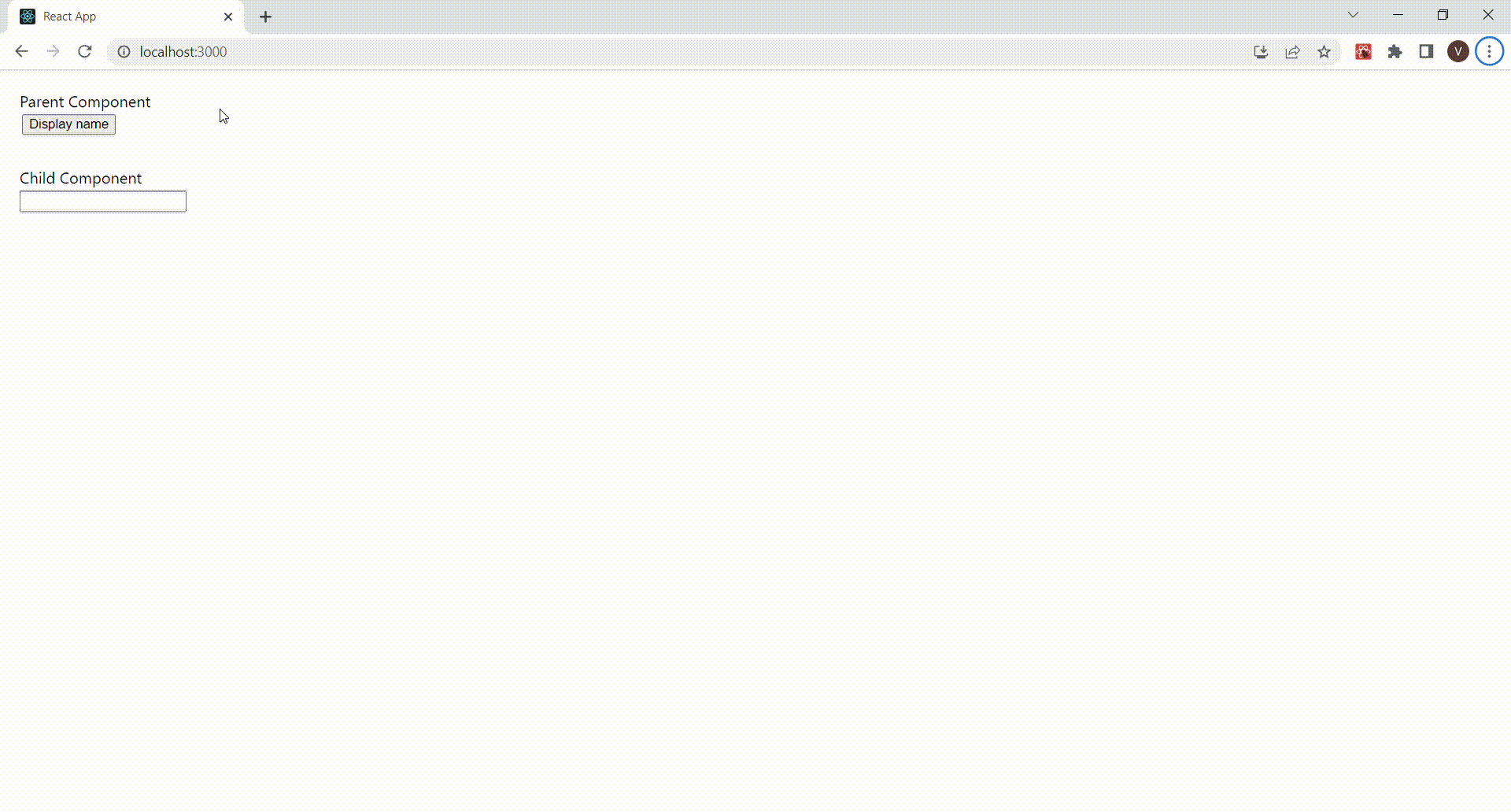
Share your thoughts in the comments
Please Login to comment...