How to use Google Fonts in React ?
Last Updated :
26 Feb, 2024
Google Fonts is a widely used popular library of open-source web fonts that provides a vast collection of typefaces to enhance the visual appeal of web applications.
In this tutorial, we will cover different methods to add custom fonts (Google font) in your React application. Before adding custom fonts to your project, you should be familiar with certain concepts mentioned below.
Prerequisites
How to add Custom Fonts to React Project
There are different methods to add custom fonts to your React Project
Adding the Google fonts in the React application is very easy and we will see the steps to add Google fonts to React project. But first, let us quickly see how to create a React application.
Manually add Google fonts
Manually add Google Fonts in React by including the link tag with the Google Fonts URL in the public/index.html file’s <head> section, then apply the font using CSS in React components.
Steps to Manually use Google Fonts in React:
Step 1:Â Select a Google Font from their website.
Visit the link- Browse Fonts - Google Fonts and select the font you want to add. Here we are using the Monsterrat Font for instance.
Step 2:Â Integrating the font into React
Copy the <link> tag of the font into the public/index.html file of your React app to use in our project:
<link rel=”preconnect” href=”https://fonts.googleapis.com”>
<link rel=”preconnect” href=”https://fonts.gstatic.com” crossorigin>
<link href=”https://fonts.googleapis.com/css2?family=Montserrat:wght@500&display=swap” rel=”stylesheet”>
Step 3: Use the Imported Fonts
body {
font-family: 'Montserrat', sans-serif;
}
Example 1: The below example demonstrates the use of Google fonts in specific react elements.
Javascript
import "./styles.css" ;
export default function App() {
return (
<div className= "App" >
<h1 className= "gfg" >GeeksforGeeks</h1>
<h2>How to use Google fonts in React JS</h2>
<div className= "font-container" >
<p className= "text" >
This text is written in Monsterrat Font
from Google Fonts{ " " }
</p>
</div>
</div>
);
}
|
CSS
.App {
text-align : center ;
}
.gfg {
color : green ;
}
.font-container {
font-family : "Montserrat" , sans-serif ;
}
.text {
font-size : 30px ;
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1" />
< link rel = "preconnect"
< link rel = "preconnect"
< link href =
rel = "stylesheet" >
< title >React App</ title >
</ head >
< body >
< div id = "root" ></ div >
</ body >
</ html >
|
Step to run the application: Open the terminal and type the following command. Â
npm start
Output:Â
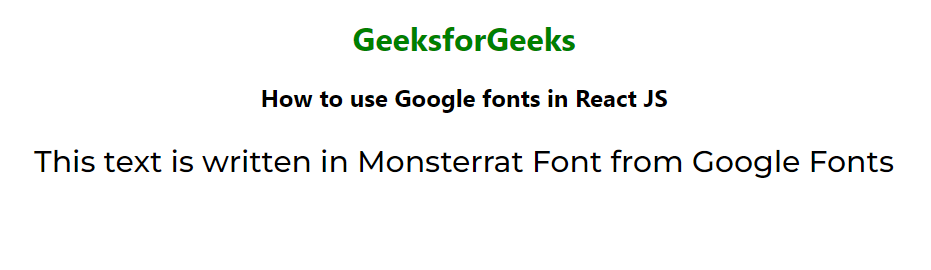
Using styled-components Library
Utilize the styled-components library in React to apply Google Fonts by defining component styles and specifying the desired font-family property using the font import syntax.
To Install the Required Module, use this command in the terminal
npm i styled-components
The Updated dependencies in package.json file.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"styled-components": "^6.1.1",
"web-vitals": "^2.1.4"
},
Example 2: This example demonstrates the usage of the styled-components library in the React app.
Javascript
import "./styles.css" ;
import React from "react" ;
import styled from "styled-components" ;
const StyledText = styled.h1`
font-family: "Monsterrat" , sans-serif;
font-size: 30px;
`;
export default function App() {
return (
<div className= "App" >
<h1 className= "gfg" >GeeksforGeeks</h1>
<h2>How to use Google fonts in React JS</h2>
<div>
<StyledText>
Welcome to GeeksforGeeks!
</StyledText>
</div>
</div>
);
}
|
CSS
.App {
text-align : center ;
}
.gfg {
color : green ;
}
.text {
font-size : 30px ;
}
|
Step to run the application: Open the terminal and type the following command. Â
npm start
Output:
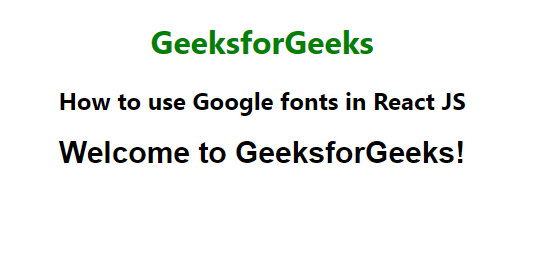
Using @import inside CSS
Directly import the required fonts in the CSS file using the @import command
Step 2: Use the Imported Fonts
body {
font-family: 'Comfortaa', sans-serif;
}
Step to run the application: Open the terminal and type the following command. Â
npm start
Common Errors While Using Custom Font in React
Some common errors faced by developers while adding custom fonts to their React projects are:
1. Font Not Found or not loading
Custom font may not be applied to the application because of font file error. Check if the font file path is correct and if the font file is imported correctly.
2. Using Unsupported Font Formats
React primarily supports .ttf and .otf files. Make sure to convert unsupported file types before adding them to your project.
3. Font Loading Delay
Initially, the application displays default fonts and later changes it to the custom font. You can try optimizing fonts or preloading them.
4. Performance Issues
Large custom font files can impact the application’s performance. Make sure to compress large font files before using or using font optimization.
5. Platform Specific Errors
Each platform (android and IOS ) has different font handling operations. Some fonts may not be compatible with Android or IOS. Follow specific guidelines for each platform.
Wrapping Up
Fonts are the building block of better user experience. Using custom fonts allows us to create unique applications and differentiate them from other applications.
This tutorial shows three methods to use Google Fonts in the React application. Each method is explained with easy-to-follow steps and examples. Create your unique ReactJS by adding custom fonts to your project after completing this guide.
Share your thoughts in the comments
Please Login to comment...