Implementing Drag and Drop Functionality with useRef
Last Updated :
04 Apr, 2024
Implementing drag-and-drop functionality using React’s useRef
hook involves creating a reference to the draggable element and handling drag events. The useRef
hook allows us to access and manipulate DOM elements directly in React functional components. By using useRef
, we can track the position and behaviour of the dragged element during drag-and-drop interactions efficiently.
Output Preview:
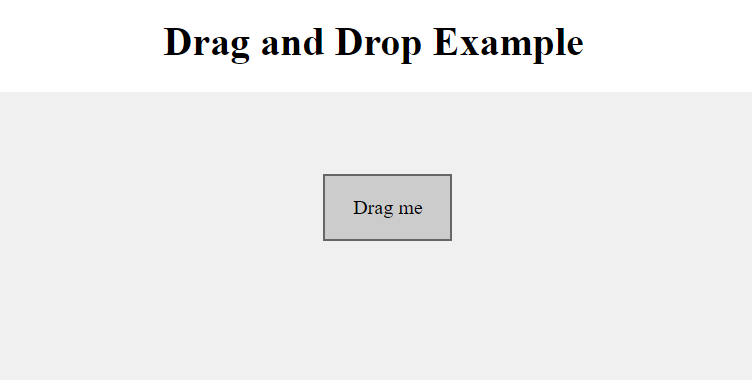
Prerequisites:
Steps to Setup the Application:
Step 1: Create a reactJS application by using this command
npx create-react-app drag-and-drop-app
Step 2: Navigate to project directory
cd drag-and-drop-app
Folder Structure:
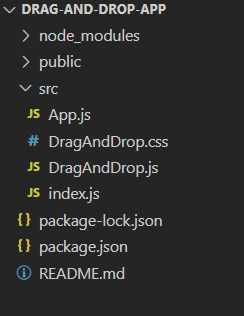
Approach to create Drag & Drop Functionality with useRef :
- First define CSS styles for the drag-and-drop container and draggable items using classes like
.drag-and-drop
and .drag-item
. - Use
useRef
in the React component to create a reference to the draggable item (dragItemRef
in this example). - Implement
handleDragStart
function to set data during drag start and update the dragItemRef
with the dragged element. - Create
handleDragOver
function to prevent default behavior, calculate new position based on mouse coordinates, and update the dragged element’s position accordingly. - Render the drag-and-drop container and draggable item components in your main App component (
App.js
) and handle drag events accordingly.
Example: Illustration to design drag and drop functionality using useref.
CSS
/* DragAndDrop.css */
.drag-and-drop {
width: 100vw;
height: 100vh;
background-color: #f0f0f0;
}
h1 {
text-align: center;
}
.drag-item {
width: 100px;
height: 50px;
background-color: #ccc;
border: 2px solid #666;
display: flex;
justify-content: center;
align-items: center;
font-size: 16px;
cursor: move;
position: absolute;
}
JavaScript
// DragAndDrop.js
import React, { useRef } from 'react';
import './DragAndDrop.css';
const DragAndDrop = () => {
const dragItemRef = useRef(null);
const handleDragStart = (e) => {
const target = e.target;
e.dataTransfer.setData('text/plain', '');
dragItemRef.current = target;
};
const handleDragOver = (e) => {
e.preventDefault();
const target = dragItemRef.current;
if (!target) return;
const x = e.clientX - target.offsetWidth / 2;
const y = e.clientY - target.offsetHeight / 2;
target.style.left = x + 'px';
target.style.top = y + 'px';
};
return (
<div
className="drag-and-drop"
onDragOver={handleDragOver}>
<div
className="drag-item"
draggable="true"
onDragStart={handleDragStart}>
Drag me
</div>
</div>
);
};
export default DragAndDrop;
JavaScript
// App.js
import React from 'react';
import DragAndDrop from './DragAndDrop';
const App = () => {
return (
<div>
<h1>Drag and Drop Example</h1>
<DragAndDrop />
</div>
);
};
export default App;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output: Your project will be shown in the URL http://localhost:3000/
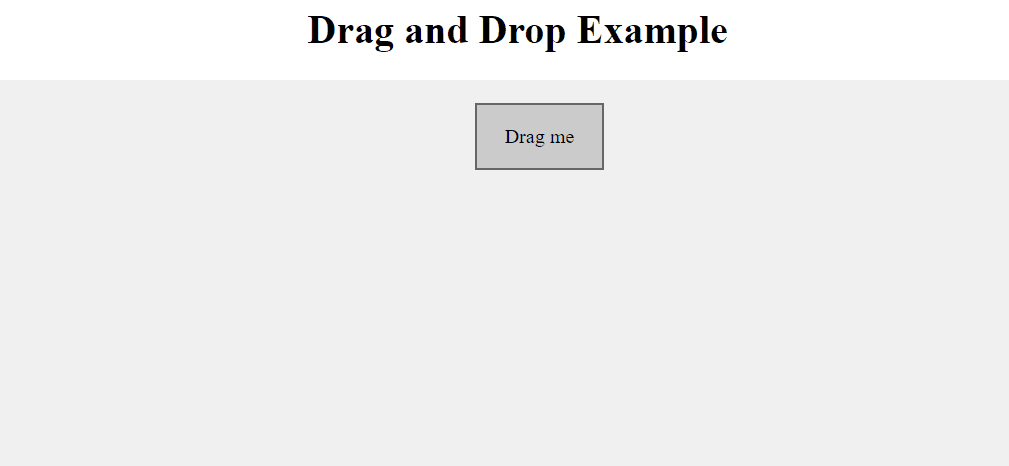
Share your thoughts in the comments
Please Login to comment...