How useRef Hook is useful in React ?
Last Updated :
05 Mar, 2024
Hooks provides a way to useState and other React features in functional components without the need for class components. We can either use built-in hooks or customize and make our own. One of those hooks in useRef. It allows reference to a value that does not require rendering. It can be used to store a mutable value that does not cause a re-render when updated. It can be used to access a DOM element directly.
Primary Uses of useRef:
1. Accessing DOM elements
Referring to DOM elements directly within your component is a common use case for useRef. Instead of employing DOM queries like document.getElementById, useRef allows direct referencing of elements.
Javascript
import React, { useRef } from 'react' ;
const CustomComponent = () => {
const inputRef = useRef( null );
const handleClick = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type= "text" />
<button
onClick={handleClick}>
Focus Input
</button>
</div>
);
};
|
2. Storing previous values
Utilizing useRef enables the storage of a value that persists across renders without triggering re-rendering when updated. This proves beneficial for maintaining previous values without causing unnecessary renders.
Javascript
import React, {
useEffect,
useRef
} from 'react' ;
const CustomValueComponent = ({ value }) => {
const prevValueRef = useRef();
useEffect(() => {
prevValueRef.current = value;
}, [value]);
return (
<div>
<p>Current Value: {value}</p>
<p>Previous Value: {prevValueRef.current}</p>
</div>
);
};
|
3. Navigating External Libraries
In certain scenarios, when incorporating external libraries that require a mutable reference to data, useRef can prove to be quite useful. Keep in mind that as useRef doesn’t induce re-renders, if you require component updates to be reflected in the UI, useState should be preferred. Reserve the use of useRef for managing mutable values or references without influencing component rendering.
Javascript
import React, {
useEffect,
useRef
} from 'react' ;
import externalLibrary from 'some-external-library' ;
const CustomLibraryComponent = () => {
const dataRef = useRef([]);
useEffect(() => {
externalLibrary.updateData(dataRef.current);
}, []);
};
|
Combining useRef, useImperativeHandle, and forwardRef enables the creation of custom input components with programmatically focused input methods. This approach offers a controlled and explicit means of interacting with a child component’s methods or properties from its parent.
Javascript
import React, {
useRef,
useImperativeHandle,
forwardRef
} from 'react' ;
const ModifiedInputComponent = forwardRef((props, ref) => {
const inputRef = useRef( null );
useImperativeHandle(ref, () => ({
focusInput: () => {
inputRef.current.focus();
}
}));
return (
<input
ref={inputRef}
type= "text"
placeholder={props.placeholder}
/>
);
});
export default ModifiedInputComponent;
|
Creating a reference to the input element in the child component is achieved using useRef. Subsequently, by utilizing useImperativeHandle, we expose a method named focusInput to the parent component via the ref. This mechanism empowers the parent component to invoke focusInput on the child component’s ref, facilitating programmatic focusing of the input element.
Javascript
import React, { useRef } from 'react' ;
import ModifiedInputComponent
from './ModifiedInputComponent' ;
const ModifiedParentComponent = () => {
const inputRef = useRef( null );
const handleFocusButtonClick = () => {
if (inputRef.current) {
inputRef.current.focusInput();
}
};
return (
<div>
{ }
<ModifiedInputComponent ref={inputRef}
placeholder= "Enter your name" />
<button onClick={handleFocusButtonClick}>
Focus Input
</button>
</div>
);
};
export default ModifiedParentComponent;
|
Utilizing useRef in the parent component establishes a reference (inputRef) aimed at interacting with the focusInput method of the child component. Upon clicking the “Focus Input” button, the handleFocusButtonClick function executes. Within this function, the focusInput method is invoked on the child component’s ref, consequently focusing the input element.
This example showcases the collaborative use of useRef and useImperativeHandle to construct a more regulated interface for parent components to interact with child components.
Steps to Create a React App:
Step 1: Create a React application using the following command.
npm create-react-app my-app
Step 2: Naviage to the root directory of your folder using the following commad.
cd my-app
Example: Below is an example to show a scnerario where useRef is useful.
Javascript
import { useRef } from 'react' ;
export default function Counter() {
let ref = useRef(0);
function handleClick() {
ref.current = ref.current + 1;
alert( 'You clicked' +
ref.current + 'times' );
}
return (
<button onClick={handleClick}>
Click me!
</button>
);
}
|
Start your application using the following command.
npm start
Ouput:
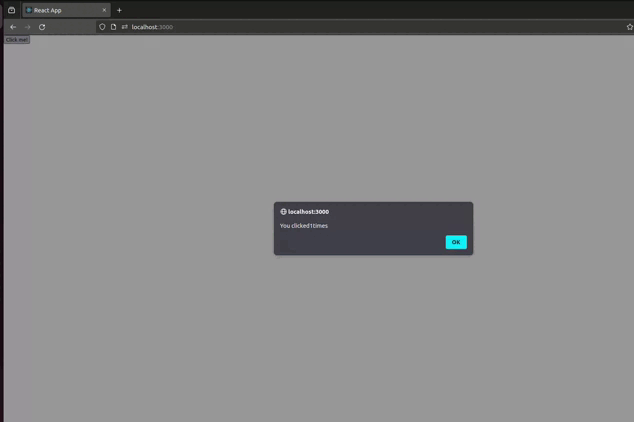
Output
Conclusion:
Referring to DOM elements directly within React components is a common need, but traditional methods like document.getElementById() can be inefficient in a React context. useRef offers a more efficient way to reference DOM elements, manage mutable values, and interact with child components imperatively. Its ability to persist values across renders without triggering re-renders is particularly useful for scenarios like managing DOM focus efficiently. Thus, useRef serves as a valuable tool for optimizing performance and state management in React applications.
Share your thoughts in the comments
Please Login to comment...