Create a Context Provider for Theming using React Hooks
Last Updated :
14 Mar, 2024
In this article, we are going to build a context provider for managing the theme of a React app using React hooks. This will allow the user to switch between dark and light themes throughout the application. Centralizing the theme will give us an edge as the application will be able to efficiently propagate theme changes to all components since we will wrap our entire application with the theme context provider created.
Output Preview: Let us have a look at how the final output will look like.
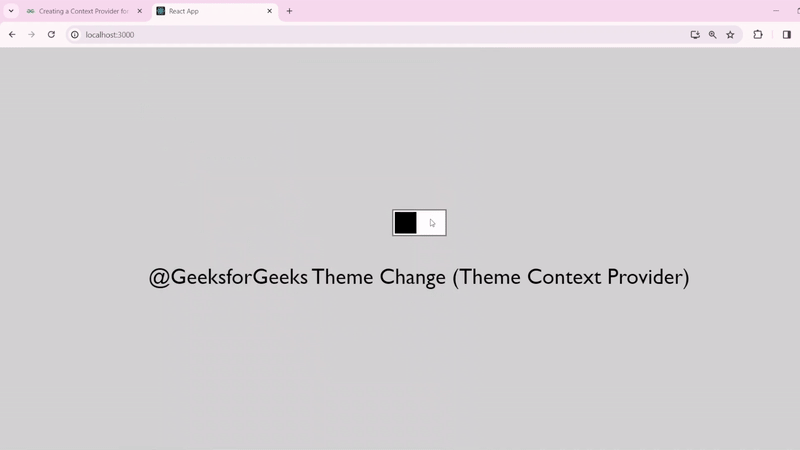
Preview Image of the Final Output
Prerequisites
Approach to Create Context Provider for Theming using React:
ThemeContext.js
defines a context for managing the theme state and provides a ThemeProvider
component to wrap the application.ThemeProvider
component utilizes the useState
hook to manage the theme state and exposes a toggleTheme
function to toggle between light and dark themes.useTheme
hook is provided to consume the theme context within components.index.js
wraps the App
component with the ThemeProvider
to make the theme context available throughout the application.App.js
utilizes the useTheme
hook to access the theme state and toggleTheme
function.- Theme-dependent styles are applied dynamically based on the current theme using inline styles. Toggling the theme updates the background color and button appearance accordingly.
- The
toggleTheme
function is invoked when the theme toggle button is clicked, updating the theme state between light and dark themes
Steps to Create the React App:
Step 1: Create a new react app and enter into it by running the following commands
npx create-react-app theme-context
cd theme-context
Step 2: Create `ThemeContext.js` at `src` where we will build our theme provider using createContext API.
Step 3: We will build a simple div in App.js, the styling of which will be conditionally changed as the state of theme changes. Obviously, we will create a toggle button to change the state of the theme.
Folder Structure:

project structure
Example Code: Provide below are the codes for the working example of a theme provider built using useContext API and useState hook.
CSS
/* App.css */
* {
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
transition: 0.5s ease-in-out;
margin: 0;
padding: 0;
box-sizing: border-box;
}
Javascript
// src/ThemeContext.js
import React, {
createContext,
useContext,
useState
} from 'react';
const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export const useTheme = () => useContext(ThemeContext);
Javascript
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { ThemeProvider } from './ThemeContext';
ReactDOM.render(
<React.StrictMode>
<ThemeProvider>
<App />
</ThemeProvider>
</React.StrictMode>,
document.getElementById('root')
);
Javascript
// src/App.js
import React from 'react';
import { useTheme } from './ThemeContext.js';
import './App.css';
const App = () => {
const { theme, toggleTheme } = useTheme();
return (
<>
<div style={{
display: 'flex', justifyContent: 'center',
alignItems: 'center', width: '100%',
height: '100vh',
background: theme === 'light' ? 'lightgray' : 'black' }}>
<div style={{
display: 'flex', flexDirection: 'column',
alignItems: 'center' }}>
<div>
<div onClick={() => toggleTheme()}
style={{
border: '1px solid gray', position: 'realtive',
width: '40px', height: '20px',
background: theme === 'light' ? 'white' : 'black' }}>
<div style={{ position: 'relative', top: '1px',
left: theme === 'light' ? '1px' : '21px',
width: '16px', height: '16px',
background: theme === 'light' ? 'black' : 'white' }}>
</div>
</div>
</div>
<div style={{
marginTop: '20px',
color: theme === 'light' ? 'black' : 'white' }}>
@GeeksforGeeks Theme Change (Theme Context Provider)
</div>
</div>
</div>
</>
);
};
export default App;
Start your application using the following command.
npm start
Output:
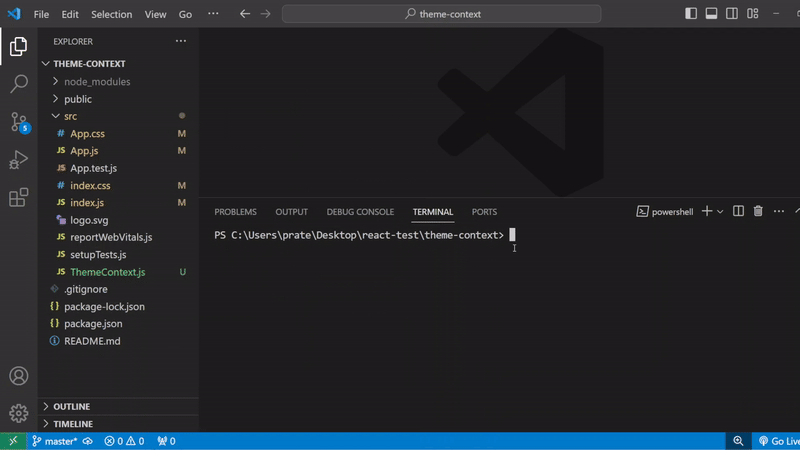
Context Provider for Theming using React Hooks
Share your thoughts in the comments
Please Login to comment...